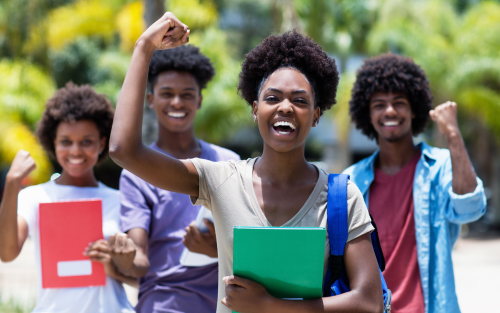
26 Apr Best Practices For Handling Exceptions In Python
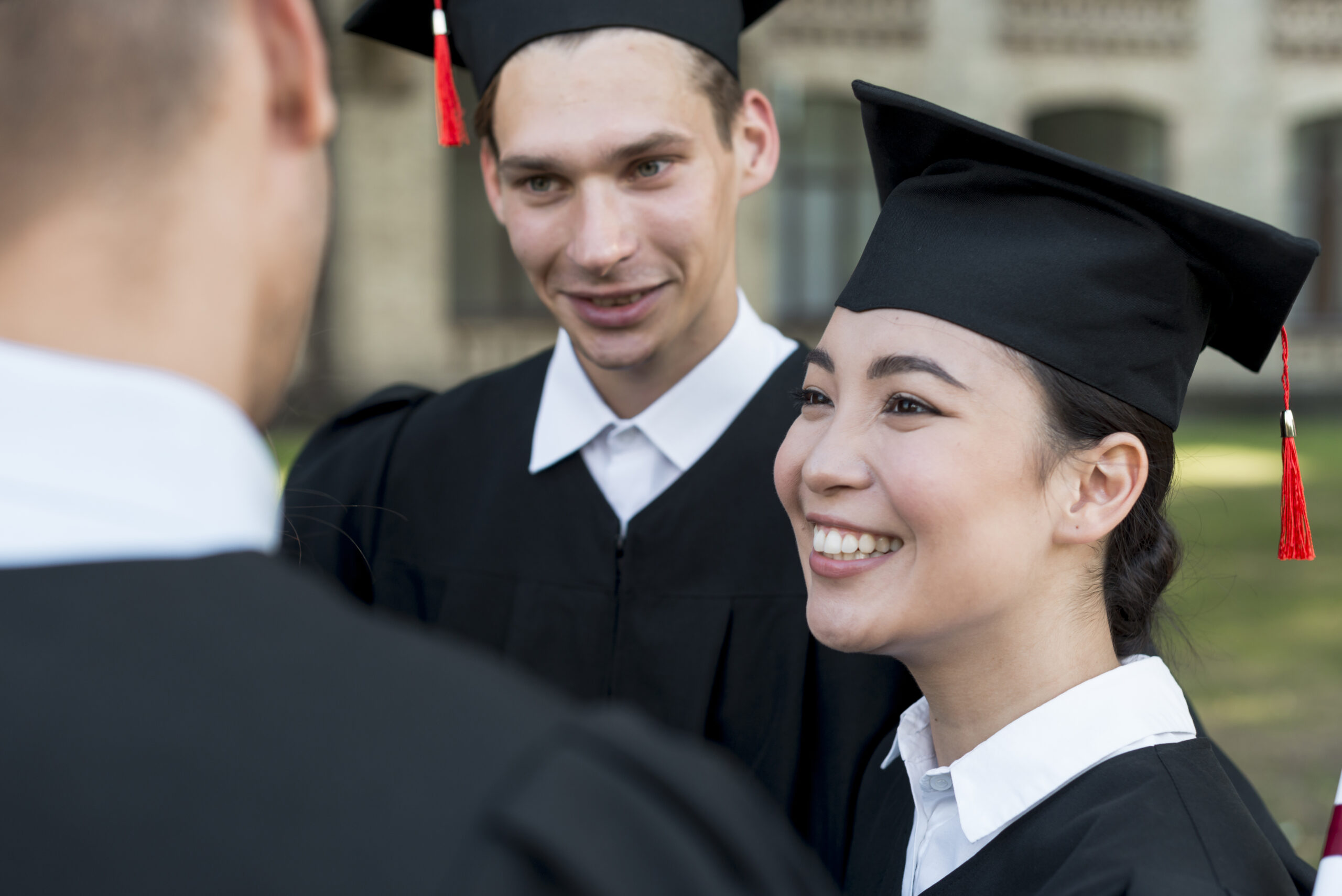
Errors and exceptions are common in programming, and they can occur for a variety of reasons, such as invalid input, system failures, or programming errors. As a developer, it’s essential to handle these exceptions gracefully to ensure that your application remains stable and continues to function as expected. In Python, exceptions are objects that are raised when an error occurs, and they can be handled using the try and except statements. In this blog post, we will explore best practices for handling exceptions in Python.
Be Specific When Catching Exceptions: When catching exceptions, it’s important to be as specific as possible. Instead of using a generic except statement to catch all exceptions, use specific except statements that handle only the expected exceptions. This helps to prevent unintended consequences and makes it easier to identify and fix errors. For example:
try:
# some code
except ValueError:
# handle ValueError exception
except KeyError:
# handle KeyError exception
except:
# handle all other exceptions
Log Exceptions: It’s important to log exceptions to help diagnose and troubleshoot errors. By logging exceptions, you can identify patterns, track error frequency, and determine where to focus your efforts. In Python, you can use the logging module to log exceptions. For example:
import logging
try:
# some code
except Exception as e:
logging.exception("Exception occurred")
Raising Exceptions: Sometimes it’s necessary to raise exceptions explicitly to indicate that something has gone wrong. You can raise exceptions using the raise statement, which takes an exception object as an argument. For example:
def divide(a, b):
if b == 0:
raise ValueError("Cannot divide by zero")
return a / b
Use Context Managers: Context managers provide a way to manage resources, such as files or network connections, by automatically closing them when they are no longer needed. They are a powerful tool for handling exceptions because they ensure that resources are properly closed, even if an exception occurs. In Python, you can use the with statement to create a context manager. For example:
with open("file.txt", "w") as f:
f.write("Hello, world!")
Use Finally Blocks: Finally blocks are used to clean up resources, regardless of whether an exception occurred or not. They are executed after the try block and any except blocks, even if an exception was raised. Finally blocks are useful for releasing resources or closing files that were opened in the try block. For example:
try:
# some code
except:
# handle exception
finally:
# cleanup resources
Don’t Use Bare Except Clauses: Bare except clauses catch all exceptions, including system exceptions and user-defined exceptions. This can make it difficult to diagnose and troubleshoot errors because it’s unclear what caused the exception. Instead, use specific except clauses to handle expected exceptions, and use a top-level except clause to catch any unexpected exceptions. For example:
try:
# some code
except ValueError:
# handle ValueError exception
except Exception as e:
# handle all other exceptions
logging.exception("Unexpected exception occurred")
Use Multiple Except Clauses: In some cases, you may need to handle multiple exceptions with the same code. Instead of duplicating the code for each exception, you can use multiple except clauses to handle them together. For example:
try:
# some code
except (ValueError, KeyError):
# handle ValueError or KeyError exception
Conclusion
Latest Topic
-
Cloud-Native Technologies: Best Practices
20 April, 2024 -
Generative AI with Llama 3: Shaping the Future
15 April, 2024 -
Mastering Llama 3: The Ultimate Guide
10 April, 2024
Category
- Assignment Help
- Homework Help
- Programming
- Trending Topics
- C Programming Assignment Help
- Art, Interactive, And Robotics
- Networked Operating Systems Programming
- Knowledge Representation & Reasoning Assignment Help
- Digital Systems Assignment Help
- Computer Design Assignment Help
- Artificial Life And Digital Evolution
- Coding and Fundamentals: Working With Collections
- UML Online Assignment Help
- Prolog Online Assignment Help
- Natural Language Processing Assignment Help
- Julia Assignment Help
- Golang Assignment Help
- Design Implementation Of Network Protocols
- Computer Architecture Assignment Help
- Object-Oriented Languages And Environments
- Coding Early Object and Algorithms: Java Coding Fundamentals
- Deep Learning In Healthcare Assignment Help
- Geometric Deep Learning Assignment Help
- Models Of Computation Assignment Help
- Systems Performance And Concurrent Computing
- Advanced Security Assignment Help
- Typescript Assignment Help
- Computational Media Assignment Help
- Design And Analysis Of Algorithms
- Geometric Modelling Assignment Help
- JavaScript Assignment Help
- MySQL Online Assignment Help
- Programming Practicum Assignment Help
- Public Policy, Legal, And Ethical Issues In Computing, Privacy, And Security
- Computer Vision
- Advanced Complexity Theory Assignment Help
- Big Data Mining Assignment Help
- Parallel Computing And Distributed Computing
- Law And Computer Science Assignment Help
- Engineering Distributed Objects For Cloud Computing
- Building Secure Computer Systems Assignment Help
- Ada Assignment Help
- R Programming Assignment Help
- Oracle Online Assignment Help
- Languages And Automata Assignment Help
- Haskell Assignment Help
- Economics And Computation Assignment Help
- ActionScript Assignment Help
- Audio Programming Assignment Help
- Bash Assignment Help
- Computer Graphics Assignment Help
- Groovy Assignment Help
- Kotlin Assignment Help
- Object Oriented Languages And Environments
- COBOL ASSIGNMENT HELP
- Bayesian Statistical Probabilistic Programming
- Computer Network Assignment Help
- Django Assignment Help
- Lambda Calculus Assignment Help
- Operating System Assignment Help
- Computational Learning Theory
- Delphi Assignment Help
- Concurrent Algorithms And Data Structures Assignment Help
- Machine Learning Assignment Help
- Human Computer Interface Assignment Help
- Foundations Of Data Networking Assignment Help
- Continuous Mathematics Assignment Help
- Compiler Assignment Help
- Computational Biology Assignment Help
- PostgreSQL Online Assignment Help
- Lua Assignment Help
- Human Computer Interaction Assignment Help
- Ethics And Responsible Innovation Assignment Help
- Communication And Ethical Issues In Computing
- Computer Science
- Combinatorial Optimisation Assignment Help
- Ethical Computing In Practice
- HTML Homework Assignment Help
- Linear Algebra Assignment Help
- Perl Assignment Help
- Artificial Intelligence Assignment Help
- Uncategorized
- Ethics And Professionalism Assignment Help
- Human Augmentics Assignment Help
- Linux Assignment Help
- PHP Assignment Help
- Assembly Language Assignment Help
- Dart Assignment Help
- Complete Python Bootcamp From Zero To Hero In Python Corrected Version
- Swift Assignment Help
- Computational Complexity Assignment Help
- Probability And Computing Assignment Help
- MATLAB Programming For Engineers
- Introduction To Statistical Learning
- Database Systems Implementation Assignment Help
- Computational Game Theory Assignment Help
- Database Assignment Help
- Probabilistic Model Checking Assignment Help
- Mathematics For Computer Science And Philosophy
- Introduction To Formal Proof Assignment Help
- Creative Coding Assignment Help
- Foundations Of Self-Programming Agents Assignment Help
- Machine Organization Assignment Help
- Software Design Assignment Help
- Data Communication And Networking Assignment Help
- Computational Biology
- Data Structure Assignment Help
- Foundations Of Software Engineering Assignment Help
- Mathematical Foundations Of Computing
- Principles Of Programming Languages Assignment Help
- Software Engineering Capstone Assignment Help
- Algorithms and Data Structures Assignment Help
No Comments