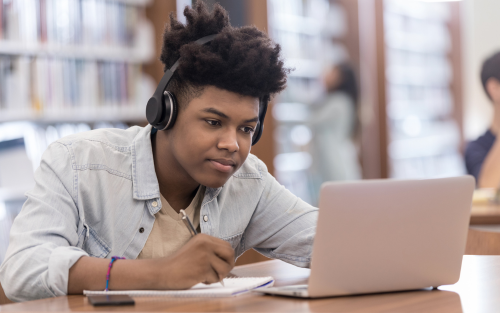
02 May Building A Progressive Web App With Angular
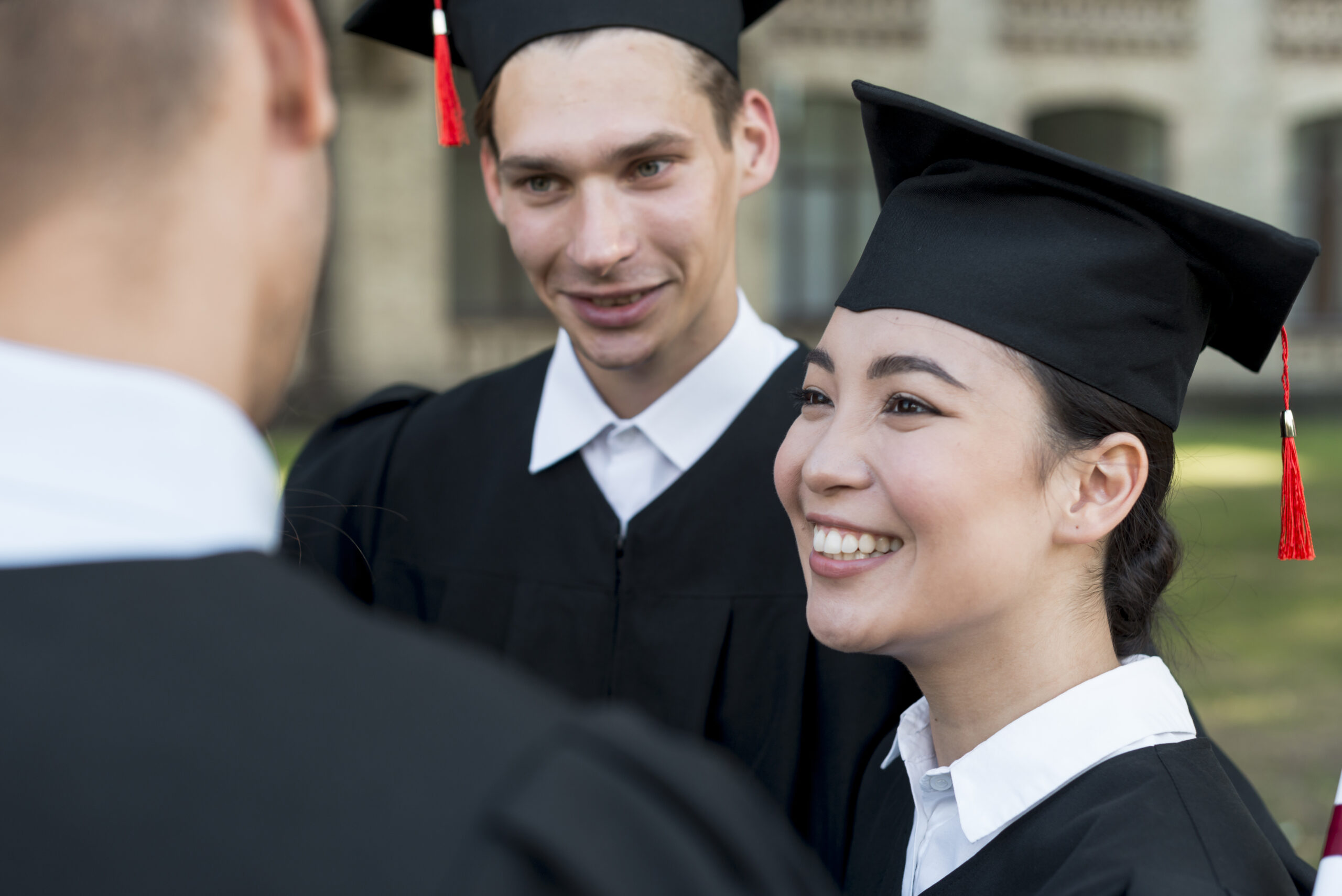
Introduction
Progressive Web Apps (PWAs) are web applications that offer the features of native apps while still being accessible through a web browser. They provide a reliable, fast, and engaging user experience on any device and platform, with or without an internet connection. Angular, one of the most popular web development frameworks, provides a powerful platform for building PWAs. In this article, we will explore how to build a simple PWA with Angular.
Discover how to build a progressive web app (PWA) using Angular. In this tutorial, you’ll learn how to leverage the power of Angular, a popular JavaScript framework, to create modern web applications that provide an app-like experience across different devices and platforms. Explore the key principles of progressive web apps, understand the fundamentals of Angular, and build a robust and responsive web app that can be installed on users’ devices and work offline.
Building a progressive web app with Angular allows you to combine the best of web and mobile app experiences. Progressive web apps leverage modern web technologies to deliver fast, reliable, and engaging user experiences. Angular, with its component-based architecture, provides a solid foundation for building scalable and responsive web applications.
This tutorial will guide you through the process of building a progressive web app using Angular. You’ll learn how to set up a new Angular project using the Angular CLI, create reusable components, and organize your code using Angular modules. Angular’s powerful routing capabilities will enable you to create navigation flows within your app.
To transform your Angular web app into a progressive web app, you’ll explore the concepts of service workers and the web app manifest. Service workers enable offline functionality and background synchronization, allowing your app to work seamlessly even without an internet connection. The web app manifest allows users to install your app on their devices, giving them quick access to your app from the home screen.
Throughout the tutorial, you’ll also learn about Angular development tools, performance optimization techniques, and best practices for building high-quality progressive web apps. Angular Material, a UI component library for Angular, can be utilized to enhance the visual appeal and usability of your app.
By the end of the tutorial, you’ll have a fully functional progressive web app built with Angular that delivers a smooth and engaging user experience across various devices and platforms.
Building a progressive web app with Angular opens up opportunities to reach a wider audience and deliver exceptional user experiences. Whether you’re a beginner or an experienced Angular developer, this tutorial will equip you with the knowledge and skills to create modern web apps that blur the line between web and native applications.
What is a Progressive Web App?
A Progressive Web App is a web application that offers a native-like user experience by leveraging modern web technologies and best practices. PWAs can be installed on a user’s device and accessed from the home screen, just like a native app. They offer features such as offline access, push notifications, and the ability to work across multiple devices and platforms.
Why Build a PWA with Angular?
Angular is a popular web development framework that offers a robust set of features for building PWAs. It provides a powerful toolset for building fast and responsive applications that work across different devices and platforms. Some of the key features of Angular that make it an ideal choice for building PWAs include:
Service Workers: Angular provides built-in support for Service Workers, a key technology for building PWAs. Service Workers enable offline access, push notifications, and background syncing, making PWAs more reliable and engaging.
PWA Features: Angular provides built-in support for PWA features such as Add to Home Screen, which allows users to install the app on their device’s home screen, and Web App Manifest, which provides metadata about the app.
Angular Universal: Angular Universal is a server-side rendering solution that allows PWAs to be rendered on the server, improving performance and SEO.
Building a Simple PWA with Angular
To build a simple PWA with Angular, we will create a weather app that displays the current weather for a given location. We will use the OpenWeatherMap API to fetch weather data and display it in our app. Here are the steps to build the app:
Step 1: Set up an Angular Project
First, we need to set up an Angular project. We can use the Angular CLI to create a new project with the following command:
ng new angular-pwa-weather
This command will create a new Angular project with the name “angular-pwa-weather”. Once the project is created, we can navigate to the project directory and run it with the following command:
cd angular-pwa-weather
ng serve
Step 2: Add a Service Worker
To add Service Worker support to our app, we can use the built-in Angular Service Worker module. We can add it to our app with the following command:
ng add @angular/pwa
This command will install the necessary dependencies and add the Service Worker configuration to our app.
-First, create a new file called weather.service.ts
inside the src/app
folder.
-Next, import the necessary modules and services from Angular and RxJS:
import { Injectable } from '@angular/core';
import { HttpClient } from '@angular/common/http';
import { Observable } from 'rxjs/Observable';
import { catchError, map } from 'rxjs/operators';
import 'rxjs/add/observable/throw';
-Use the @Injectable
decorator to declare the WeatherService
class as a service that can be injected into other components:
@Injectable()
export class WeatherService {
constructor(private http: HttpClient) {}
}
-Define a function that will send a GET request to the OpenWeatherMap API to fetch weather data for a particular city. The function should take the name of the city as an argument and return an Observable that emits the weather data:
getWeather(city: string): Observable<any> {
const url = `https://api.openweathermap.org/data/2.5/weather?q=${city}&appid=${API_KEY}`;
return this.http
.get(url)
.pipe(
map(response => response),
catchError(error => Observable.throw(error))
);
}
-In the getWeather()
function, we are using the Angular HttpClient
service to make an HTTP GET request to the OpenWeatherMap API. We are also using the RxJS map()
and catchError()
operators to transform the response and handle any errors that might occur.
-Finally, we need to define the API_KEY
constant at the top of the file:
const API_KEY = 'YOUR_API_KEY';
Note that you need to replace YOUR_API_KEY
with your own API key from OpenWeatherMap.
With the WeatherService
created, we can now use it in our weather.component.ts
file to fetch weather data for a particular city.
FAQs: Building A Progressive Web App With Angular
What is a Progressive Web App (PWA)?
A Progressive Web App (PWA) is a web application that combines the best features of web and mobile applications, providing users with a native app-like experience. PWAs are built using web technologies (HTML, CSS, and JavaScript) but offer features such as offline functionality, push notifications, and device hardware access.
What is Angular?
Angular is a popular open-source JavaScript framework developed and maintained by Google. It is used for building web applications, including PWAs. Angular provides a robust and scalable framework for creating dynamic, single-page applications with a modular architecture, data binding, and a powerful set of features and tools.
What are the benefits of building a PWA with Angular?
Building a PWA with Angular offers several advantages, including:
- Cross-platform compatibility: PWAs built with Angular can run on various platforms, including desktop and mobile devices, without the need for platform-specific code.
- Offline functionality: Angular Service Workers allow PWAs to work offline or in low-connectivity scenarios, caching app resources and providing a seamless user experience.
- Improved performance: Angular’s performance optimization features, such as lazy loading and Ahead-of-Time (AOT) compilation, help create fast and responsive PWAs.
- Native-like experience: PWAs built with Angular can leverage features like push notifications, home screen installation, and device hardware access, providing a native app-like experience to users.
- Easy maintenance: Angular’s modular architecture, component-based approach, and extensive tooling make it easier to maintain and update PWAs over time.
- SEO-friendly: Angular supports server-side rendering (SSR), which helps improve search engine optimization (SEO) and allows PWAs to be more discoverable by search engines.
What are the basic steps to build a PWA with Angular?
The basic steps to build a PWA with Angular include:
- Set up an Angular development environment.
- Create a new Angular project using the Angular CLI.
- Design and develop the user interface of your PWA using Angular components and templates.
- Implement offline functionality using Angular Service Workers.
- Optimize performance by applying Angular best practices, such as lazy loading, AOT compilation, and code minification.
- Test your PWA across different devices and browsers to ensure compatibility.
- Deploy your PWA to a web server or a hosting platform.
- Register your PWA with appropriate app manifests and configure service worker caching strategies.
- Continuously monitor and update your PWA based on user feedback and changing requirements.
Can I reuse code from an existing Angular application when building a PWA?
Yes, if you already have an Angular application, you can reuse a significant portion of the code when building a PWA. Angular’s component-based architecture and code modularity make it easier to extract and reuse components, services, and other resources in the PWA development process.
Can I publish my Angular PWA to app stores?
While PWAs are primarily web-based applications, some app stores, such as the Microsoft Store, allow PWAs to be published as “store apps.” This enables users to discover and install your PWA from the app store. However, it’s important to note that not all app stores support PWAs, and the process and requirements may vary between platforms.
Are there any limitations to consider when building a PWA with Angular?
Some limitations to consider when building a PWA with Angular include:
- Limited access to certain device APIs: While PWAs can access many device features, there may be some limitations compared to native apps. Not all device APIs may be available or have the same level of functionality.
- Browser support: Although most modern browsers support PWAs, some older browsers may have limited or no support for certain PWA features. It’s important to test and ensure compatibility across a range of browsers.
- Storage limitations: PWAs typically have limited storage compared to native apps. Local storage options like IndexedDB and Web Storage should be used judiciously to manage data and resources efficiently.
Are there resources available to learn more about building PWAs with Angular?
Yes, there are numerous resources available to learn more about building PWAs with Angular. The official Angular documentation provides detailed guides and tutorials on building PWAs. Additionally, there are online courses, blogs, and community forums where developers share their experiences, best practices, and code samples related to building PWAs with Angular.
Can I integrate third-party libraries or frameworks into my Angular PWA?
Yes, Angular supports the integration of third-party libraries and frameworks. Many popular JavaScript libraries and frameworks can be easily integrated into Angular applications. This allows you to leverage additional functionality and features provided by those libraries when building your Angular PWA.
Can I deploy my Angular PWA to different hosting platforms?
Yes, you can deploy your Angular PWA to various hosting platforms, including cloud-based services like Amazon Web Services (AWS), Microsoft Azure, or Google Cloud Platform. Additionally, you can deploy your PWA to traditional web hosting providers or your own servers. The deployment process typically involves building and packaging your Angular app and then uploading it to the hosting platform of your choice.
Conclusion
Latest Topic
-
Cloud-Native Technologies: Best Practices
20 April, 2024 -
Generative AI with Llama 3: Shaping the Future
15 April, 2024 -
Mastering Llama 3: The Ultimate Guide
10 April, 2024
Category
- Assignment Help
- Homework Help
- Programming
- Trending Topics
- C Programming Assignment Help
- Art, Interactive, And Robotics
- Networked Operating Systems Programming
- Knowledge Representation & Reasoning Assignment Help
- Digital Systems Assignment Help
- Computer Design Assignment Help
- Artificial Life And Digital Evolution
- Coding and Fundamentals: Working With Collections
- UML Online Assignment Help
- Prolog Online Assignment Help
- Natural Language Processing Assignment Help
- Julia Assignment Help
- Golang Assignment Help
- Design Implementation Of Network Protocols
- Computer Architecture Assignment Help
- Object-Oriented Languages And Environments
- Coding Early Object and Algorithms: Java Coding Fundamentals
- Deep Learning In Healthcare Assignment Help
- Geometric Deep Learning Assignment Help
- Models Of Computation Assignment Help
- Systems Performance And Concurrent Computing
- Advanced Security Assignment Help
- Typescript Assignment Help
- Computational Media Assignment Help
- Design And Analysis Of Algorithms
- Geometric Modelling Assignment Help
- JavaScript Assignment Help
- MySQL Online Assignment Help
- Programming Practicum Assignment Help
- Public Policy, Legal, And Ethical Issues In Computing, Privacy, And Security
- Computer Vision
- Advanced Complexity Theory Assignment Help
- Big Data Mining Assignment Help
- Parallel Computing And Distributed Computing
- Law And Computer Science Assignment Help
- Engineering Distributed Objects For Cloud Computing
- Building Secure Computer Systems Assignment Help
- Ada Assignment Help
- R Programming Assignment Help
- Oracle Online Assignment Help
- Languages And Automata Assignment Help
- Haskell Assignment Help
- Economics And Computation Assignment Help
- ActionScript Assignment Help
- Audio Programming Assignment Help
- Bash Assignment Help
- Computer Graphics Assignment Help
- Groovy Assignment Help
- Kotlin Assignment Help
- Object Oriented Languages And Environments
- COBOL ASSIGNMENT HELP
- Bayesian Statistical Probabilistic Programming
- Computer Network Assignment Help
- Django Assignment Help
- Lambda Calculus Assignment Help
- Operating System Assignment Help
- Computational Learning Theory
- Delphi Assignment Help
- Concurrent Algorithms And Data Structures Assignment Help
- Machine Learning Assignment Help
- Human Computer Interface Assignment Help
- Foundations Of Data Networking Assignment Help
- Continuous Mathematics Assignment Help
- Compiler Assignment Help
- Computational Biology Assignment Help
- PostgreSQL Online Assignment Help
- Lua Assignment Help
- Human Computer Interaction Assignment Help
- Ethics And Responsible Innovation Assignment Help
- Communication And Ethical Issues In Computing
- Computer Science
- Combinatorial Optimisation Assignment Help
- Ethical Computing In Practice
- HTML Homework Assignment Help
- Linear Algebra Assignment Help
- Perl Assignment Help
- Artificial Intelligence Assignment Help
- Uncategorized
- Ethics And Professionalism Assignment Help
- Human Augmentics Assignment Help
- Linux Assignment Help
- PHP Assignment Help
- Assembly Language Assignment Help
- Dart Assignment Help
- Complete Python Bootcamp From Zero To Hero In Python Corrected Version
- Swift Assignment Help
- Computational Complexity Assignment Help
- Probability And Computing Assignment Help
- MATLAB Programming For Engineers
- Introduction To Statistical Learning
- Database Systems Implementation Assignment Help
- Computational Game Theory Assignment Help
- Database Assignment Help
- Probabilistic Model Checking Assignment Help
- Mathematics For Computer Science And Philosophy
- Introduction To Formal Proof Assignment Help
- Creative Coding Assignment Help
- Foundations Of Self-Programming Agents Assignment Help
- Machine Organization Assignment Help
- Software Design Assignment Help
- Data Communication And Networking Assignment Help
- Computational Biology
- Data Structure Assignment Help
- Foundations Of Software Engineering Assignment Help
- Mathematical Foundations Of Computing
- Principles Of Programming Languages Assignment Help
- Software Engineering Capstone Assignment Help
- Algorithms and Data Structures Assignment Help
No Comments