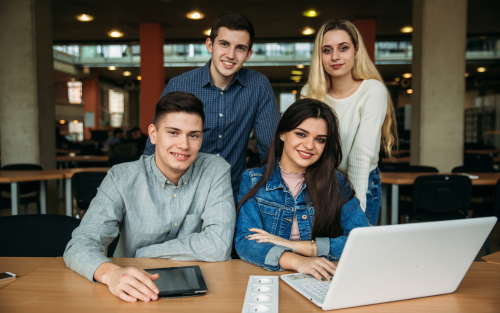
02 May Building A Simple Todo List App With Vue.Js
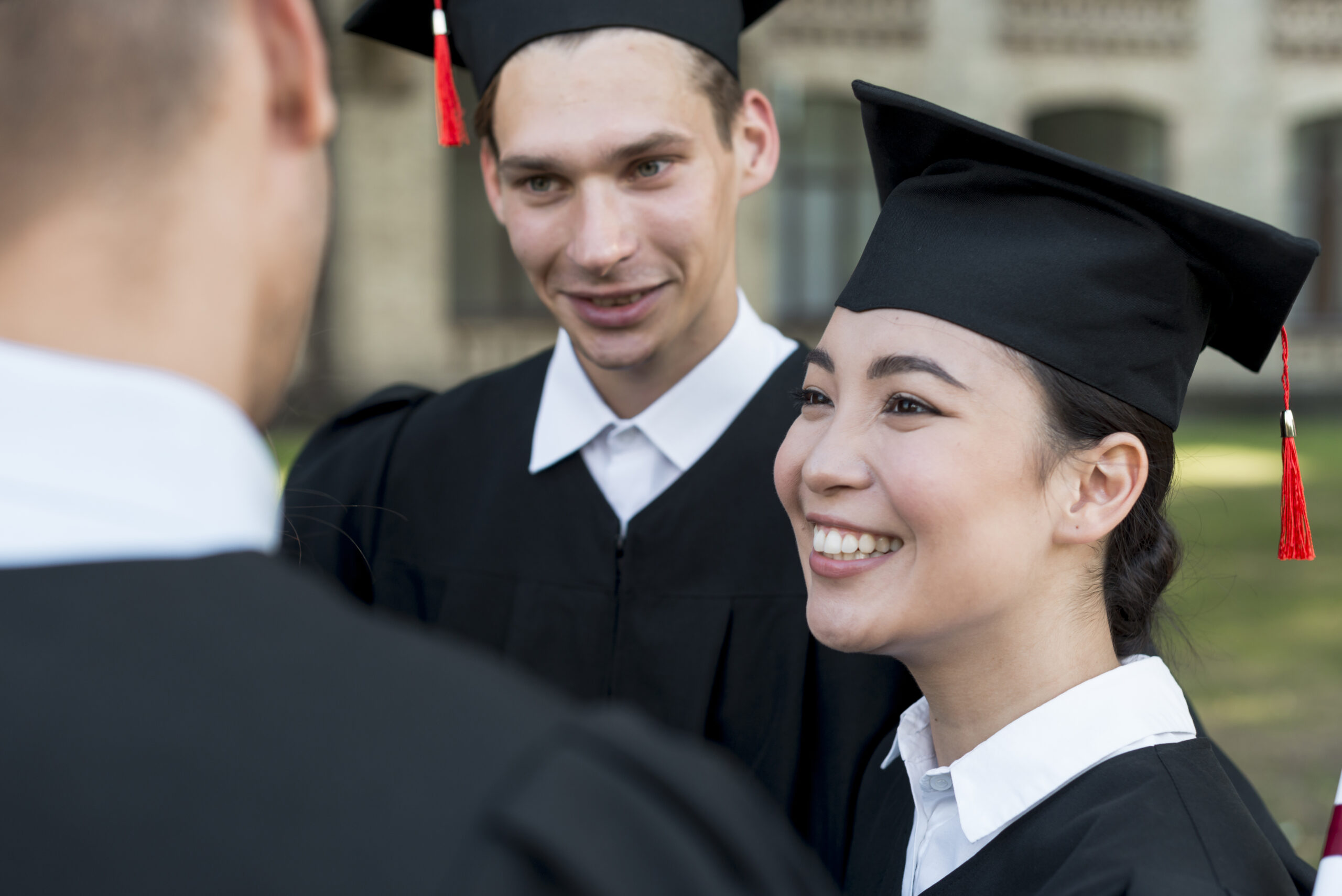
Introduction
Vue.js is a popular JavaScript framework that allows developers to create dynamic web applications. One of the many benefits of Vue.js is its simplicity, making it an excellent choice for creating small to medium-sized applications. In this tutorial, we will be building a simple Todo List app with Vue.js.
Learn how to build a simple todo list app using Vue.js, a popular JavaScript framework for building user interfaces. This tutorial guides you through the process of creating a responsive and interactive todo list application with Vue.js. Discover the basics of Vue.js components, data binding, and event handling. By the end of this tutorial, you’ll have a functioning todo list app that allows users to add, delete, and mark tasks as complete.
Building a simple todo list app with Vue.js allows you to experience the power and simplicity of this popular JavaScript framework. In this tutorial, you’ll learn the basics of Vue.js and how to leverage its features to create a responsive and interactive todo list application.
You’ll start by setting up a Vue.js project and creating the necessary components for the todo list app. You’ll explore data binding to display and update the task list dynamically. Event handling mechanisms will enable users to add new tasks, mark tasks as complete, and delete tasks.
The tutorial will cover essential Vue.js concepts such as computed properties for task filtering and dynamic rendering using directives. You’ll also learn about Vue.js form handling for adding new tasks and Vue.js lifecycle hooks for performing actions at specific stages of the component lifecycle.
Throughout the tutorial, you’ll gain insights into Vue.js best practices and recommended approaches for structuring your todo list app. You’ll have the opportunity to enhance the app by incorporating additional features such as task editing, task prioritization, or data persistence.
By the end of the tutorial, you’ll have a fully functional todo list app built with Vue.js, ready to be expanded or customized according to your needs. This project will provide you with a solid foundation in Vue.js frontend development and empower you to build more complex and interactive applications.
Building a simple todo list app with Vue.js is an excellent way to grasp the core concepts of this powerful JavaScript framework. Whether you’re new to Vue.js or looking to enhance your existing knowledge, this tutorial will equip you with the skills to create responsive and interactive user interfaces with ease.
Prerequisites
To follow this tutorial, you will need basic knowledge of HTML, CSS, and JavaScript. You should also have Node.js and Vue.js installed on your machine.
Setting Up the Project
To start, create a new directory for your project and open it in your preferred text editor. Next, create a new file named index.html and add the following code:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<meta http-equiv="X-UA-Compatible" content="ie=edge" />
<title>Todo List</title>
</head>
<body>
<div id="app">
<h1>Todo List</h1>
</div>
<script src=“https://cdn.jsdelivr.net/npm/vue”></script>
<script src=“app.js”></script>
</body>
</html>
This code sets up the basic HTML structure of our app and includes Vue.js and a JavaScript file named app.js that we will create later.
Creating the Vue Instance
In the app.js file, add the following code:
const app = new Vue({
el: '#app',
data: {
todos: [
{ id: 1, text: 'Buy milk' },
{ id: 2, text: 'Take out the trash' },
{ id: 3, text: 'Do laundry' },
],
},
});
This code creates a new Vue instance and sets its el property to ‘#app’, which tells Vue to render the app inside the element with the ID of app. We also define a data property called todos that contains an array of three objects representing our initial Todo items.
Displaying the Todo List
To display the Todo list, update the HTML code in index.html as follows:
<div id="app">
<h1>Todo List</h1>
<ul>
<li v-for="todo in todos" :key="todo.id">{{ todo.text }}</li>
</ul>
</div>
This code uses the v-for directive to loop through each Todo item in the todos array and display its text property in a list item.
Adding New Todos
To add new Todos, we’ll create a form with an input field and a button. Add the following code below the Todo list in index.html:
<form @submit.prevent="addTodo">
<input type="text" v-model="newTodo" placeholder="Enter a new Todo" />
<button>Add Todo</button>
</form>
This code creates a form with an input field and a button. We’ve added a v-model directive to the input field, which binds its value to the newTodo property on our Vue instance. The @submit.prevent directive prevents the default form submission behavior and calls the addTodo method when the form is submitted.
Next, add the addTodo method to the Vue instance:
const app = new Vue({
el: '#app',
data: {
todos: [
{ id: 1, text: 'Buy milk' },
{ id: 2, text: 'Take out the trash' },
{ id: 3, text: 'Do laundry' },
],
newTodo: '',
},
methods: {
addTodo() {
if (this.newTodo.trim() !== '') {
this.todos.push({
id: this.todos.length + 1,
text: this.newTodo,
});
this.newTodo = '';
}
},
},
});
This code defines the addTodo method, which checks if the newTodo property is not an empty string and adds a new Todo object to the todos array with a unique ID and the text entered in the input field. Finally, it resets the value of newTodo to an empty string.
Styling the App
Lastly, we’ll add some simple styling to our app to make it look a bit more presentable. Add the following CSS code to the head section of index.html:
<style>
#app {
max-width: 400px;
margin: 0 auto;
font-family: Arial, Helvetica, sans-serif;
text-align: center;
}
input[type=‘text’] {
padding: 8px;
font-size: 16px;
border-radius: 4px;
border: 1px solid #ccc;
}
button {
background-color: #4caf50;
color: #fff;
border: none;
padding: 8px 16px;
margin-left: 8px;
border-radius: 4px;
cursor: pointer;
}
ul {
list-style: none;
padding: 0;
margin: 16px 0;
text-align: left;
}
li {
margin: 8px 0;
padding: 8px;
border: 1px solid #ccc;
border-radius: 4px;
}
</style>
This code styles the app with a centered container, a styled input field and button, and a simple list style for the Todo items.
FAQs
What is Vue.js?
Vue.js is a progressive JavaScript framework for building user interfaces. It provides a simple and flexible syntax that allows developers to create interactive web applications.
Why should I use Vue.js for building a todo list app?
Vue.js is an excellent choice for building a todo list app due to its simplicity and reactivity. Vue.js’s reactive data-binding enables real-time updates to the user interface, making it easy to reflect changes in the todo list dynamically. Additionally, Vue.js offers a rich ecosystem of libraries and plugins that can enhance the development process.
How do I get started with building a todo list app using Vue.js?
To get started with building a todo list app with Vue.js, you can follow these steps:
- Install Vue.js using npm or include it via a CDN.
- Create a new Vue instance in your HTML file.
- Define the data properties for your todo list.
- Create methods to handle adding, editing, and deleting todo items.
- Use Vue directives to bind the data to the UI and handle user interactions.
How can I persist the todo list data?
You can persist the todo list data by using browser storage mechanisms such as LocalStorage or IndexedDB. These APIs allow you to store and retrieve data locally on the user’s device, ensuring that the todo list remains accessible even after page refreshes or browser restarts.
Can I customize the design and layout of the todo list app?
Yes, Vue.js allows for easy customization of the design and layout of your todo list app. You can use CSS frameworks like Bootstrap or Tailwind CSS, or create your own custom styles using CSS or CSS-in-JS libraries. Vue.js’s component-based architecture makes it straightforward to apply custom styles to individual components.
How can I handle user authentication and authorization in a todo list app?
User authentication and authorization can be implemented using various techniques in combination with Vue.js. You can leverage authentication services like Firebase Authentication or implement your own server-side authentication. Additionally, you can use Vue Router to define protected routes and restrict access to certain features based on user roles or permissions.
Can I add additional features to the todo list app, such as sorting or filtering?
Yes, Vue.js provides a rich set of features and libraries that can be easily integrated into your todo list app. For example, you can use Vue.js plugins like Vue Router for routing, Vuex for state management, and libraries like Lodash for sorting or filtering functionality. These additional features can enhance the user experience and make the todo list app more versatile.
Is it possible to deploy the todo list app to a web server or hosting platform?
Yes, you can deploy your Vue.js todo list app to a web server or hosting platform of your choice. Vue.js applications can be built into static files that can be hosted on services like GitHub Pages, Netlify, or AWS S3. You can also deploy Vue.js apps as server-rendered applications using frameworks like Nuxt.js.
Are there any performance considerations when building a todo list app with Vue.js?
While Vue.js is known for its excellent performance, there are still some performance considerations to keep in mind. Avoid unnecessary re-renders by using proper component lifecycle hooks and optimizing computed properties. Implement virtual scrolling or pagination for large todo lists to improve rendering performance. Additionally, bundle optimization techniques like code splitting and lazy-loading can reduce initial load times.
Are there any resources or tutorials available for building a todo list app with Vue.js?
Yes, there are plenty of resources and tutorials available for building a todo list app with Vue.js. You can find official documentation and guides on the Vue.js website. Additionally, there are numerous tutorials, blog posts, and video courses available online that provide step-by-step instructions and code examples to help you build a todo list app with Vue.js.
Conclusion
In this tutorial, we’ve built a simple Todo List app with Vue.js. We learned how to create a new Vue instance, display data in the app, and update that data with user input. We also added some simple styling to make our app look presentable. By following this tutorial, you should now have a basic understanding of how to build a Vue.js app and be able to apply these principles to create more complex applications.
Latest Topic
-
Cloud-Native Technologies: Best Practices
20 April, 2024 -
Generative AI with Llama 3: Shaping the Future
15 April, 2024 -
Mastering Llama 3: The Ultimate Guide
10 April, 2024
Category
- Assignment Help
- Homework Help
- Programming
- Trending Topics
- C Programming Assignment Help
- Art, Interactive, And Robotics
- Networked Operating Systems Programming
- Knowledge Representation & Reasoning Assignment Help
- Digital Systems Assignment Help
- Computer Design Assignment Help
- Artificial Life And Digital Evolution
- Coding and Fundamentals: Working With Collections
- UML Online Assignment Help
- Prolog Online Assignment Help
- Natural Language Processing Assignment Help
- Julia Assignment Help
- Golang Assignment Help
- Design Implementation Of Network Protocols
- Computer Architecture Assignment Help
- Object-Oriented Languages And Environments
- Coding Early Object and Algorithms: Java Coding Fundamentals
- Deep Learning In Healthcare Assignment Help
- Geometric Deep Learning Assignment Help
- Models Of Computation Assignment Help
- Systems Performance And Concurrent Computing
- Advanced Security Assignment Help
- Typescript Assignment Help
- Computational Media Assignment Help
- Design And Analysis Of Algorithms
- Geometric Modelling Assignment Help
- JavaScript Assignment Help
- MySQL Online Assignment Help
- Programming Practicum Assignment Help
- Public Policy, Legal, And Ethical Issues In Computing, Privacy, And Security
- Computer Vision
- Advanced Complexity Theory Assignment Help
- Big Data Mining Assignment Help
- Parallel Computing And Distributed Computing
- Law And Computer Science Assignment Help
- Engineering Distributed Objects For Cloud Computing
- Building Secure Computer Systems Assignment Help
- Ada Assignment Help
- R Programming Assignment Help
- Oracle Online Assignment Help
- Languages And Automata Assignment Help
- Haskell Assignment Help
- Economics And Computation Assignment Help
- ActionScript Assignment Help
- Audio Programming Assignment Help
- Bash Assignment Help
- Computer Graphics Assignment Help
- Groovy Assignment Help
- Kotlin Assignment Help
- Object Oriented Languages And Environments
- COBOL ASSIGNMENT HELP
- Bayesian Statistical Probabilistic Programming
- Computer Network Assignment Help
- Django Assignment Help
- Lambda Calculus Assignment Help
- Operating System Assignment Help
- Computational Learning Theory
- Delphi Assignment Help
- Concurrent Algorithms And Data Structures Assignment Help
- Machine Learning Assignment Help
- Human Computer Interface Assignment Help
- Foundations Of Data Networking Assignment Help
- Continuous Mathematics Assignment Help
- Compiler Assignment Help
- Computational Biology Assignment Help
- PostgreSQL Online Assignment Help
- Lua Assignment Help
- Human Computer Interaction Assignment Help
- Ethics And Responsible Innovation Assignment Help
- Communication And Ethical Issues In Computing
- Computer Science
- Combinatorial Optimisation Assignment Help
- Ethical Computing In Practice
- HTML Homework Assignment Help
- Linear Algebra Assignment Help
- Perl Assignment Help
- Artificial Intelligence Assignment Help
- Uncategorized
- Ethics And Professionalism Assignment Help
- Human Augmentics Assignment Help
- Linux Assignment Help
- PHP Assignment Help
- Assembly Language Assignment Help
- Dart Assignment Help
- Complete Python Bootcamp From Zero To Hero In Python Corrected Version
- Swift Assignment Help
- Computational Complexity Assignment Help
- Probability And Computing Assignment Help
- MATLAB Programming For Engineers
- Introduction To Statistical Learning
- Database Systems Implementation Assignment Help
- Computational Game Theory Assignment Help
- Database Assignment Help
- Probabilistic Model Checking Assignment Help
- Mathematics For Computer Science And Philosophy
- Introduction To Formal Proof Assignment Help
- Creative Coding Assignment Help
- Foundations Of Self-Programming Agents Assignment Help
- Machine Organization Assignment Help
- Software Design Assignment Help
- Data Communication And Networking Assignment Help
- Computational Biology
- Data Structure Assignment Help
- Foundations Of Software Engineering Assignment Help
- Mathematical Foundations Of Computing
- Principles Of Programming Languages Assignment Help
- Software Engineering Capstone Assignment Help
- Algorithms and Data Structures Assignment Help
No Comments