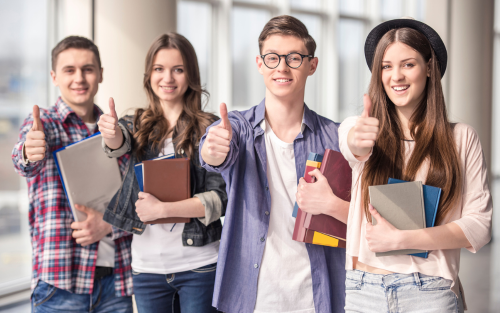
25 Apr Building A Simple Chatbot Using Python
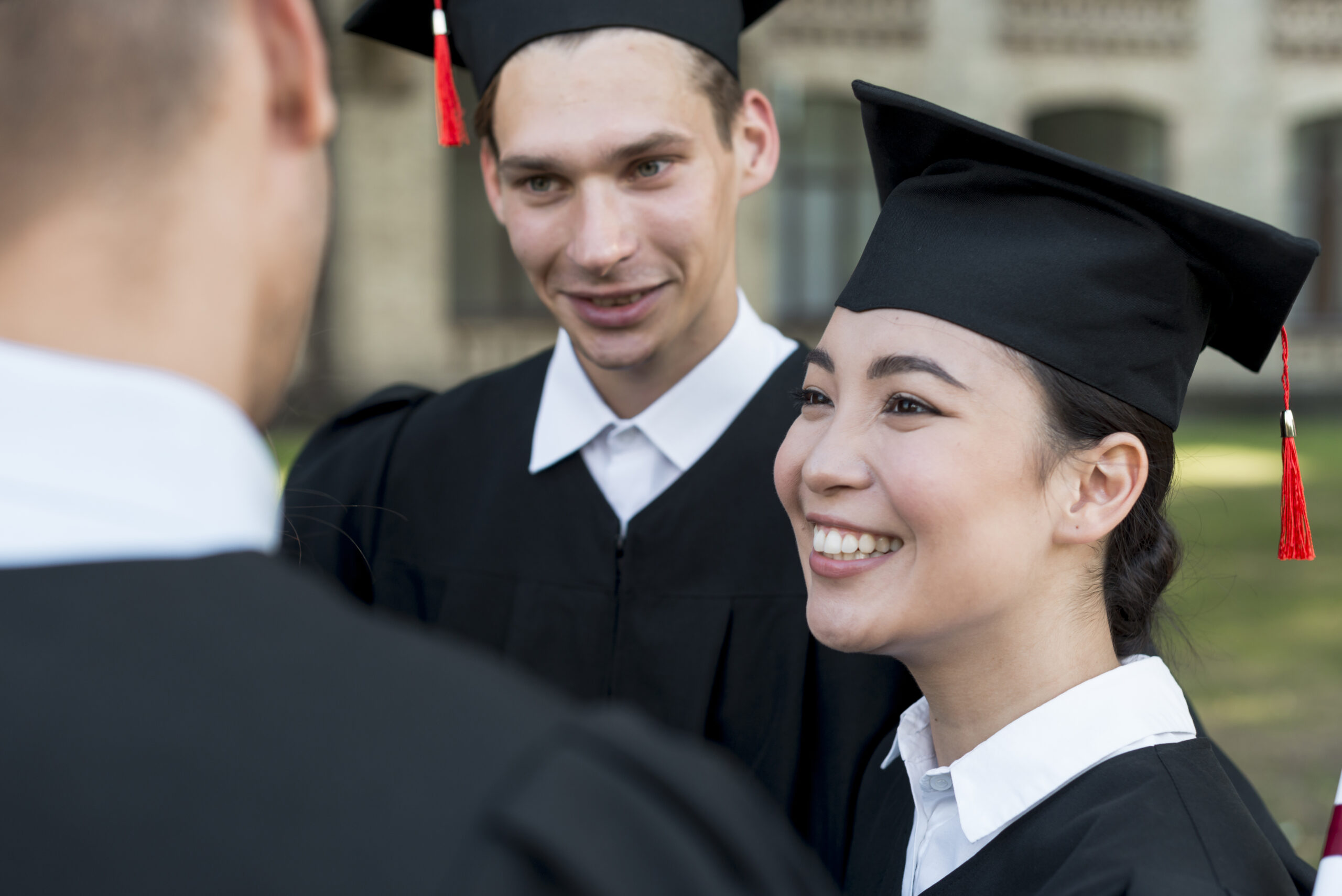
Chatbots have become increasingly popular in recent years, as they provide a way to automate customer service and support, and provide users with quick and easy access to information. In this tutorial, we’ll walk you through the process of building a simple chatbot using Python.
The chatbot we’ll build will use natural language processing (NLP) to understand user inputs and generate appropriate responses. We’ll use the Python programming language and the Natural Language Toolkit (NLTK) library to implement the chatbot.
Prerequisites
Before we get started building our chatbot, there are a few prerequisites we’ll need to have in place:
Python 3.x installed on your computer
The NLTK library installed in Python
Basic knowledge of Python programming language and natural language processing (NLP)
Step 1: Install NLTK Library
The first step in building our chatbot is to install the NLTK library in Python. NLTK is a popular Python library used for natural language processing tasks such as tokenization, stemming, tagging, parsing, and semantic reasoning.
To install NLTK, open your terminal or command prompt and run the following command:
pip install nltk
Step 2: Import NLTK Library and Download Corpora
Once NLTK is installed, we’ll need to import it into our Python script. We’ll also need to download some corpora, which are large bodies of text used for training and testing NLP models.
Open a Python script in your preferred code editor and add the following lines of code:
import nltk
nltk.download('punkt')
nltk.download('wordnet')
The first line imports the NLTK library, and the next two lines download the ‘punkt’ and ‘wordnet’ corpora. These corpora are used for tokenization and stemming, respectively.
Step 3: Define Chatbot Functions
Next, we’ll define some functions that our chatbot will use to process user inputs and generate responses. These functions will use NLTK to perform tasks such as tokenization, stemming, and semantic reasoning.
from nltk.stem import WordNetLemmatizer
import numpy as np
import random
import string
#Importing the necessary libraries
f=open('chatbot.txt','r',errors = 'ignore')
raw=f.read()
raw=raw.lower()
sent_tokens = nltk.sent_tokenize(raw)
word_tokens = nltk.word_tokenize(raw)
lemmer = WordNetLemmatizer()
def LemTokens(tokens):
return [lemmer.lemmatize(token) for token in tokens]
remove_punct_dict = dict((ord(punct), None) for punct in string.punctuation)
def LemNormalize(text):
return LemTokens(nltk.word_tokenize(text.lower().translate(remove_punct_dict)))
The WordNetLemmatizer
function is used for stemming, which is the process of reducing words to their base form. The sent_tokenize
and word_tokenize
functions are used for tokenization, which is the process of breaking text into individual words and sentences.
The LemTokens
function applies the lemmatization process to the tokens generated by the word_tokenize
function. The remove_punct_dict
variable is used to remove any punctuation from the user input.
The LemNormalize
function applies the LemTokens
and remove_punct_dict
functions to the user input.
Step 4: Define Chatbot Responses
Next, we’ll define some responses that our chatbot will generate based on user inputs. We’ll create a dictionary that maps user inputs to appropriate responses.
GREETING_INPUTS = ["hello", "hi", "greetings", "sup", "what
In addition to the above, another key aspect of building a chatbot is the use of natural language processing (NLP) techniques. NLP involves the use of algorithms to enable computers to understand, interpret, and generate human language. NLP techniques such as sentiment analysis, entity recognition, and intent classification can help the chatbot understand and respond appropriately to user inputs.
To implement NLP in Python, you can use libraries such as NLTK (Natural Language Toolkit) and spaCy. These libraries provide pre-trained models and tools for various NLP tasks, which can be integrated into your chatbot.
Once you have incorporated NLP techniques into your chatbot, you can also consider implementing machine learning algorithms to improve the chatbot’s performance. For example, you can use machine learning algorithms to train your chatbot to recognize user intent more accurately and respond accordingly.
To train a machine learning model for your chatbot, you will need a dataset of labeled user inputs and corresponding chatbot responses. You can create this dataset by collecting real user inputs and responses, or by generating synthetic data using techniques such as data augmentation.
Once you have a dataset, you can use a machine learning framework such as TensorFlow or scikit-learn to train a model. The trained model can then be integrated into your chatbot to improve its performance.
Conclusion
In this blog post, we have covered the basics of building a chatbot using Python. We started by discussing the benefits of chatbots and the various types of chatbots available. We then covered the key components of a chatbot and how to implement them using Python. We also discussed the importance of incorporating NLP techniques and machine learning algorithms to improve the chatbot’s performance.
Building a chatbot can be a challenging task, but with the right tools and techniques, it can be a rewarding experience. By following the steps outlined in this blog post, you can build a simple chatbot using Python that can respond to user inputs and provide helpful information.
Latest Topic
-
Cloud-Native Technologies: Best Practices
20 April, 2024 -
Generative AI with Llama 3: Shaping the Future
15 April, 2024 -
Mastering Llama 3: The Ultimate Guide
10 April, 2024
Category
- Assignment Help
- Homework Help
- Programming
- Trending Topics
- C Programming Assignment Help
- Art, Interactive, And Robotics
- Networked Operating Systems Programming
- Knowledge Representation & Reasoning Assignment Help
- Digital Systems Assignment Help
- Computer Design Assignment Help
- Artificial Life And Digital Evolution
- Coding and Fundamentals: Working With Collections
- UML Online Assignment Help
- Prolog Online Assignment Help
- Natural Language Processing Assignment Help
- Julia Assignment Help
- Golang Assignment Help
- Design Implementation Of Network Protocols
- Computer Architecture Assignment Help
- Object-Oriented Languages And Environments
- Coding Early Object and Algorithms: Java Coding Fundamentals
- Deep Learning In Healthcare Assignment Help
- Geometric Deep Learning Assignment Help
- Models Of Computation Assignment Help
- Systems Performance And Concurrent Computing
- Advanced Security Assignment Help
- Typescript Assignment Help
- Computational Media Assignment Help
- Design And Analysis Of Algorithms
- Geometric Modelling Assignment Help
- JavaScript Assignment Help
- MySQL Online Assignment Help
- Programming Practicum Assignment Help
- Public Policy, Legal, And Ethical Issues In Computing, Privacy, And Security
- Computer Vision
- Advanced Complexity Theory Assignment Help
- Big Data Mining Assignment Help
- Parallel Computing And Distributed Computing
- Law And Computer Science Assignment Help
- Engineering Distributed Objects For Cloud Computing
- Building Secure Computer Systems Assignment Help
- Ada Assignment Help
- R Programming Assignment Help
- Oracle Online Assignment Help
- Languages And Automata Assignment Help
- Haskell Assignment Help
- Economics And Computation Assignment Help
- ActionScript Assignment Help
- Audio Programming Assignment Help
- Bash Assignment Help
- Computer Graphics Assignment Help
- Groovy Assignment Help
- Kotlin Assignment Help
- Object Oriented Languages And Environments
- COBOL ASSIGNMENT HELP
- Bayesian Statistical Probabilistic Programming
- Computer Network Assignment Help
- Django Assignment Help
- Lambda Calculus Assignment Help
- Operating System Assignment Help
- Computational Learning Theory
- Delphi Assignment Help
- Concurrent Algorithms And Data Structures Assignment Help
- Machine Learning Assignment Help
- Human Computer Interface Assignment Help
- Foundations Of Data Networking Assignment Help
- Continuous Mathematics Assignment Help
- Compiler Assignment Help
- Computational Biology Assignment Help
- PostgreSQL Online Assignment Help
- Lua Assignment Help
- Human Computer Interaction Assignment Help
- Ethics And Responsible Innovation Assignment Help
- Communication And Ethical Issues In Computing
- Computer Science
- Combinatorial Optimisation Assignment Help
- Ethical Computing In Practice
- HTML Homework Assignment Help
- Linear Algebra Assignment Help
- Perl Assignment Help
- Artificial Intelligence Assignment Help
- Uncategorized
- Ethics And Professionalism Assignment Help
- Human Augmentics Assignment Help
- Linux Assignment Help
- PHP Assignment Help
- Assembly Language Assignment Help
- Dart Assignment Help
- Complete Python Bootcamp From Zero To Hero In Python Corrected Version
- Swift Assignment Help
- Computational Complexity Assignment Help
- Probability And Computing Assignment Help
- MATLAB Programming For Engineers
- Introduction To Statistical Learning
- Database Systems Implementation Assignment Help
- Computational Game Theory Assignment Help
- Database Assignment Help
- Probabilistic Model Checking Assignment Help
- Mathematics For Computer Science And Philosophy
- Introduction To Formal Proof Assignment Help
- Creative Coding Assignment Help
- Foundations Of Self-Programming Agents Assignment Help
- Machine Organization Assignment Help
- Software Design Assignment Help
- Data Communication And Networking Assignment Help
- Computational Biology
- Data Structure Assignment Help
- Foundations Of Software Engineering Assignment Help
- Mathematical Foundations Of Computing
- Principles Of Programming Languages Assignment Help
- Software Engineering Capstone Assignment Help
- Algorithms and Data Structures Assignment Help
No Comments