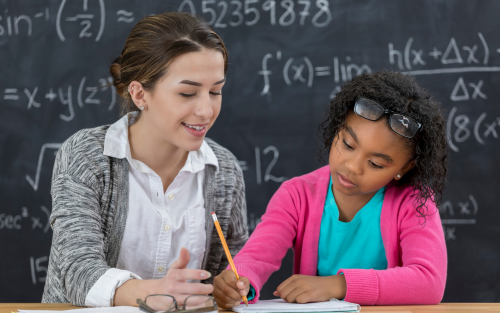
02 May Tips For Writing More Maintainable Javascript Code
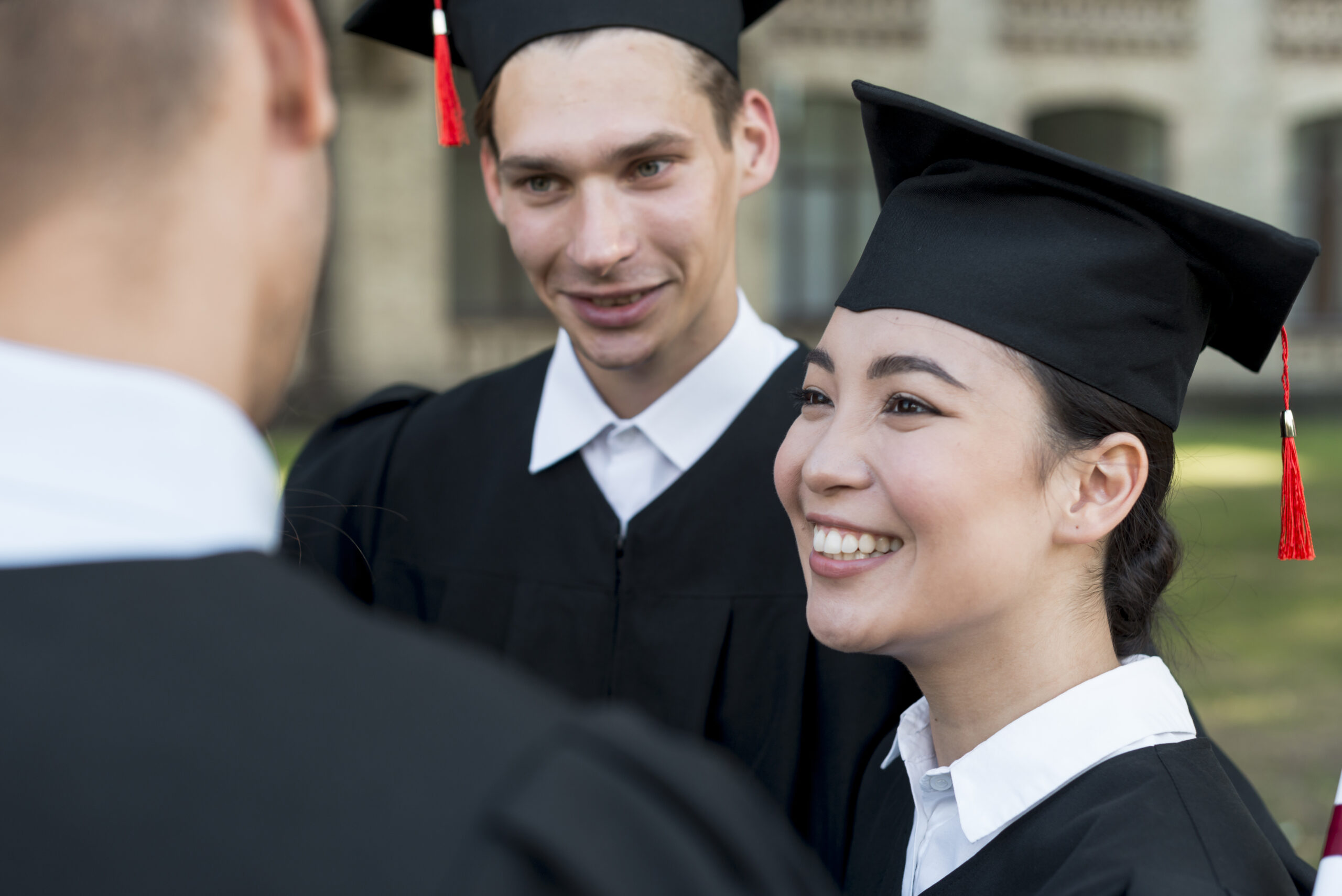
Javascript is one of the most popular programming languages, and it is used extensively in web development. Writing maintainable Javascript code is essential to ensure that your code is easy to read, understand, and modify. In this article, we will discuss some tips for writing more maintainable Javascript code.
Improve the reliability and maintainability of your JavaScript code with these valuable tips for writing more robust JavaScript applications. Follow best practices such as enabling strict mode, adhering to coding conventions, validating input data, handling errors gracefully, and optimizing code performance. Minimize the use of global variables, practice defensive programming, implement testing, and regularly refactor your code for better robustness. These tips will help you create JavaScript code that is less prone to errors, easier to maintain, and delivers a seamless user experience.
Tips For Writing More Robust JavaScript Code
Write modular code: One of the best ways to write maintainable code is by breaking your code into smaller, more manageable modules. This makes your code more readable and understandable, and it also makes it easier to maintain. Use modules to encapsulate functionality and keep related code together.
Use descriptive variable and function names: One of the most important aspects of writing maintainable code is using descriptive variable and function names. This makes your code easier to understand, especially when it is read by someone who did not write the code. Avoid using abbreviations or single-letter variable names, and instead use descriptive names that clearly convey what the variable or function does.
Write comments: Comments are a great way to add context to your code and explain what is happening. They can also be used to indicate the purpose of a function or variable, which can be helpful when someone else needs to read and understand your code. Make sure to write comments that are clear, concise, and easy to understand.
Avoid global variables: Global variables can make your code difficult to maintain and debug, especially in large projects. Instead, try to keep your variables as local as possible and limit the scope of your functions. This makes it easier to identify where variables are being used and reduces the chances of naming collisions.
Use consistent coding style: Consistent coding style makes your code more readable and easier to maintain. Consistency can be achieved by using the same naming conventions, formatting, and indentation throughout your codebase. You can use tools like ESLint to enforce a consistent coding style across your project.
Use meaningful whitespace: Using whitespace to separate blocks of code can make it easier to read and understand. Use whitespace to break up large blocks of code and separate logical sections of your code. This makes your code more readable and easier to maintain.
Use modern language features: JavaScript is constantly evolving, and new features are being added with each new version of the language. Using modern language features can make your code more concise, readable, and maintainable. For example, use arrow functions instead of traditional function declarations, and use let and const instead of var for variable declarations.
Write testable code: Writing testable code is essential for maintaining and debugging your code. Write code that is modular and has clear inputs and outputs. This makes it easier to write unit tests, which can help you catch bugs and ensure that your code is working as expected.
Use a consistent coding standard: Using a consistent coding standard can make your code more maintainable and reduce the chances of errors. It is important to agree on a coding standard with your team and ensure that everyone follows it. This makes it easier to read, understand, and maintain each other’s code.
Document your code: Documenting your code is essential for maintaining and debugging it. Use tools like JSDoc to add comments to your code that describe what each function does, what parameters it expects, and what it returns. This makes it easier for other developers to understand and use your code.
FAQs: Tips For Writing More Robust JavaScript Code
Why is writing robust JavaScript code important?
Writing robust JavaScript code is essential for creating reliable and maintainable applications. It helps prevent errors, handle unexpected situations gracefully, and ensures the code can withstand changes and updates over time. Robust code improves the overall quality, stability, and longevity of the software.
What are some tips for writing more robust JavaScript code?
- Use strict mode: Enable strict mode in your JavaScript files to catch common mistakes and enforce stricter coding rules.
- Validate input data: Always validate and sanitize input data to prevent security vulnerabilities and ensure the code can handle unexpected values.
- Handle errors gracefully: Implement error handling mechanisms, such as try-catch blocks, to catch and handle exceptions properly. This prevents application crashes and improves the user experience.
- Follow coding conventions: Stick to consistent coding conventions and style guidelines to enhance code readability and maintainability. This makes it easier for others to understand and work with your code.
- Break down complex tasks: Divide complex tasks into smaller, manageable functions and modules. This promotes code reusability, testability, and makes troubleshooting and debugging easier.
- Use meaningful variable and function names: Choose descriptive names for variables and functions that accurately convey their purpose and functionality. This improves code readability and reduces the likelihood of confusion or bugs.
- Avoid global variables: Minimize the use of global variables to prevent variable conflicts and unintended side effects. Encapsulate variables within appropriate scopes whenever possible.
- Write unit tests: Create comprehensive unit tests to verify the functionality of your code and catch potential bugs early on. Automated testing helps ensure that changes to the codebase do not introduce regressions.
- Document your code: Add comments and documentation to explain the purpose, functionality, and usage of your code. This helps other developers understand and maintain the code in the future.
- Regularly refactor and optimize: Continuously refactor your code to improve its structure and efficiency. Identify and eliminate code smells, optimize performance bottlenecks, and adhere to best practices.
How can I ensure cross-browser compatibility for robust JavaScript code?
To ensure cross-browser compatibility, follow these practices:
- Test your code on multiple browsers and versions to identify and address compatibility issues.
- Use feature detection rather than relying on browser-specific features or behaviors.
- Consider using polyfills or shims to provide missing functionality in older browsers.
- Leverage popular JavaScript libraries or frameworks that handle cross-browser compatibility for you.
What tools are available for linting and code analysis in JavaScript?
There are several tools available for linting and code analysis in JavaScript, including ESLint, JSLint, and JSHint. These tools can identify potential issues, enforce coding conventions, and help you write cleaner and more robust code.
Is code optimization important for writing robust JavaScript code?
Code optimization can improve the performance and efficiency of your JavaScript code, but it is not directly related to robustness. However, optimizing your code can indirectly contribute to robustness by reducing the likelihood of performance issues, memory leaks, or unexpected behaviors.
Should I use design patterns in JavaScript to write more robust code?
Design patterns can be beneficial for writing more robust JavaScript code as they provide proven solutions to common software design problems. Patterns like the Module pattern, Observer pattern, and Singleton pattern can help improve code organization, encapsulation, and maintainability.
How can I handle asynchronous operations in JavaScript to ensure robustness?
JavaScript provides several mechanisms for handling asynchronous operations, such as callbacks, Promises, and async/await. By properly handling asynchronous operations and managing errors, you can ensure that your code remains robust and responsive, even in asynchronous environments.
Can using a package manager like npm enhance code robustness?
Using a package manager like npm can enhance code robustness by providing access to a vast ecosystem of reliable and well-tested JavaScript packages. Leveraging established packages saves time and reduces the risk of introducing bugs or reinventing the wheel.
Are there any common pitfalls to avoid when writing robust JavaScript code?
Some common pitfalls to avoid include:
- Not handling error cases or failing to provide meaningful error messages.
- Neglecting input validation and not sanitizing user input, leading to security vulnerabilities.
- Relying too heavily on global variables, leading to potential conflicts and unexpected behavior.
- Not properly handling memory management, which can result in memory leaks.
- Overcomplicating code by not following the principle of “Keep It Simple, Stupid” (KISS).
How can I ensure code maintainability while focusing on robustness?
Maintainability and robustness often go hand in hand. By following best practices for writing robust code, such as modularizing your code, using meaningful names, and documenting thoroughly, you can improve code maintainability. Additionally, writing unit tests and regularly refactoring your code can ensure that it remains manageable and adaptable over time.
Conclusion
Writing maintainable JavaScript code is essential for ensuring that your code is easy to read, understand, and modify. By following the tips outlined in this article, you can make your code more maintainable, reduce the chances of errors, and improve the overall quality of your code. Remember to write modular code, use descriptive variable and function names, write comments, avoid global variables, use consistent coding style, use meaningful whitespace, use modern language features,
Latest Topic
-
Cloud-Native Technologies: Best Practices
20 April, 2024 -
Generative AI with Llama 3: Shaping the Future
15 April, 2024 -
Mastering Llama 3: The Ultimate Guide
10 April, 2024
Category
- Assignment Help
- Homework Help
- Programming
- Trending Topics
- C Programming Assignment Help
- Art, Interactive, And Robotics
- Networked Operating Systems Programming
- Knowledge Representation & Reasoning Assignment Help
- Digital Systems Assignment Help
- Computer Design Assignment Help
- Artificial Life And Digital Evolution
- Coding and Fundamentals: Working With Collections
- UML Online Assignment Help
- Prolog Online Assignment Help
- Natural Language Processing Assignment Help
- Julia Assignment Help
- Golang Assignment Help
- Design Implementation Of Network Protocols
- Computer Architecture Assignment Help
- Object-Oriented Languages And Environments
- Coding Early Object and Algorithms: Java Coding Fundamentals
- Deep Learning In Healthcare Assignment Help
- Geometric Deep Learning Assignment Help
- Models Of Computation Assignment Help
- Systems Performance And Concurrent Computing
- Advanced Security Assignment Help
- Typescript Assignment Help
- Computational Media Assignment Help
- Design And Analysis Of Algorithms
- Geometric Modelling Assignment Help
- JavaScript Assignment Help
- MySQL Online Assignment Help
- Programming Practicum Assignment Help
- Public Policy, Legal, And Ethical Issues In Computing, Privacy, And Security
- Computer Vision
- Advanced Complexity Theory Assignment Help
- Big Data Mining Assignment Help
- Parallel Computing And Distributed Computing
- Law And Computer Science Assignment Help
- Engineering Distributed Objects For Cloud Computing
- Building Secure Computer Systems Assignment Help
- Ada Assignment Help
- R Programming Assignment Help
- Oracle Online Assignment Help
- Languages And Automata Assignment Help
- Haskell Assignment Help
- Economics And Computation Assignment Help
- ActionScript Assignment Help
- Audio Programming Assignment Help
- Bash Assignment Help
- Computer Graphics Assignment Help
- Groovy Assignment Help
- Kotlin Assignment Help
- Object Oriented Languages And Environments
- COBOL ASSIGNMENT HELP
- Bayesian Statistical Probabilistic Programming
- Computer Network Assignment Help
- Django Assignment Help
- Lambda Calculus Assignment Help
- Operating System Assignment Help
- Computational Learning Theory
- Delphi Assignment Help
- Concurrent Algorithms And Data Structures Assignment Help
- Machine Learning Assignment Help
- Human Computer Interface Assignment Help
- Foundations Of Data Networking Assignment Help
- Continuous Mathematics Assignment Help
- Compiler Assignment Help
- Computational Biology Assignment Help
- PostgreSQL Online Assignment Help
- Lua Assignment Help
- Human Computer Interaction Assignment Help
- Ethics And Responsible Innovation Assignment Help
- Communication And Ethical Issues In Computing
- Computer Science
- Combinatorial Optimisation Assignment Help
- Ethical Computing In Practice
- HTML Homework Assignment Help
- Linear Algebra Assignment Help
- Perl Assignment Help
- Artificial Intelligence Assignment Help
- Uncategorized
- Ethics And Professionalism Assignment Help
- Human Augmentics Assignment Help
- Linux Assignment Help
- PHP Assignment Help
- Assembly Language Assignment Help
- Dart Assignment Help
- Complete Python Bootcamp From Zero To Hero In Python Corrected Version
- Swift Assignment Help
- Computational Complexity Assignment Help
- Probability And Computing Assignment Help
- MATLAB Programming For Engineers
- Introduction To Statistical Learning
- Database Systems Implementation Assignment Help
- Computational Game Theory Assignment Help
- Database Assignment Help
- Probabilistic Model Checking Assignment Help
- Mathematics For Computer Science And Philosophy
- Introduction To Formal Proof Assignment Help
- Creative Coding Assignment Help
- Foundations Of Self-Programming Agents Assignment Help
- Machine Organization Assignment Help
- Software Design Assignment Help
- Data Communication And Networking Assignment Help
- Computational Biology
- Data Structure Assignment Help
- Foundations Of Software Engineering Assignment Help
- Mathematical Foundations Of Computing
- Principles Of Programming Languages Assignment Help
- Software Engineering Capstone Assignment Help
- Algorithms and Data Structures Assignment Help
No Comments