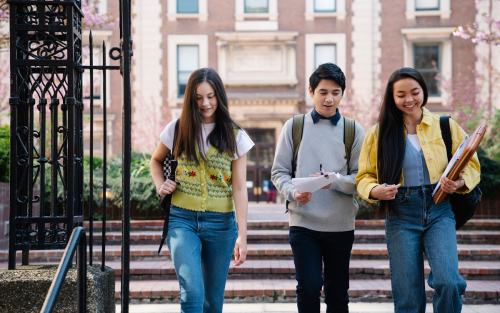
26 Apr Best Practices For Debugging JavaScript
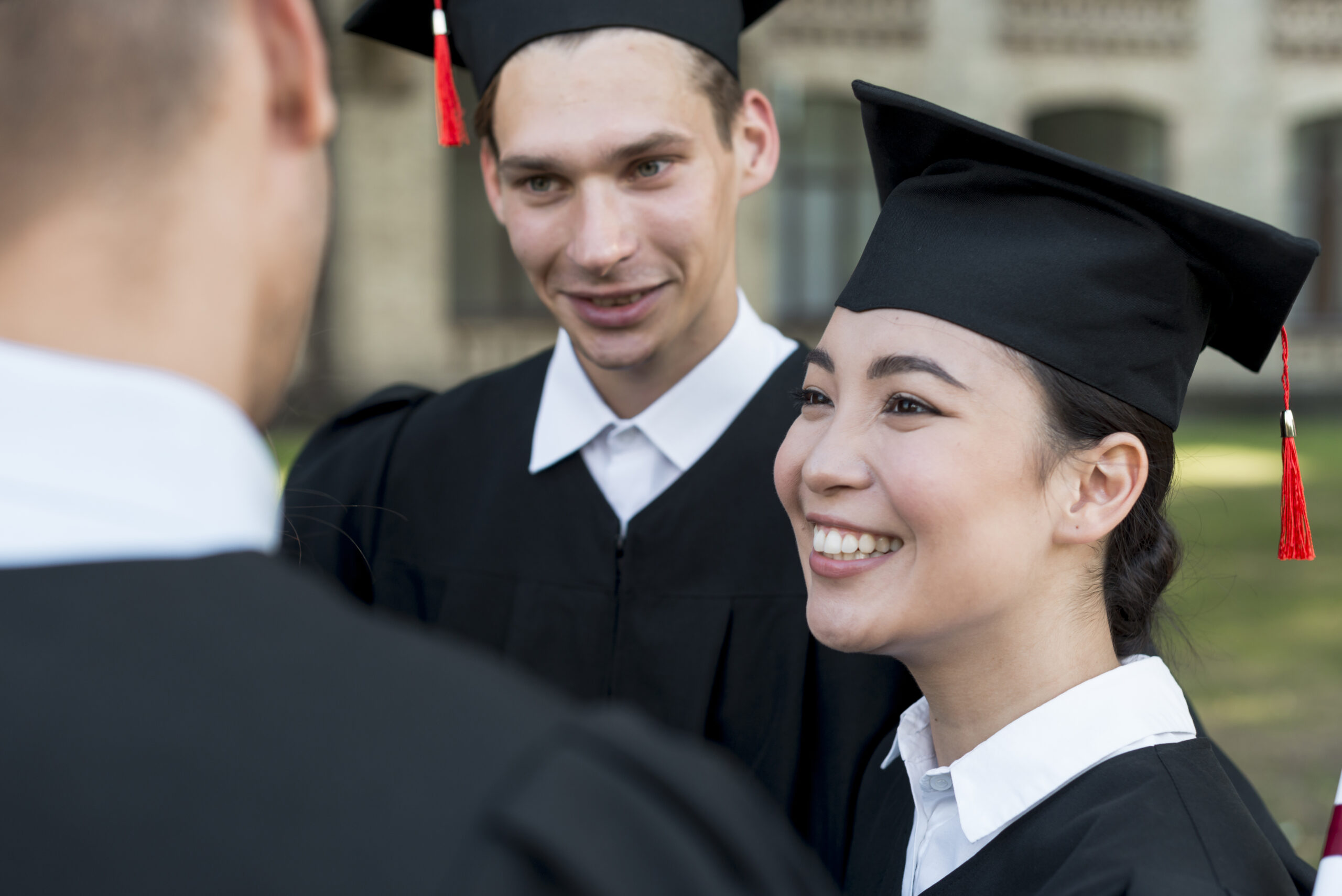
JavaScript is an incredibly versatile and powerful programming language that is used extensively in web development. However, with great power comes great responsibility, and debugging JavaScript code can be a time-consuming and frustrating process.
In this article, we will explore some best practices for debugging JavaScript code that can help you save time and avoid common mistakes.
Use console.log()
One of the most basic and essential tools for debugging JavaScript code is the console.log() function. This function allows you to output messages to the browser console, which can be used to track the state of variables and functions during runtime.
For example, if you want to check the value of a variable, you can use the console.log() function to output it to the console:
let x = 10;
console.log(x); // outputs 10 to the console
You can also use console.log() to output strings and other data types to the console.
Use breakpoints
Breakpoints are another powerful tool for debugging JavaScript code. They allow you to pause the execution of your code at a specific point and inspect the values of variables and functions.
You can set breakpoints in your code using the debugger keyword:
function multiply(x, y) {
debugger;
return x * y;
}
let result = multiply(2, 3);
console.log(result);
When the code runs, it will pause at the debugger statement, allowing you to inspect the values of x and y.
Use the browser’s developer tools
Modern web browsers come with built-in developer tools that allow you to inspect and debug JavaScript code. These tools can be accessed by pressing F12 or by right-clicking on a webpage and selecting “Inspect Element”.
Once you have opened the developer tools, you can use them to inspect the HTML, CSS, and JavaScript code of a webpage. You can also use the console to execute JavaScript code and debug your scripts.
Use try-catch statements
When your JavaScript code encounters an error, it will typically stop executing, which can make debugging difficult. To prevent this, you can use try-catch statements to catch and handle errors.
try {
// code that might throw an error
} catch (error) {
// code to handle the error
}
By wrapping your code in a try-catch statement, you can prevent your code from stopping when an error occurs, and instead handle the error gracefully.
Use descriptive variable names
One common mistake that developers make when writing JavaScript code is using cryptic or unclear variable names. This can make it difficult to understand and debug your code, especially when working with large codebases.
To avoid this, use descriptive variable names that clearly indicate what the variable represents. This will make your code easier to read, understand, and debug.
Use linting tools
Linting tools are automated tools that analyze your code for errors and potential problems. They can help you identify syntax errors, unused variables, and other issues that can make your code difficult to debug.
Some popular JavaScript linting tools include JSLint, ESLint, and JSHint. These tools can be integrated into your development workflow, allowing you to catch errors and potential issues before they cause problems.
Test your code
Finally, one of the best ways to avoid bugs and other issues in your JavaScript code is to test it thoroughly. This can involve writing unit tests, integration tests, and other types of tests to ensure that your code works as expected.
Testing can be a time-consuming process, but it can save you a lot of time and frustration in the long run by helping you catch and fix issues early on.
Conclusion
In conclusion, debugging is an essential skill for any JavaScript developer, and understanding best practices can significantly improve the efficiency and effectiveness of the process. By adopting a systematic approach and using the right tools, developers can quickly identify and resolve issues, leading to better-performing, more reliable applications.
Some of the best practices for debugging JavaScript include using a debugger, console logs, and error messages, isolating the problem, and checking for common mistakes. Additionally, staying up to date with the latest tools and techniques can further improve the debugging process.
By following these best practices, developers can not only solve issues faster but also prevent them from occurring in the first place. Debugging may be challenging, but with the right mindset and approach, it can be a rewarding experience that helps create better applications.
Latest Topic
-
Cloud-Native Technologies: Best Practices
20 April, 2024 -
Generative AI with Llama 3: Shaping the Future
15 April, 2024 -
Mastering Llama 3: The Ultimate Guide
10 April, 2024
Category
- Assignment Help
- Homework Help
- Programming
- Trending Topics
- C Programming Assignment Help
- Art, Interactive, And Robotics
- Networked Operating Systems Programming
- Knowledge Representation & Reasoning Assignment Help
- Digital Systems Assignment Help
- Computer Design Assignment Help
- Artificial Life And Digital Evolution
- Coding and Fundamentals: Working With Collections
- UML Online Assignment Help
- Prolog Online Assignment Help
- Natural Language Processing Assignment Help
- Julia Assignment Help
- Golang Assignment Help
- Design Implementation Of Network Protocols
- Computer Architecture Assignment Help
- Object-Oriented Languages And Environments
- Coding Early Object and Algorithms: Java Coding Fundamentals
- Deep Learning In Healthcare Assignment Help
- Geometric Deep Learning Assignment Help
- Models Of Computation Assignment Help
- Systems Performance And Concurrent Computing
- Advanced Security Assignment Help
- Typescript Assignment Help
- Computational Media Assignment Help
- Design And Analysis Of Algorithms
- Geometric Modelling Assignment Help
- JavaScript Assignment Help
- MySQL Online Assignment Help
- Programming Practicum Assignment Help
- Public Policy, Legal, And Ethical Issues In Computing, Privacy, And Security
- Computer Vision
- Advanced Complexity Theory Assignment Help
- Big Data Mining Assignment Help
- Parallel Computing And Distributed Computing
- Law And Computer Science Assignment Help
- Engineering Distributed Objects For Cloud Computing
- Building Secure Computer Systems Assignment Help
- Ada Assignment Help
- R Programming Assignment Help
- Oracle Online Assignment Help
- Languages And Automata Assignment Help
- Haskell Assignment Help
- Economics And Computation Assignment Help
- ActionScript Assignment Help
- Audio Programming Assignment Help
- Bash Assignment Help
- Computer Graphics Assignment Help
- Groovy Assignment Help
- Kotlin Assignment Help
- Object Oriented Languages And Environments
- COBOL ASSIGNMENT HELP
- Bayesian Statistical Probabilistic Programming
- Computer Network Assignment Help
- Django Assignment Help
- Lambda Calculus Assignment Help
- Operating System Assignment Help
- Computational Learning Theory
- Delphi Assignment Help
- Concurrent Algorithms And Data Structures Assignment Help
- Machine Learning Assignment Help
- Human Computer Interface Assignment Help
- Foundations Of Data Networking Assignment Help
- Continuous Mathematics Assignment Help
- Compiler Assignment Help
- Computational Biology Assignment Help
- PostgreSQL Online Assignment Help
- Lua Assignment Help
- Human Computer Interaction Assignment Help
- Ethics And Responsible Innovation Assignment Help
- Communication And Ethical Issues In Computing
- Computer Science
- Combinatorial Optimisation Assignment Help
- Ethical Computing In Practice
- HTML Homework Assignment Help
- Linear Algebra Assignment Help
- Perl Assignment Help
- Artificial Intelligence Assignment Help
- Uncategorized
- Ethics And Professionalism Assignment Help
- Human Augmentics Assignment Help
- Linux Assignment Help
- PHP Assignment Help
- Assembly Language Assignment Help
- Dart Assignment Help
- Complete Python Bootcamp From Zero To Hero In Python Corrected Version
- Swift Assignment Help
- Computational Complexity Assignment Help
- Probability And Computing Assignment Help
- MATLAB Programming For Engineers
- Introduction To Statistical Learning
- Database Systems Implementation Assignment Help
- Computational Game Theory Assignment Help
- Database Assignment Help
- Probabilistic Model Checking Assignment Help
- Mathematics For Computer Science And Philosophy
- Introduction To Formal Proof Assignment Help
- Creative Coding Assignment Help
- Foundations Of Self-Programming Agents Assignment Help
- Machine Organization Assignment Help
- Software Design Assignment Help
- Data Communication And Networking Assignment Help
- Computational Biology
- Data Structure Assignment Help
- Foundations Of Software Engineering Assignment Help
- Mathematical Foundations Of Computing
- Principles Of Programming Languages Assignment Help
- Software Engineering Capstone Assignment Help
- Algorithms and Data Structures Assignment Help
No Comments