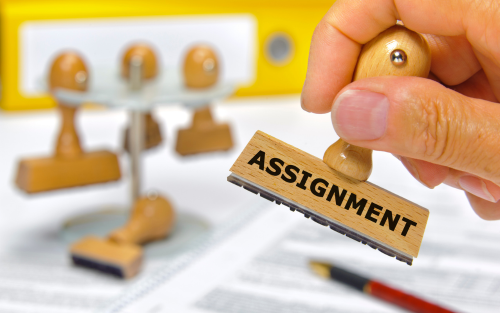
01 May Tips For Writing More Scalable Javascript Code
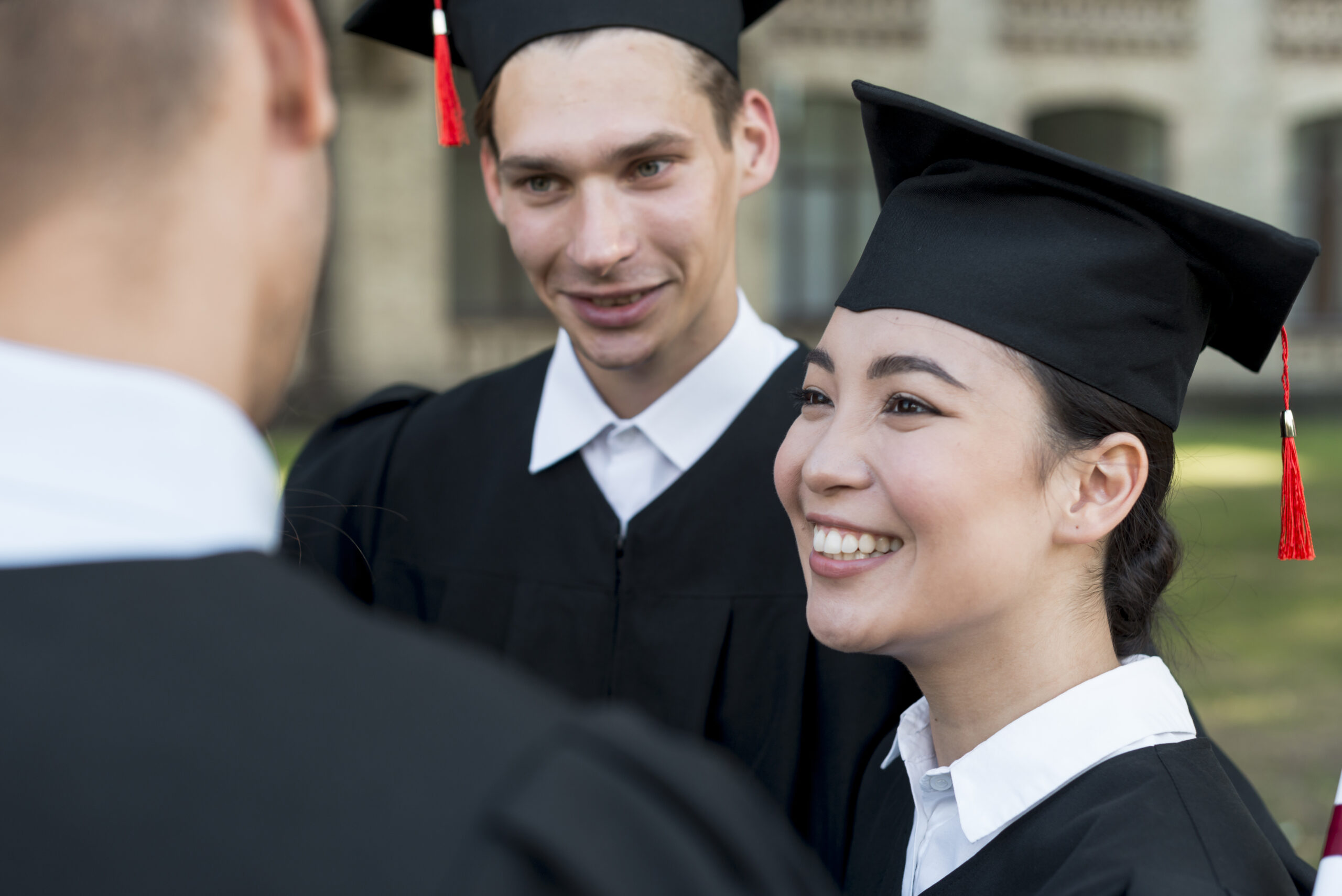
As web applications grow larger and more complex, it becomes essential to write scalable JavaScript code. Writing scalable code means that the code can handle increased traffic, user requests, and data processing without crashing or slowing down the application. In this article, we will discuss some tips for writing more scalable JavaScript code.
Tip 1: Use a module bundler
When writing JavaScript code for larger applications, it’s essential to use a module bundler. A module bundler is a tool that combines separate JavaScript files into a single file, which can be loaded by the browser. This approach not only speeds up the application’s loading time but also helps to avoid naming collisions and namespace issues.
Some popular module bundlers for JavaScript include Webpack, Rollup, and Browserify.
Tip 2: Use a JavaScript framework
Using a JavaScript framework can make writing scalable code much more manageable. A framework provides a structure for the application and often includes features such as data binding, templating, and routing. This reduces the amount of custom code that needs to be written, making it easier to maintain and update the application.
Some popular JavaScript frameworks include React, Angular, and Vue.
Tip 3: Avoid global variables
Global variables can cause issues when writing scalable JavaScript code. If multiple scripts rely on the same global variable, it can be challenging to manage and update the application. Instead, it’s better to use module-level variables or pass variables through functions.
Tip 4: Optimize loops and conditionals
When processing large amounts of data, it’s essential to optimize loops and conditionals. This can be achieved by using methods such as map, filter, and reduce instead of for loops. These methods can handle large data sets more efficiently and are easier to read and maintain.
Tip 5: Use caching and memoization
Caching and memoization can significantly improve the performance of JavaScript code. Caching involves storing the result of a function call, so it can be reused if the function is called again with the same input. Memoization is a similar technique that involves caching the result of a function call for a particular set of inputs.
Tip 6: Minify and compress JavaScript files
Minifying and compressing JavaScript files can reduce the file size, which can significantly improve the application’s performance. Minification involves removing unnecessary characters, such as comments and white space, from the code. Compression involves reducing the size of the code by encoding it using algorithms such as Gzip or Brotli.
Tip 7: Use asynchronous code
Asynchronous code can help to avoid blocking the application’s main thread, making it more scalable. Asynchronous code involves using functions such as setTimeout, setInterval, and Promises to execute code in the background while other code continues to run.
Tip 8: Use testing and debugging tools
Using testing and debugging tools can help to identify and fix issues in the code before they become major problems. Testing tools such as Jest and Mocha can be used to test the code’s functionality and performance, while debugging tools such as Chrome DevTools can be used to debug the code and identify performance bottlenecks.
Tip 9: Use a linter
Using a linter can help to identify and fix common issues in the code, such as syntax errors and unused variables. Linting tools such as ESLint can be used to enforce coding standards and ensure that the code is consistent and easy to read.
Tip 10: Keep code modular and maintainable
Keeping code modular and maintainable can make it easier to update and scale the application. This can be achieved by breaking the code down into smaller, reusable components and using descriptive variable and function names.
FAQs
What does it mean for JavaScript code to be scalable?
Scalable JavaScript code refers to code that can handle increased workloads and larger datasets without sacrificing performance or introducing significant complexity. It should be able to accommodate growth in user base, data volume, and feature requirements while maintaining a high level of maintainability.
What are the benefits of writing scalable JavaScript code?
Writing scalable JavaScript code offers benefits such as improved performance, easier code maintenance and debugging, better code reusability, and the ability to accommodate future growth and changes in requirements without significant refactoring.
How can you structure JavaScript code for scalability?
To structure JavaScript code for scalability, consider organizing code into modules or components based on functionality, ensuring separation of concerns, adopting modular design patterns like the module pattern or revealing module pattern, and leveraging frameworks or libraries that promote scalable architectures, such as MVC (Model-View-Controller) or MVVM (Model-View-ViewModel).
What are some best practices for writing scalable JavaScript code?
- Follow coding conventions and style guides to ensure consistency and readability.
- Break down complex code into smaller functions or modules to promote reusability.
- Avoid global variables and use proper scoping techniques.
- Optimize performance by minimizing DOM manipulation, reducing unnecessary network requests, and optimizing algorithms.
- Implement error handling and use appropriate exception handling techniques.
- Use asynchronous programming techniques, such as Promises or async/await, to handle long-running operations without blocking the main thread.
- Implement caching mechanisms for frequently accessed data or resources.
- Apply code optimization techniques, such as minification and bundling, to reduce file size and improve loading speed.
- Write test cases and perform unit testing to ensure code quality and catch regressions early.
How can you ensure scalability in JavaScript frameworks like React or Angular?
- Follow component-based architecture and break down complex UIs into smaller reusable components.
- Optimize rendering performance by implementing shouldComponentUpdate or memoization techniques to prevent unnecessary re-renders.
- Leverage lazy loading and code splitting to improve initial loading speed.
- Implement state management solutions like Redux or Vuex to centralize and manage application state.
- Utilize server-side rendering or static site generation to improve initial page load performance.
- Design scalable APIs and use data fetching techniques like pagination or infinite scrolling to efficiently handle large datasets.
How can you handle scalability in JavaScript applications that interact with backend services or APIs?
- Implement client-side caching mechanisms to reduce the number of requests to the backend.
- Optimize API calls by batching or aggregating requests, implementing caching on the server-side, or utilizing techniques like GraphQL to fetch precisely the required data.
- Consider using load balancing techniques or employing a scalable backend infrastructure to handle increased traffic and ensure high availability.
How can you monitor and optimize the performance of a scalable JavaScript application?
- Utilize browser developer tools to analyze network requests, inspect rendering performance, and identify performance bottlenecks.
- Implement logging and monitoring solutions to track application performance, identify errors, and gather insights for optimization.
- Use performance profiling tools to identify areas of code that are causing performance degradation.
- Perform load testing and stress testing to evaluate the application’s performance under heavy loads and identify potential bottlenecks.
What are some recommended techniques for handling scalability in real-time or WebSocket-based JavaScript applications?
- Employ techniques like horizontal scaling and load balancing to handle increased WebSocket connections.
- Use message queuing systems or publish-subscribe patterns to distribute messages efficiently among different instances or processes.
- Implement state management techniques, such as CRDTs (Conflict-free Replicated Data Types), for managing shared state across multiple instances.
How can you ensure backward compatibility and scalability in JavaScript code?
- Follow versioning best practices and maintain a clear versioning strategy to handle backward compatibility.
- Implement feature toggles or flags to control the availability of new features and gradually roll them out.
- Write robust and extensive automated tests to catch regressions when introducing new changes or updates.
How can you optimize the performance of JavaScript code in a mobile or low-bandwidth environment?
- Minimize the use of large libraries or frameworks to reduce file sizes.
- Optimize images and other assets for mobile devices.
- Implement lazy loading techniques to defer the loading of non-critical resources.
- Use compression techniques like GZIP to reduce the size of network responses.
- Optimize network requests by reducing the number of requests and implementing efficient caching strategies.
Conclusion
In this article, we have discussed ten tips for writing more scalable JavaScript code. These tips include using a module bundler, using a JavaScript framework, avoiding global variables, optimizing loops and conditionals, using caching and memoization, minifying and compressing JavaScript files, using asynchronous code, using testing and debugging tools, using a linter, and keeping code modular and maintainable.
By following these tips, developers can write code that is scalable, efficient, and easy to maintain. Writing scalable code is essential for creating successful and high-performing web applications. With these tips in mind, developers can create applications that are well-suited to handle the demands of today’s digital landscape.
Latest Topic
-
Cloud-Native Technologies: Best Practices
20 April, 2024 -
Generative AI with Llama 3: Shaping the Future
15 April, 2024 -
Mastering Llama 3: The Ultimate Guide
10 April, 2024
Category
- Assignment Help
- Homework Help
- Programming
- Trending Topics
- C Programming Assignment Help
- Art, Interactive, And Robotics
- Networked Operating Systems Programming
- Knowledge Representation & Reasoning Assignment Help
- Digital Systems Assignment Help
- Computer Design Assignment Help
- Artificial Life And Digital Evolution
- Coding and Fundamentals: Working With Collections
- UML Online Assignment Help
- Prolog Online Assignment Help
- Natural Language Processing Assignment Help
- Julia Assignment Help
- Golang Assignment Help
- Design Implementation Of Network Protocols
- Computer Architecture Assignment Help
- Object-Oriented Languages And Environments
- Coding Early Object and Algorithms: Java Coding Fundamentals
- Deep Learning In Healthcare Assignment Help
- Geometric Deep Learning Assignment Help
- Models Of Computation Assignment Help
- Systems Performance And Concurrent Computing
- Advanced Security Assignment Help
- Typescript Assignment Help
- Computational Media Assignment Help
- Design And Analysis Of Algorithms
- Geometric Modelling Assignment Help
- JavaScript Assignment Help
- MySQL Online Assignment Help
- Programming Practicum Assignment Help
- Public Policy, Legal, And Ethical Issues In Computing, Privacy, And Security
- Computer Vision
- Advanced Complexity Theory Assignment Help
- Big Data Mining Assignment Help
- Parallel Computing And Distributed Computing
- Law And Computer Science Assignment Help
- Engineering Distributed Objects For Cloud Computing
- Building Secure Computer Systems Assignment Help
- Ada Assignment Help
- R Programming Assignment Help
- Oracle Online Assignment Help
- Languages And Automata Assignment Help
- Haskell Assignment Help
- Economics And Computation Assignment Help
- ActionScript Assignment Help
- Audio Programming Assignment Help
- Bash Assignment Help
- Computer Graphics Assignment Help
- Groovy Assignment Help
- Kotlin Assignment Help
- Object Oriented Languages And Environments
- COBOL ASSIGNMENT HELP
- Bayesian Statistical Probabilistic Programming
- Computer Network Assignment Help
- Django Assignment Help
- Lambda Calculus Assignment Help
- Operating System Assignment Help
- Computational Learning Theory
- Delphi Assignment Help
- Concurrent Algorithms And Data Structures Assignment Help
- Machine Learning Assignment Help
- Human Computer Interface Assignment Help
- Foundations Of Data Networking Assignment Help
- Continuous Mathematics Assignment Help
- Compiler Assignment Help
- Computational Biology Assignment Help
- PostgreSQL Online Assignment Help
- Lua Assignment Help
- Human Computer Interaction Assignment Help
- Ethics And Responsible Innovation Assignment Help
- Communication And Ethical Issues In Computing
- Computer Science
- Combinatorial Optimisation Assignment Help
- Ethical Computing In Practice
- HTML Homework Assignment Help
- Linear Algebra Assignment Help
- Perl Assignment Help
- Artificial Intelligence Assignment Help
- Uncategorized
- Ethics And Professionalism Assignment Help
- Human Augmentics Assignment Help
- Linux Assignment Help
- PHP Assignment Help
- Assembly Language Assignment Help
- Dart Assignment Help
- Complete Python Bootcamp From Zero To Hero In Python Corrected Version
- Swift Assignment Help
- Computational Complexity Assignment Help
- Probability And Computing Assignment Help
- MATLAB Programming For Engineers
- Introduction To Statistical Learning
- Database Systems Implementation Assignment Help
- Computational Game Theory Assignment Help
- Database Assignment Help
- Probabilistic Model Checking Assignment Help
- Mathematics For Computer Science And Philosophy
- Introduction To Formal Proof Assignment Help
- Creative Coding Assignment Help
- Foundations Of Self-Programming Agents Assignment Help
- Machine Organization Assignment Help
- Software Design Assignment Help
- Data Communication And Networking Assignment Help
- Computational Biology
- Data Structure Assignment Help
- Foundations Of Software Engineering Assignment Help
- Mathematical Foundations Of Computing
- Principles Of Programming Languages Assignment Help
- Software Engineering Capstone Assignment Help
- Algorithms and Data Structures Assignment Help
No Comments