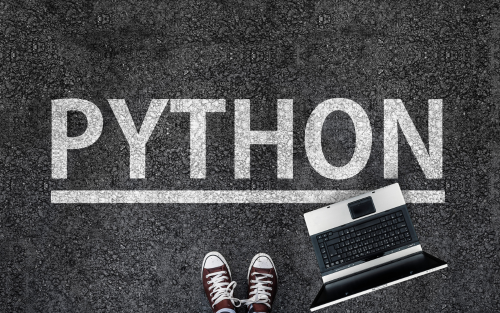
15 Apr How Do I Work With JSON Data In Python?
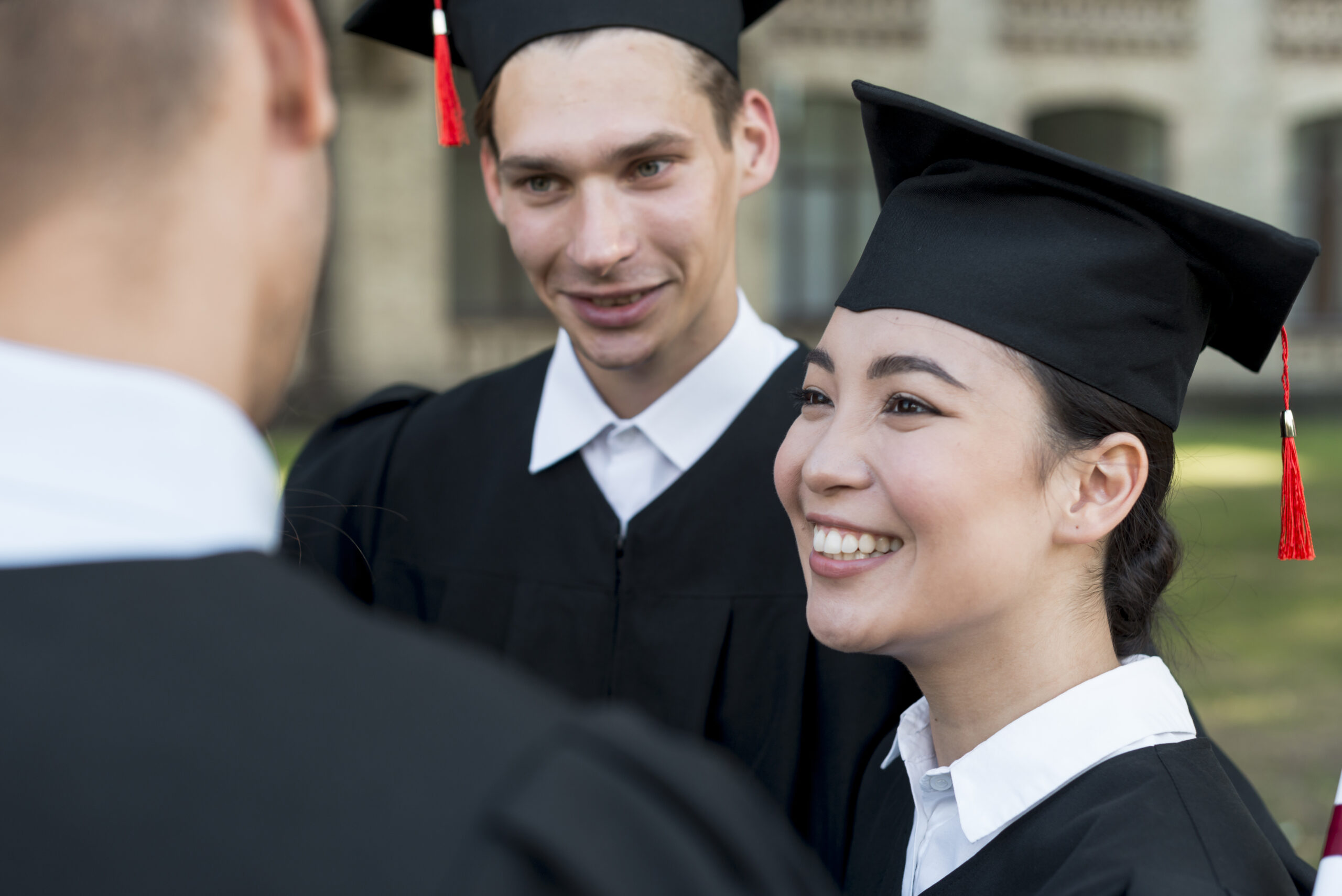
JSON (JavaScript Object Notation) is a popular format for data exchange between different systems. It is a lightweight and human-readable data interchange format that is easy to parse and generate. Python provides built-in support for working with JSON data through the json
module. In this article, we will explore how to work with JSON data in Python.
What is JSON?
JSON is a text format that is used to represent data in a structured way. It consists of key-value pairs, arrays, and nested objects. JSON is widely used for data exchange between web applications, as it is supported by all modern programming languages and web browsers.
JSON is based on two data structures: a collection of key-value pairs, and an ordered list of values. These two structures are represented in JSON as objects and arrays, respectively. An object is a collection of key-value pairs enclosed in curly braces {}
, while an array is an ordered list of values enclosed in square brackets []
.
Here is an example of a JSON object:
{
"name": "John Smith",
"age": 35,
"email": "john.smith@example.com"
}
In this example, we have a JSON object with three key-value pairs. The keys are name
, age
, and email
, and the corresponding values are "John Smith"
, 35
, and "john.smith@example.com"
, respectively.
JSON can also represent nested objects and arrays. Here is an example of a JSON object with a nested array:
{
"name": "John Smith",
"age": 35,
"email": "john.smith@example.com",
"hobbies": ["reading", "traveling", "playing guitar"]
}
In this example, we have a JSON object with four key-value pairs. The hobbies
key has an associated array value that contains three strings.
Working with JSON in Python
Python provides built-in support for working with JSON data through the json
module. The json
module provides methods for encoding and decoding JSON data, as well as manipulating JSON objects and arrays.
Encoding JSON data
To encode a Python object as JSON data, you can use the json.dumps()
method. This method takes a Python object as input and returns a JSON-formatted string.
Here is an example:
import json
data = {
"name": "John Smith",
"age": 35,
"email": "john.smith@example.com",
"hobbies": ["reading", "traveling", "playing guitar"]
}
json_data = json.dumps(data)
print(json_data)
In this example, we create a Python dictionary object data
that contains the same key-value pairs as the previous JSON example. We then use the json.dumps()
method to encode this object as a JSON-formatted string. Finally, we print the JSON-formatted string.
Decoding JSON data
To decode a JSON-formatted string into a Python object, you can use the json.loads()
method. This method takes a JSON-formatted string as input and returns a Python object.
Here is an example:
import json
json_data = '{"name": "John Smith", "age": 35, "email": "john.smith@example.com", "hobbies": ["reading", "traveling", "playing guitar"]}'
data = json.loads(json_data)
print(data)
In this example, we create a JSON-formatted string json_data
that contains the same data as the previous example. We then use the json.loads()
method to decode this string into a Python object. Finally, we print the Python object.
Working with JSON files
Python also provides methods for working with JSON files. To read JSON data from a file, you can use the json.load()
method. This method takes a file object as input and returns a Python object.
Here is an example:
import json
with open('data.json', 'r') as f:
data = json.load(f)
print(data)
In this example, we use the open()
function to open a file named data.json
in read mode. We then use the json.load()
method to read the JSON data from the file and store it in a Python object data
. Finally, we print the Python object.
To write JSON data to a file, you can use the json.dump()
method. This method takes a Python object and a file object as input and writes the JSON data to the file.
Here is an example:
import json
data = {
"name": "John Smith",
"age": 35,
"email": "john.smith@example.com",
"hobbies": ["reading", "traveling", "playing guitar"]
}
with open('data.json', 'w') as f:
json.dump(data, f)
In this example, we create a Python object data
that contains the same key-value pairs as the previous examples. We then use the open()
function to open a file named data.json
in write mode. We use the json.dump()
method to write the JSON data from the Python object data
to the file. Finally, we close the file.
Manipulating JSON data
Python provides methods for manipulating JSON data, such as adding or removing key-value pairs, or modifying the values of existing pairs.
Here is an example of how to add a key-value pair to a JSON object:
import json
json_data = '{"name": "John Smith", "age": 35, "email": "john.smith@example.com", "hobbies": ["reading", "traveling", "playing guitar"]}'
data = json.loads(json_data)
data['address'] = '123 Main St'
json_data = json.dumps(data)
print(json_data)
In this example, we create a JSON-formatted string json_data
that contains the same data as the previous examples. We then use the json.loads()
method to decode this string into a Python object data
. We add a new key-value pair to the data
object using square bracket notation. We then use the json.dumps()
method to encode the modified data
object as a JSON-formatted string. Finally, we print the JSON-formatted string.
Conclusion
In this article, we have explored how to work with JSON data in Python. We have learned how to encode and decode JSON data using the json
module, as well as how to read and write JSON data to files. We have also seen how to manipulate JSON data using Python’s built-in methods. By using these techniques, you can easily work with JSON data in your Python projects.
Latest Topic
-
Cloud-Native Technologies: Best Practices
20 April, 2024 -
Generative AI with Llama 3: Shaping the Future
15 April, 2024 -
Mastering Llama 3: The Ultimate Guide
10 April, 2024
Category
- Assignment Help
- Homework Help
- Programming
- Trending Topics
- C Programming Assignment Help
- Art, Interactive, And Robotics
- Networked Operating Systems Programming
- Knowledge Representation & Reasoning Assignment Help
- Digital Systems Assignment Help
- Computer Design Assignment Help
- Artificial Life And Digital Evolution
- Coding and Fundamentals: Working With Collections
- UML Online Assignment Help
- Prolog Online Assignment Help
- Natural Language Processing Assignment Help
- Julia Assignment Help
- Golang Assignment Help
- Design Implementation Of Network Protocols
- Computer Architecture Assignment Help
- Object-Oriented Languages And Environments
- Coding Early Object and Algorithms: Java Coding Fundamentals
- Deep Learning In Healthcare Assignment Help
- Geometric Deep Learning Assignment Help
- Models Of Computation Assignment Help
- Systems Performance And Concurrent Computing
- Advanced Security Assignment Help
- Typescript Assignment Help
- Computational Media Assignment Help
- Design And Analysis Of Algorithms
- Geometric Modelling Assignment Help
- JavaScript Assignment Help
- MySQL Online Assignment Help
- Programming Practicum Assignment Help
- Public Policy, Legal, And Ethical Issues In Computing, Privacy, And Security
- Computer Vision
- Advanced Complexity Theory Assignment Help
- Big Data Mining Assignment Help
- Parallel Computing And Distributed Computing
- Law And Computer Science Assignment Help
- Engineering Distributed Objects For Cloud Computing
- Building Secure Computer Systems Assignment Help
- Ada Assignment Help
- R Programming Assignment Help
- Oracle Online Assignment Help
- Languages And Automata Assignment Help
- Haskell Assignment Help
- Economics And Computation Assignment Help
- ActionScript Assignment Help
- Audio Programming Assignment Help
- Bash Assignment Help
- Computer Graphics Assignment Help
- Groovy Assignment Help
- Kotlin Assignment Help
- Object Oriented Languages And Environments
- COBOL ASSIGNMENT HELP
- Bayesian Statistical Probabilistic Programming
- Computer Network Assignment Help
- Django Assignment Help
- Lambda Calculus Assignment Help
- Operating System Assignment Help
- Computational Learning Theory
- Delphi Assignment Help
- Concurrent Algorithms And Data Structures Assignment Help
- Machine Learning Assignment Help
- Human Computer Interface Assignment Help
- Foundations Of Data Networking Assignment Help
- Continuous Mathematics Assignment Help
- Compiler Assignment Help
- Computational Biology Assignment Help
- PostgreSQL Online Assignment Help
- Lua Assignment Help
- Human Computer Interaction Assignment Help
- Ethics And Responsible Innovation Assignment Help
- Communication And Ethical Issues In Computing
- Computer Science
- Combinatorial Optimisation Assignment Help
- Ethical Computing In Practice
- HTML Homework Assignment Help
- Linear Algebra Assignment Help
- Perl Assignment Help
- Artificial Intelligence Assignment Help
- Uncategorized
- Ethics And Professionalism Assignment Help
- Human Augmentics Assignment Help
- Linux Assignment Help
- PHP Assignment Help
- Assembly Language Assignment Help
- Dart Assignment Help
- Complete Python Bootcamp From Zero To Hero In Python Corrected Version
- Swift Assignment Help
- Computational Complexity Assignment Help
- Probability And Computing Assignment Help
- MATLAB Programming For Engineers
- Introduction To Statistical Learning
- Database Systems Implementation Assignment Help
- Computational Game Theory Assignment Help
- Database Assignment Help
- Probabilistic Model Checking Assignment Help
- Mathematics For Computer Science And Philosophy
- Introduction To Formal Proof Assignment Help
- Creative Coding Assignment Help
- Foundations Of Self-Programming Agents Assignment Help
- Machine Organization Assignment Help
- Software Design Assignment Help
- Data Communication And Networking Assignment Help
- Computational Biology
- Data Structure Assignment Help
- Foundations Of Software Engineering Assignment Help
- Mathematical Foundations Of Computing
- Principles Of Programming Languages Assignment Help
- Software Engineering Capstone Assignment Help
- Algorithms and Data Structures Assignment Help
No Comments