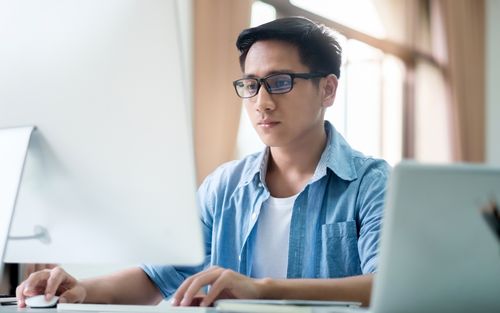
09 May Variables And Data Types In Python
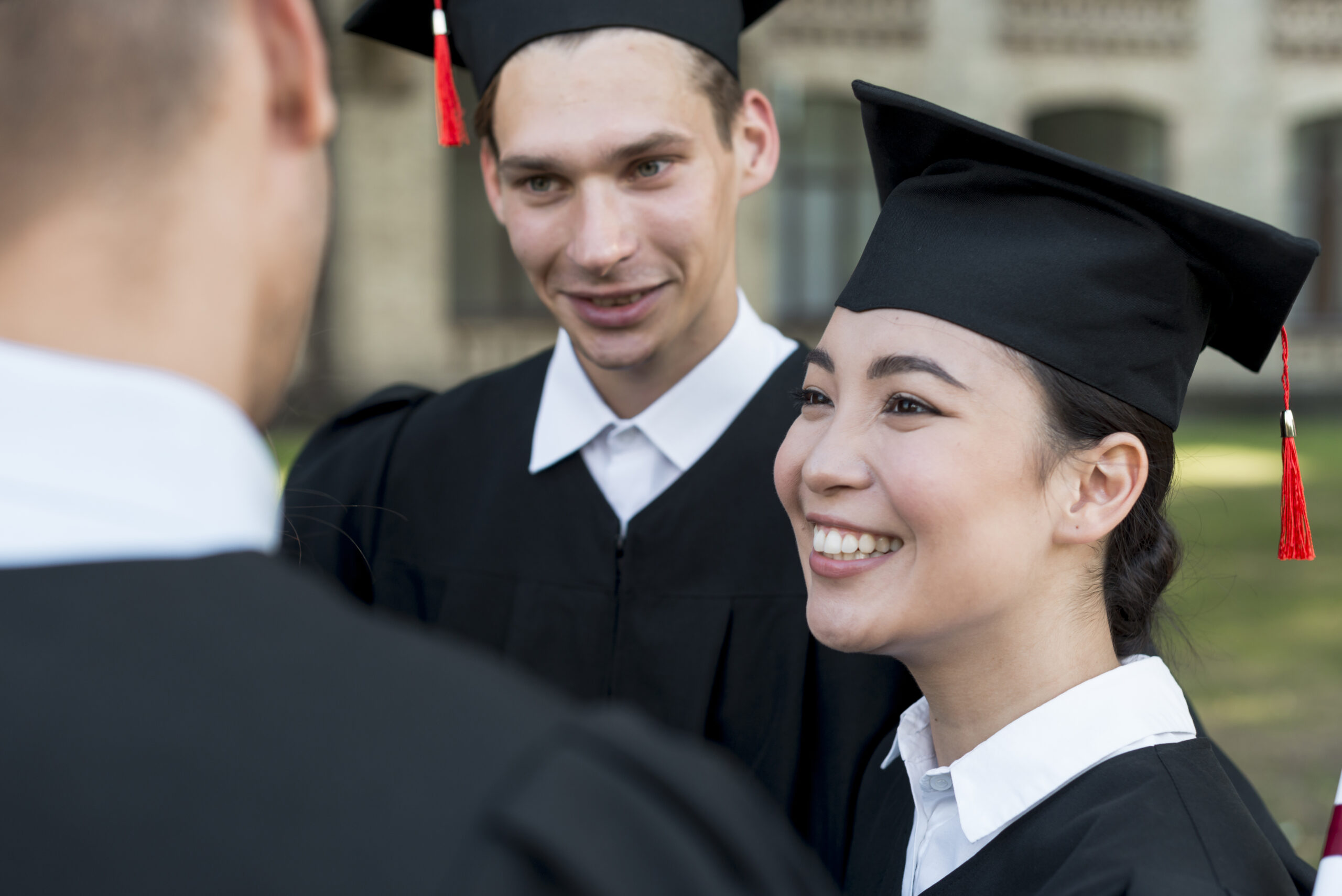
Introduction
Python is a high-level, interpreted programming language that is widely used in a variety of applications, including web development, scientific computing, artificial intelligence, data analysis, and more. Python offers a wide range of data types that allow programmers to work with different types of data, including integers, strings, lists, tuples, and dictionaries. In this article, we will discuss variables and data types in Python in detail.
What are Variables?
Variables are the memory locations in a program that hold values that can be modified during the execution of the program. In Python, variables are created when they are first assigned a value. The assignment operator (=) is used to assign a value to a variable.
For example, consider the following code snippet:
x = 10
print(x)
In this code snippet, we are creating a variable x
and assigning the value 10 to it. We are then printing the value of x
using the print()
function. The output of this code snippet will be:
10
Here, the variable x
holds the value 10, which can be used later in the program.
Data Types in Python:
Python supports a wide range of data types that allow programmers to work with different types of data. Let’s discuss the most commonly used data types in Python.
Numbers: Python supports three types of numerical data types: integers, floating-point numbers, and complex numbers.
Integers: Integers are whole numbers without a decimal point. For example, 1, 2, 3, 4, 5, etc., are integers in Python. To create an integer variable in Python, we simply assign an integer value to a variable. For example:
x = 10
Here, we are creating an integer variable x
and assigning the value 10 to it.
Floating-point numbers: Floating-point numbers are decimal numbers. For example, 1.23, 3.14, etc., are floating-point numbers in Python. To create a floating-point variable in Python, we simply assign a floating-point value to a variable. For example:
x = 3.14
Here, we are creating a floating-point variable x
and assigning the value 3.14 to it.
Complex numbers: Complex numbers are numbers with a real and imaginary part. To create a complex number in Python, we use the j
or J
suffix to denote the imaginary part. For example:
x = 2 + 3j
Here, we are creating a complex number variable x
with a real part of 2 and an imaginary part of 3.
Strings: Strings are used to represent textual data in Python. A string is a sequence of characters enclosed in single or double quotes. For example:
x = 'Hello, World!'
Here, we are creating a string variable x
with the value ‘Hello, World!’. We can also use double quotes to create a string:
x = "Hello, World!"
Here, we are creating a string variable x
with the same value as before.
Lists: Lists are used to store a collection of items in Python. A list can contain items of different data types, including numbers, strings, and other lists. To create a list in Python, we enclose the items in square brackets, separated by commas. For example:
x = [1, 2, 3, 'four', 'five']
Here, we are creating a list variable x
with five items: three integers and two strings.
Tuples: Tuples are similar to lists in that they can store a collection of items, but unlike lists, they are immutable, meaning their values cannot be changed once they are created. To create a tuple in Python, we enclose the items in parentheses, separated by commas. For example:
x = (1, 2, 3, 'four', 'five')
Here, we are creating a tuple variable x
with five items: three integers and two strings.
Dictionaries: Dictionaries are used to store a collection of key-value pairs in Python. Each key is associated with a value, and we can use the key to retrieve the corresponding value. To create a dictionary in Python, we enclose the key-value pairs in curly braces, separated by colons. For example:
x = {'name': 'John', 'age': 30, 'city': 'New York'}
Here, we are creating a dictionary variable x
with three key-value pairs: ‘name’: ‘John’, ‘age’: 30, and ‘city’: ‘New York’. We can access the value associated with a key using the square bracket notation. For example:
print(x['name']) # Output: John
Here, we are printing the value associated with the key ‘name’ in the dictionary x
.
Type Conversion: In Python, we can convert one data type to another using type conversion functions. Let’s discuss some of the commonly used type conversion functions in Python.
-int(): The int()
function is used to convert a value to an integer data type. For example:
x = '10'
y = int(x)
print(y) # Output: 10
Here, we are converting the string value ’10’ to an integer value using the int()
function.
-float(): The float()
function is used to convert a value to a floating-point data type. For example:
x = '3.14'
y = float(x)
print(y) # Output: 3.14
Here, we are converting the string value ‘3.14’ to a floating-point value using the float()
function.
str(): The str()
function is used to convert a value to a string data type. For example:
x = 10
y = str(x)
print(y) # Output: '10'
Here, we are converting the integer value 10 to a string value using the str()
function.
list(): The list()
function is used to convert a value to a list data type. For example:
x = (1, 2, 3)
y = list(x)
print(y) # Output: [1, 2, 3]
Here, we are converting the tuple (1, 2, 3)
to a list [1, 2, 3]
using the list()
function.
Case Study
Let’s consider an example of using data types in Python in a real-world scenario. A company wants to store its employee data, including their names, ages, addresses, and salaries. They want to be able to add new employees, remove existing ones, and retrieve employee data as required.
To achieve this, we can use a dictionary in Python to store the employee data. Each key in the dictionary represents an employee ID, and its corresponding value is another dictionary that stores the employee’s details. For example:
employees = {
1: {'name': 'John', 'age': 30, 'address': '123 Main St', 'salary': 50000},
2: {'name': 'Jane', 'age': 25, 'address': '456 Elm St', 'salary': 60000},
3: {'name': 'Bob', 'age': 40, 'address': '789 Oak St', 'salary': 70000}
}
Here, we have created a dictionary employees
with three key-value pairs, where each value is another dictionary that stores an employee’s details. We can add a new employee to the dictionary by simply assigning a new key-value pair, like so:
employees[4] = {'name': 'Alice', 'age': 35, 'address': '321 Maple St', 'salary': 80000}
We can remove an existing employee from the dictionary using the del
statement, like so:
del employees[2]
We can retrieve an employee’s details by using the square bracket notation to access the dictionary’s key-value pairs, like so:
print(employees[1]['name']) # Output: John
Here, we are printing the name of the employee with ID 1.
Quizz:
What is a variable in Python?
What are the commonly used data types in Python?
How do you create a tuple in Python?
What is a dictionary in Python?
What is type conversion in Python?
Answers:
-A variable in Python is a named memory location that stores a value.
-The commonly used data types in Python are numbers, strings, lists, tuples, and dictionaries.
-To create a tuple in Python, we enclose the items in parentheses, separated by commas. For example: x = (1, 2, 3, 'four', 'five')
-A dictionary in Python is used to store a collection of key-value pairs. Each key is associated with a value, and we can use the key to retrieve the corresponding value.
-Type conversion in Python is the process of converting one data type to another using type conversion functions like int()
, float()
, str()
, and list()
.
FAQ’s
Q: What is the difference between a list and a tuple in Python? A: A list is a mutable data type in Python, meaning its values can be changed once it is created. In contrast, a tuple is an immutable data type in Python, meaning its values cannot be changed once it is created.
Q: What is the use of dictionaries in Python? A: Dictionaries in Python are used to store a collection of key-value pairs, where each key is associated with a value. Dictionaries provide a convenient way to access and manipulate data using keys.
Q: Can we store different data types in a list or a tuple in Python? A: Yes, both lists and tuples in Python can store different data types, including numbers, strings, and other data structures like lists and tuples.
Conclusion
In Python, data types are an essential concept that allows us to store and manipulate different types of data. We have covered various data types, including numbers, strings, lists, tuples, and dictionaries, and discussed how to work with them in Python. We have also seen how to create and use variables to store data, and how to convert between different data types using type conversion functions.
Understanding data types in Python is crucial for developing any software application that involves working with data. Python’s rich set of built-in data types and its flexibility in working with them makes it an ideal choice for a wide range of applications, from web development to scientific computing.
In summary, mastering data types in Python is a fundamental step in becoming a proficient Python developer, and it is worth investing time and effort in learning them thoroughly.
Latest Topic
-
Cloud-Native Technologies: Best Practices
20 April, 2024 -
Generative AI with Llama 3: Shaping the Future
15 April, 2024 -
Mastering Llama 3: The Ultimate Guide
10 April, 2024
Category
- Assignment Help
- Homework Help
- Programming
- Trending Topics
- C Programming Assignment Help
- Art, Interactive, And Robotics
- Networked Operating Systems Programming
- Knowledge Representation & Reasoning Assignment Help
- Digital Systems Assignment Help
- Computer Design Assignment Help
- Artificial Life And Digital Evolution
- Coding and Fundamentals: Working With Collections
- UML Online Assignment Help
- Prolog Online Assignment Help
- Natural Language Processing Assignment Help
- Julia Assignment Help
- Golang Assignment Help
- Design Implementation Of Network Protocols
- Computer Architecture Assignment Help
- Object-Oriented Languages And Environments
- Coding Early Object and Algorithms: Java Coding Fundamentals
- Deep Learning In Healthcare Assignment Help
- Geometric Deep Learning Assignment Help
- Models Of Computation Assignment Help
- Systems Performance And Concurrent Computing
- Advanced Security Assignment Help
- Typescript Assignment Help
- Computational Media Assignment Help
- Design And Analysis Of Algorithms
- Geometric Modelling Assignment Help
- JavaScript Assignment Help
- MySQL Online Assignment Help
- Programming Practicum Assignment Help
- Public Policy, Legal, And Ethical Issues In Computing, Privacy, And Security
- Computer Vision
- Advanced Complexity Theory Assignment Help
- Big Data Mining Assignment Help
- Parallel Computing And Distributed Computing
- Law And Computer Science Assignment Help
- Engineering Distributed Objects For Cloud Computing
- Building Secure Computer Systems Assignment Help
- Ada Assignment Help
- R Programming Assignment Help
- Oracle Online Assignment Help
- Languages And Automata Assignment Help
- Haskell Assignment Help
- Economics And Computation Assignment Help
- ActionScript Assignment Help
- Audio Programming Assignment Help
- Bash Assignment Help
- Computer Graphics Assignment Help
- Groovy Assignment Help
- Kotlin Assignment Help
- Object Oriented Languages And Environments
- COBOL ASSIGNMENT HELP
- Bayesian Statistical Probabilistic Programming
- Computer Network Assignment Help
- Django Assignment Help
- Lambda Calculus Assignment Help
- Operating System Assignment Help
- Computational Learning Theory
- Delphi Assignment Help
- Concurrent Algorithms And Data Structures Assignment Help
- Machine Learning Assignment Help
- Human Computer Interface Assignment Help
- Foundations Of Data Networking Assignment Help
- Continuous Mathematics Assignment Help
- Compiler Assignment Help
- Computational Biology Assignment Help
- PostgreSQL Online Assignment Help
- Lua Assignment Help
- Human Computer Interaction Assignment Help
- Ethics And Responsible Innovation Assignment Help
- Communication And Ethical Issues In Computing
- Computer Science
- Combinatorial Optimisation Assignment Help
- Ethical Computing In Practice
- HTML Homework Assignment Help
- Linear Algebra Assignment Help
- Perl Assignment Help
- Artificial Intelligence Assignment Help
- Uncategorized
- Ethics And Professionalism Assignment Help
- Human Augmentics Assignment Help
- Linux Assignment Help
- PHP Assignment Help
- Assembly Language Assignment Help
- Dart Assignment Help
- Complete Python Bootcamp From Zero To Hero In Python Corrected Version
- Swift Assignment Help
- Computational Complexity Assignment Help
- Probability And Computing Assignment Help
- MATLAB Programming For Engineers
- Introduction To Statistical Learning
- Database Systems Implementation Assignment Help
- Computational Game Theory Assignment Help
- Database Assignment Help
- Probabilistic Model Checking Assignment Help
- Mathematics For Computer Science And Philosophy
- Introduction To Formal Proof Assignment Help
- Creative Coding Assignment Help
- Foundations Of Self-Programming Agents Assignment Help
- Machine Organization Assignment Help
- Software Design Assignment Help
- Data Communication And Networking Assignment Help
- Computational Biology
- Data Structure Assignment Help
- Foundations Of Software Engineering Assignment Help
- Mathematical Foundations Of Computing
- Principles Of Programming Languages Assignment Help
- Software Engineering Capstone Assignment Help
- Algorithms and Data Structures Assignment Help
No Comments