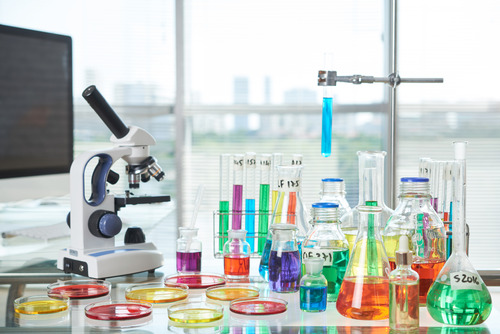
01 May Building A Progressive Web App With Polymer
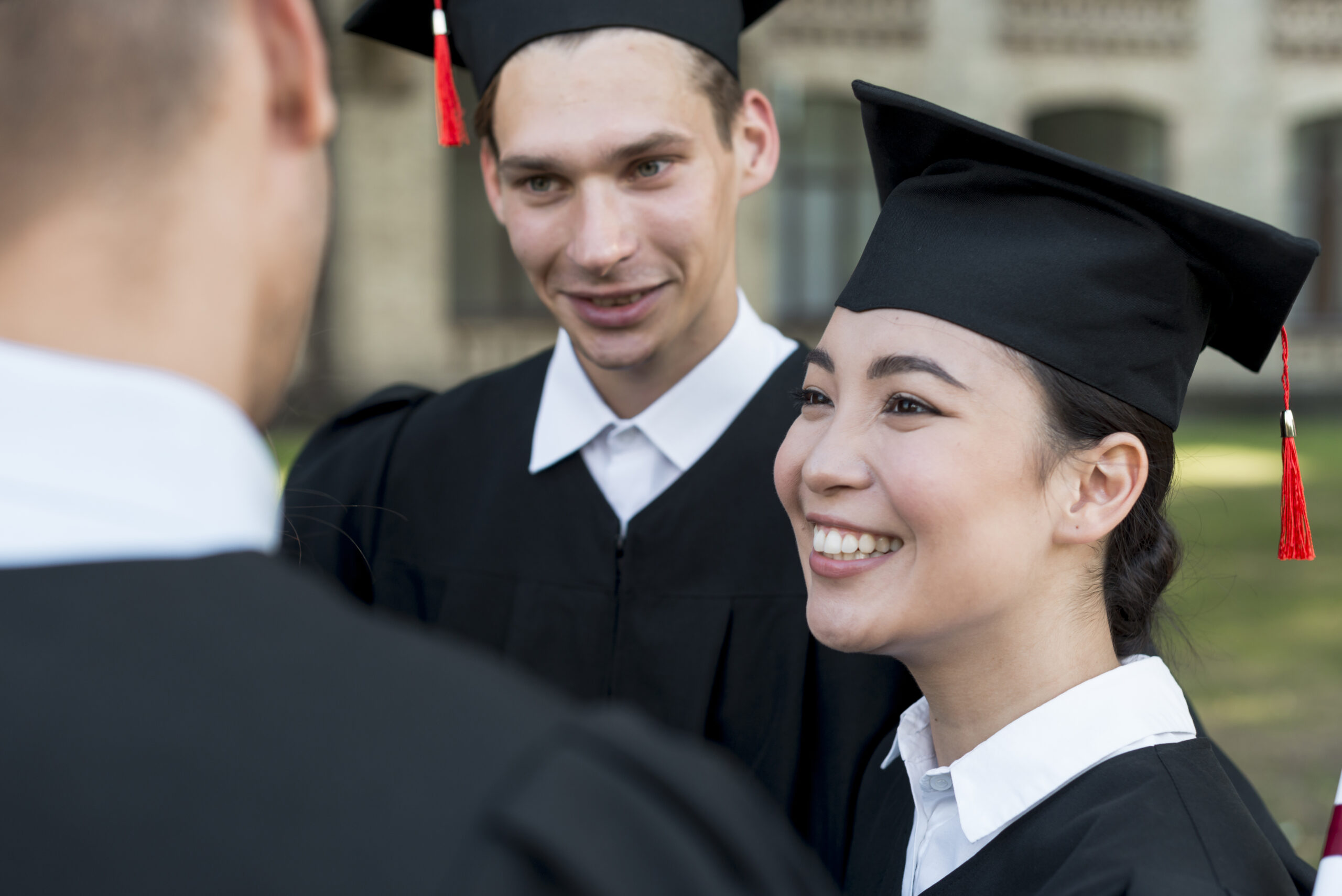
Introduction
As the world becomes increasingly mobile, web applications are more important than ever. Progressive Web Apps (PWAs) have emerged as an excellent way to build fast, reliable, and engaging web applications that offer a native-like experience on mobile devices. In this article, we will explore how to build a PWA with Polymer.
What is Polymer?
Polymer is a JavaScript library for building web applications using Web Components. Web Components allow developers to create reusable, encapsulated, and customizable HTML elements that can be used across different web applications. Polymer provides a set of tools and libraries for building and working with Web Components.
Why Use Polymer for Building a PWA?
Polymer is an excellent choice for building PWAs because it offers several features that are essential for creating high-quality web applications. These features include:
Custom Elements: Custom elements are a key feature of Web Components. Polymer provides a set of tools for creating and working with custom elements. Custom elements allow developers to create reusable and encapsulated components that can be used across different applications.
Shadow DOM: Shadow DOM is a crucial part of Web Components. Shadow DOM allows developers to encapsulate styles, scripts, and markup within a custom element, preventing them from affecting the rest of the web page.
Service Workers: Service workers are a core feature of PWAs. Service workers allow developers to cache resources, handle network requests, and provide offline support. Polymer provides a set of tools and libraries for working with service workers.
Templates: Templates are a key feature of Web Components. Polymer provides a set of tools for creating and working with templates. Templates allow developers to create reusable and customizable markup that can be used across different applications.
Now that we have understood why Polymer is an excellent choice for building PWAs, let’s dive into building a PWA with Polymer.
Step 1: Set Up Your Development Environment Before we can start building our PWA, we need to set up our development environment. We will need the following tools:
Node.js: Node.js is a JavaScript runtime that allows us to run JavaScript code outside of a web browser.
Polymer CLI: Polymer CLI is a command-line interface for building and working with Polymer projects.
A text editor: We will need a text editor for writing our code. There are many options available, including Visual Studio Code, Atom, and Sublime Text.
Step 2: Create a New Polymer Project Once we have set up our development environment, we can create a new Polymer project using the Polymer CLI. To create a new project, run the following command in your terminal:
polymer init
The Polymer CLI will then prompt you to choose a project template. For this tutorial, we will choose the “polymer-3-application” template.
Which starter template would you like to use?
(Use arrow keys)
❯ polymer-3-application - A starting template for building a Polymer 3 application
polymer-2-application - A starting template for building a Polymer 2 application
polymer-1-starter-kit - A starter kit for building a Polymer 1.x application
After selecting the “polymer-3-application” template, the Polymer CLI will prompt you to enter a name for your project. Enter a name and press enter to continue.
The Polymer CLI will then create a new directory for your project and generate the necessary files and folders.
Step 3: Build Your PWA Now that we have created a new Polymer project, we can start building our PWA. The first thing we need to do is to create the basic structure of our application. We can do this by creating a new element using the Polymer CLI. To create a new element, run the following command in your terminal:
polymer generate element my-element
This command will create a new element called “my-element” in the “src” directory of our project. We can then customize this element to suit our needs.
Next, we need to create a “manifest.json” file and a service worker file. The manifest file is a JSON file that contains metadata about our application, such as its name, icon, and theme color. The service worker is a JavaScript file that handles network requests, caching, and offline support.
To create the manifest file, create a new file called “manifest.json” in the root directory of your project and add the following code:
{
"name": "My PWA",
"short_name": "My PWA",
"icons": [{
"src": "images/icons/icon-192x192.png",
"sizes": "192x192",
"type": "image/png"
}, {
"src": "images/icons/icon-512x512.png",
"sizes": "512x512",
"type": "image/png"
}],
"start_url": "/",
"display": "standalone",
"background_color": "#ffffff",
"theme_color": "#2196f3"
}
This code defines the name of our application, its icon, and its theme color.
Next, we need to create a service worker file. To create a service worker, create a new file called “sw.js” in the root directory of your project and add the following code:
self.addEventListener('install', function(event) {
event.waitUntil(
caches.open('my-pwa-cache').then(function(cache) {
return cache.addAll([
'/',
'/index.html',
'/src/my-element/my-element.js',
'/src/my-element/my-element.html',
'/images/icons/icon-192x192.png',
'/images/icons/icon-512x512.png'
]);
})
);
});
self.addEventListener('fetch', function(event) {
event.respondWith(
caches.match(event.request).then(function(response) {
return response || fetch(event.request);
})
);
});
This code defines two event listeners: one for the “install” event, and one for the “fetch” event. The “install” event listener adds resources to the cache, and the “fetch” event listener retrieves resources from the cache.
Now that we have created our manifest and service worker files, we need to register our service worker in our application. To register our service worker, add the following code to your “index.html” file:
<script>
if ('serviceWorker' in navigator) {
window.addEventListener('load', function() {
navigator.serviceWorker.register('/sw.js');
});
}
</script>
This code checks if the user’s browser supports service workers and registers our service worker if it does.
Step 4: Add Offline Support. To add offline support to our PWA, we can modify our service worker to cache the resources that our application needs to function offline. We can do this by updating the “install” event listener in our service worker file to add the necessary resources to the cache.
self.addEventListener('install', function(event) {
event.waitUntil(
caches.open('my-pwa-cache').then(function(cache) {
return cache.addAll([
'/',
'/index.html',
'/src/my-element/my-element.js',
'/src/my-element/my-element.html',
'/images/icons/icon-192x192.png',
'/images/icons/icon-512x512.png'
]);
})
);
});
In this example, we are adding our index.html file, our my-element.js and my-element.html files, and our application icons to the cache. You can add any other resources that your application needs to function offline to the cache.
Once we have added the necessary resources to the cache, we need to modify our “fetch” event listener in our service worker to retrieve resources from the cache if they are available.
self.addEventListener('fetch', function(event) {
event.respondWith(
caches.match(event.request).then(function(response) {
return response || fetch(event.request);
})
);
});
In this example, we are using the “caches.match()” method to check if the requested resource is available in the cache. If it is, we return the cached resource. If it’s not, we retrieve the resource from the network.
Step 5: Test Your PWA Now that we have built our PWA and added offline support, we need to test it to ensure that it is functioning as expected. To test your PWA, you can use a tool like Lighthouse, which is built into the Google Chrome Developer Tools.
To run a Lighthouse audit on your PWA, open your PWA in Google Chrome and open the Developer Tools by pressing “Ctrl+Shift+I” or “Cmd+Shift+I” on a Mac. In the Developer Tools, click on the “Lighthouse” tab and select “Progressive Web App” as the category to audit. Then click on the “Generate report” button to run the audit.
The Lighthouse audit will give you a report on the performance, accessibility, best practices, and SEO of your PWA. It will also give you a score out of 100 for your PWA’s progressive web app capabilities.
Conclusion
Building a progressive web app with Polymer is a great way to create a modern, responsive, and scalable web application. By following the steps outlined in this guide, you can create a simple but effective PWA that works offline and provides a great user experience.
Remember to focus on performance, accessibility, and user experience when building your PWA, and test your PWA using tools like Lighthouse to ensure that it is functioning as expected. With the right tools and techniques, you can create a successful and scalable progressive web app with Polymer.
Latest Topic
-
Cloud-Native Technologies: Best Practices
20 April, 2024 -
Generative AI with Llama 3: Shaping the Future
15 April, 2024 -
Mastering Llama 3: The Ultimate Guide
10 April, 2024
Category
- Assignment Help
- Homework Help
- Programming
- Trending Topics
- C Programming Assignment Help
- Art, Interactive, And Robotics
- Networked Operating Systems Programming
- Knowledge Representation & Reasoning Assignment Help
- Digital Systems Assignment Help
- Computer Design Assignment Help
- Artificial Life And Digital Evolution
- Coding and Fundamentals: Working With Collections
- UML Online Assignment Help
- Prolog Online Assignment Help
- Natural Language Processing Assignment Help
- Julia Assignment Help
- Golang Assignment Help
- Design Implementation Of Network Protocols
- Computer Architecture Assignment Help
- Object-Oriented Languages And Environments
- Coding Early Object and Algorithms: Java Coding Fundamentals
- Deep Learning In Healthcare Assignment Help
- Geometric Deep Learning Assignment Help
- Models Of Computation Assignment Help
- Systems Performance And Concurrent Computing
- Advanced Security Assignment Help
- Typescript Assignment Help
- Computational Media Assignment Help
- Design And Analysis Of Algorithms
- Geometric Modelling Assignment Help
- JavaScript Assignment Help
- MySQL Online Assignment Help
- Programming Practicum Assignment Help
- Public Policy, Legal, And Ethical Issues In Computing, Privacy, And Security
- Computer Vision
- Advanced Complexity Theory Assignment Help
- Big Data Mining Assignment Help
- Parallel Computing And Distributed Computing
- Law And Computer Science Assignment Help
- Engineering Distributed Objects For Cloud Computing
- Building Secure Computer Systems Assignment Help
- Ada Assignment Help
- R Programming Assignment Help
- Oracle Online Assignment Help
- Languages And Automata Assignment Help
- Haskell Assignment Help
- Economics And Computation Assignment Help
- ActionScript Assignment Help
- Audio Programming Assignment Help
- Bash Assignment Help
- Computer Graphics Assignment Help
- Groovy Assignment Help
- Kotlin Assignment Help
- Object Oriented Languages And Environments
- COBOL ASSIGNMENT HELP
- Bayesian Statistical Probabilistic Programming
- Computer Network Assignment Help
- Django Assignment Help
- Lambda Calculus Assignment Help
- Operating System Assignment Help
- Computational Learning Theory
- Delphi Assignment Help
- Concurrent Algorithms And Data Structures Assignment Help
- Machine Learning Assignment Help
- Human Computer Interface Assignment Help
- Foundations Of Data Networking Assignment Help
- Continuous Mathematics Assignment Help
- Compiler Assignment Help
- Computational Biology Assignment Help
- PostgreSQL Online Assignment Help
- Lua Assignment Help
- Human Computer Interaction Assignment Help
- Ethics And Responsible Innovation Assignment Help
- Communication And Ethical Issues In Computing
- Computer Science
- Combinatorial Optimisation Assignment Help
- Ethical Computing In Practice
- HTML Homework Assignment Help
- Linear Algebra Assignment Help
- Perl Assignment Help
- Artificial Intelligence Assignment Help
- Uncategorized
- Ethics And Professionalism Assignment Help
- Human Augmentics Assignment Help
- Linux Assignment Help
- PHP Assignment Help
- Assembly Language Assignment Help
- Dart Assignment Help
- Complete Python Bootcamp From Zero To Hero In Python Corrected Version
- Swift Assignment Help
- Computational Complexity Assignment Help
- Probability And Computing Assignment Help
- MATLAB Programming For Engineers
- Introduction To Statistical Learning
- Database Systems Implementation Assignment Help
- Computational Game Theory Assignment Help
- Database Assignment Help
- Probabilistic Model Checking Assignment Help
- Mathematics For Computer Science And Philosophy
- Introduction To Formal Proof Assignment Help
- Creative Coding Assignment Help
- Foundations Of Self-Programming Agents Assignment Help
- Machine Organization Assignment Help
- Software Design Assignment Help
- Data Communication And Networking Assignment Help
- Computational Biology
- Data Structure Assignment Help
- Foundations Of Software Engineering Assignment Help
- Mathematical Foundations Of Computing
- Principles Of Programming Languages Assignment Help
- Software Engineering Capstone Assignment Help
- Algorithms and Data Structures Assignment Help
No Comments