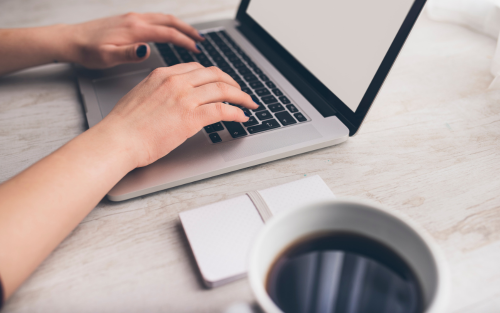
01 May Building A Simple Chatbot With Microsoft Bot Framework
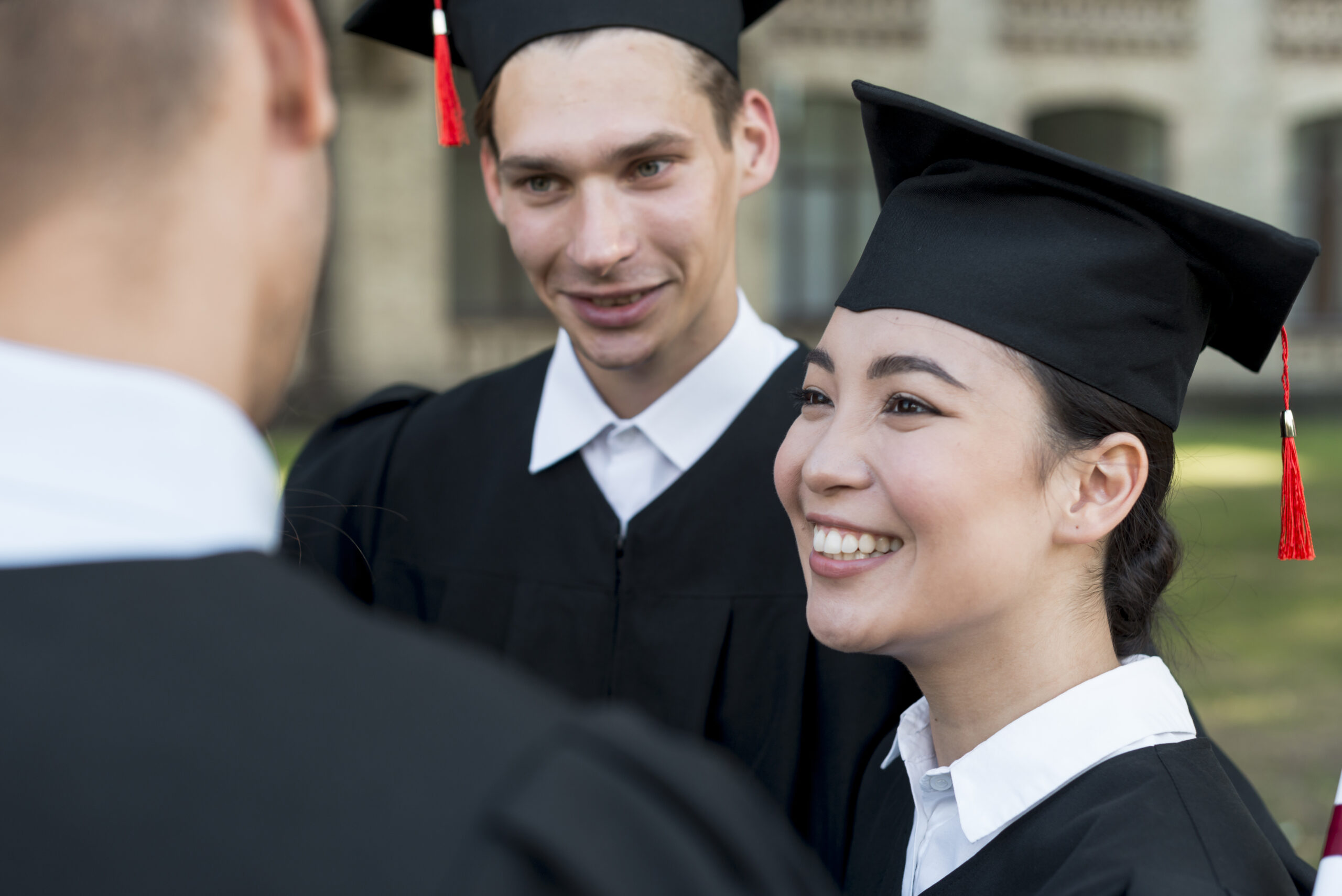
Introduction
Chatbots are becoming increasingly popular for customer service and communication purposes. They provide an easy way for businesses to interact with their customers, answering their questions and providing support. Microsoft Bot Framework is a popular tool for building chatbots that can be integrated with various messaging platforms. In this article, we will discuss how to build a simple chatbot with Microsoft Bot Framework.
Learn how to build a simple chatbot using the Microsoft Bot Framework. This step-by-step tutorial guides you through the process of creating a chatbot that can interact with users, provide automated responses, and perform basic tasks. Discover how to set up the development environment, define the bot’s behavior using dialogues, integrate natural language understanding, and deploy the chatbot to various messaging platforms. By the end of this tutorial, you’ll have a functional chatbot that can engage with users and provide valuable automated assistance.
Building a simple chatbot with the Microsoft Bot Framework enables you to create interactive conversational interfaces that can engage with users and provide automated assistance. In this tutorial, you’ll learn how to set up the development environment, define the bot’s behavior, integrate natural language understanding, and deploy the chatbot to various messaging platforms.
You’ll start by installing the necessary tools and libraries for chatbot development using the Microsoft Bot Framework SDK. Then, you’ll define the bot’s behavior using dialogues, which manage the conversation flow and handle user interactions.
Integrating natural language understanding allows your chatbot to understand user intents and extract entities from their messages. You’ll explore techniques for training and configuring the language understanding models to make your chatbot more effective in understanding user inputs.
Throughout the tutorial, you’ll learn best practices for chatbot development, including testing and debugging techniques, deployment strategies, and considerations for maintaining and updating your chatbot.
Additionally, you’ll discover how to integrate your chatbot with external services and APIs to extend its capabilities and provide more valuable responses to user queries.
By the end of this tutorial, you’ll have a functional chatbot that can engage with users, respond to their queries, and perform basic tasks. Whether you’re building a customer support bot, a virtual assistant, or an interactive information provider, the Microsoft Bot Framework provides a robust platform to develop and deploy chatbots across various messaging platforms.
Building a chatbot with the Microsoft Bot Framework opens up endless possibilities for creating intelligent and interactive conversational experiences. Start building your chatbot today and deliver automated assistance to your users.
What is Microsoft Bot Framework?
Microsoft Bot Framework is a set of tools and services for building chatbots that can be integrated with various messaging platforms, such as Skype, Facebook Messenger, and Slack. It includes a Bot Builder SDK for developing custom bots and a Bot Connector service for connecting bots to messaging platforms.
The Bot Builder SDK is a framework for building bots using C# or Node.js. It provides a set of libraries and tools for building, testing, and deploying bots. The Bot Connector service is a REST API for connecting bots to messaging platforms. It handles the communication between the bot and the messaging platform, allowing the bot to receive and respond to messages.
Building a Simple Chatbot with Microsoft Bot Framework
To build a simple chatbot with Microsoft Bot Framework, you will need to follow the steps below:
Step 1: Create a New Bot Project: To create a new bot project, you will need to download and install the Bot Builder SDK for your preferred programming language, C# or Node.js. Once you have installed the SDK, you can create a new bot project using the Bot Builder CLI (Command Line Interface).
For example, to create a new bot project using the Bot Builder CLI for Node.js, you can run the following command:
botbuilder init --name mybot --botType chat --noPrompt
This will create a new bot project named “mybot” with a chatbot template.
Step 2: Define the Bot’s Dialog: The dialog is the core component of a chatbot. It defines the conversation flow between the bot and the user. To define the bot’s dialog, you will need to create a new dialog class in your bot project and implement the dialog logic.
For example, to create a new dialog class for a simple chatbot that greets the user and asks for their name, you can define the dialog as follows:
const { ComponentDialog, TextPrompt } = require('botbuilder-dialogs');
class GreetingDialog extends ComponentDialog {
constructor(dialogId) {
super(dialogId);
this.addDialog(new TextPrompt(‘namePrompt’));
this.addDialog(
new WaterfallDialog(‘greetingDialog’, [
async (step) => {
await step.context.sendActivity(‘Hi there! What is your name?’);
return await step.prompt(‘namePrompt’, { prompt: ” });
},
async (step) => {
const name = step.result;
await step.context.sendActivity(`Nice to meet you, ${name}!`);
return await step.endDialog();
},
])
);
this.initialDialogId = ‘greetingDialog’;
}
}
module.exports.GreetingDialog = GreetingDialog;
This defines a new dialog class named “GreetingDialog” that prompts the user for their name and greets them.
Step 3: Integrate the Bot with a Messaging Platform: To integrate the bot with a messaging platform, you will need to create a new bot connector and register it with the messaging platform. The Bot Connector service provides a REST API for connecting bots to messaging platforms.
For example, to connect the bot to Facebook Messenger, you can create a new bot connector and register it with Facebook Messenger as follows:
const { BotFrameworkAdapter } = require('botbuilder');
const { GreetingDialog } = require('./dialogs/greetingDialog');
const adapter = new BotFrameworkAdapter({
appId: process.env.MicrosoftAppId,
appPassword: process.env.MicrosoftAppPassword
});
const dialog = new GreetingDialog(‘greetingDialog’);
adapter.onTurn(async (context) => {
await dialog.run(context);
});
const express = require(‘express’);
const app = express();
app.post(‘/api/messages’, (req, res) => {
adapter.processActivity(req, res, async (context) => {
await adapter.run(context);
});
});
app.listen(process.env.PORT || 3978, () => {
console.log(`Server running on port ${process.env.PORT || 3978}`);
});
This code creates a new bot connector using the BotFrameworkAdapter class and sets the bot’s App ID and App Password. It then creates a new instance of the GreetingDialog class and registers it as the dialog for the bot.
The adapter’s onTurn method is used to handle incoming messages from the messaging platform. It passes the incoming message to the dialog’s run method to process the message.
Finally, the code creates a new Express app and sets up a route for handling incoming messages from Facebook Messenger. The adapter’s processActivity method is used to process incoming messages from Facebook Messenger.
FAQs
What is Microsoft Bot Framework?
Microsoft Bot Framework is a comprehensive platform that enables developers to build and deploy chatbots across multiple channels, including websites, messaging platforms, and voice assistants. It provides a set of tools, SDKs, and services to streamline the development and management of chatbots.
Why should I use Microsoft Bot Framework to build a chatbot?
Microsoft Bot Framework offers several advantages for building chatbots, including:
- Cross-channel support: With Microsoft Bot Framework, you can build chatbots that work seamlessly across various channels, including Microsoft Teams, Slack, Facebook Messenger, and more.
- Rich features and functionality: The framework provides a wide range of features, including natural language understanding, sentiment analysis, dialog management, and integration with Microsoft Azure services.
- Bot intelligence and machine learning: Microsoft Bot Framework integrates with Azure Cognitive Services, allowing you to incorporate machine learning capabilities, such as language understanding and computer vision, into your chatbot.
- Developer-friendly tools and SDKs: The framework provides developer-friendly tools, such as the Bot Builder SDK and the Bot Framework Emulator, to simplify the bot development process and enable local testing and debugging.
- Integration with Microsoft ecosystem: If you’re already using Microsoft technologies or services, the Bot Framework seamlessly integrates with Azure services, Microsoft Teams, and other Microsoft products, making it easier to leverage existing infrastructure and resources.
What are the basic steps involved in building a simple chatbot with Microsoft Bot Framework?
The basic steps for building a chatbot with Microsoft Bot Framework include:
- Setting up a development environment with the necessary tools and SDKs.
- Defining the conversational flow and intents of the chatbot.
- Implementing natural language understanding using services like Azure Cognitive Services or LUIS (Language Understanding Intelligent Service).
- Designing and developing the chatbot’s dialogs and responses using the Bot Builder SDK.
- Testing the chatbot locally using the Bot Framework Emulator.
- Deploying the chatbot to a cloud platform, such as Azure, for production use.
- Integrating the chatbot with various messaging channels or platforms to make it accessible to users.
Can I customize the behavior and responses of my chatbot?
Yes, Microsoft Bot Framework allows you to customize the behavior and responses of your chatbot to fit your specific requirements. You can define conversational flows, implement custom logic, handle user inputs, and dynamically generate responses based on user interactions.
Can I integrate external services and APIs with my chatbot?
Yes, you can integrate external services and APIs with your chatbot using the Bot Builder SDK. Microsoft Bot Framework provides connectors and adapters to easily connect with various services and APIs, allowing your chatbot to fetch data, perform actions, or leverage additional functionalities.
Can I deploy my chatbot to multiple messaging platforms?
Yes, Microsoft Bot Framework supports deploying chatbots to multiple messaging platforms simultaneously. You can configure your chatbot to work with popular messaging platforms like Microsoft Teams, Slack, Facebook Messenger, Skype, and more, expanding your chatbot’s reach to a wider audience.
Does Microsoft Bot Framework provide analytics and monitoring for chatbots?
Yes, Microsoft Bot Framework provides analytics and monitoring capabilities to track the performance and usage of your chatbot. You can gather insights on user interactions, conversation flow, user sentiment, and more to continuously improve your chatbot’s effectiveness.
Can I add natural language understanding capabilities to my chatbot?
Yes, Microsoft Bot Framework integrates with Azure Cognitive Services, including the Language Understanding Intelligent Service (LUIS), to add natural language understanding capabilities to your chatbot. LUIS allows you to define intents, entities, and utterances to train your chatbot to understand and interpret user inputs accurately.
Are there any resources available to learn more about building chatbots with Microsoft Bot Framework?
Yes, Microsoft provides extensive documentation, tutorials, and samples on the official Microsoft Bot Framework website. You can find step-by-step guides, code samples, and community resources to help you get started and learn more about building chatbots with the framework.
Is Microsoft Bot Framework suitable for both small-scale and enterprise-level chatbot development?
Yes, Microsoft Bot Framework is suitable for both small-scale and enterprise-level chatbot development. It provides the necessary tools, scalability options, and integrations to build chatbots of various complexities and cater to different business needs. The framework can scale to handle high volumes of requests and is used by organizations of all sizes for chatbot development.
Conclusion
We have discussed how to build a simple chatbot with Microsoft Bot Framework. We have covered the basic steps of creating a new bot project, defining the bot’s dialog, and integrating the bot with a messaging platform. While this example is simple, it can be extended to create more complex chatbots with more advanced dialog flow and functionality.
Microsoft Bot Framework provides a powerful and flexible platform for building chatbots that can be integrated with a variety of messaging platforms. It supports a range of programming languages, including C# and Node.js, making it accessible to developers with different backgrounds and skill levels. With the increasing demand for chatbots in various industries, Microsoft Bot Framework is an excellent tool for building high-quality chatbots quickly and efficiently.
Latest Topic
-
Cloud-Native Technologies: Best Practices
20 April, 2024 -
Generative AI with Llama 3: Shaping the Future
15 April, 2024 -
Mastering Llama 3: The Ultimate Guide
10 April, 2024
Category
- Assignment Help
- Homework Help
- Programming
- Trending Topics
- C Programming Assignment Help
- Art, Interactive, And Robotics
- Networked Operating Systems Programming
- Knowledge Representation & Reasoning Assignment Help
- Digital Systems Assignment Help
- Computer Design Assignment Help
- Artificial Life And Digital Evolution
- Coding and Fundamentals: Working With Collections
- UML Online Assignment Help
- Prolog Online Assignment Help
- Natural Language Processing Assignment Help
- Julia Assignment Help
- Golang Assignment Help
- Design Implementation Of Network Protocols
- Computer Architecture Assignment Help
- Object-Oriented Languages And Environments
- Coding Early Object and Algorithms: Java Coding Fundamentals
- Deep Learning In Healthcare Assignment Help
- Geometric Deep Learning Assignment Help
- Models Of Computation Assignment Help
- Systems Performance And Concurrent Computing
- Advanced Security Assignment Help
- Typescript Assignment Help
- Computational Media Assignment Help
- Design And Analysis Of Algorithms
- Geometric Modelling Assignment Help
- JavaScript Assignment Help
- MySQL Online Assignment Help
- Programming Practicum Assignment Help
- Public Policy, Legal, And Ethical Issues In Computing, Privacy, And Security
- Computer Vision
- Advanced Complexity Theory Assignment Help
- Big Data Mining Assignment Help
- Parallel Computing And Distributed Computing
- Law And Computer Science Assignment Help
- Engineering Distributed Objects For Cloud Computing
- Building Secure Computer Systems Assignment Help
- Ada Assignment Help
- R Programming Assignment Help
- Oracle Online Assignment Help
- Languages And Automata Assignment Help
- Haskell Assignment Help
- Economics And Computation Assignment Help
- ActionScript Assignment Help
- Audio Programming Assignment Help
- Bash Assignment Help
- Computer Graphics Assignment Help
- Groovy Assignment Help
- Kotlin Assignment Help
- Object Oriented Languages And Environments
- COBOL ASSIGNMENT HELP
- Bayesian Statistical Probabilistic Programming
- Computer Network Assignment Help
- Django Assignment Help
- Lambda Calculus Assignment Help
- Operating System Assignment Help
- Computational Learning Theory
- Delphi Assignment Help
- Concurrent Algorithms And Data Structures Assignment Help
- Machine Learning Assignment Help
- Human Computer Interface Assignment Help
- Foundations Of Data Networking Assignment Help
- Continuous Mathematics Assignment Help
- Compiler Assignment Help
- Computational Biology Assignment Help
- PostgreSQL Online Assignment Help
- Lua Assignment Help
- Human Computer Interaction Assignment Help
- Ethics And Responsible Innovation Assignment Help
- Communication And Ethical Issues In Computing
- Computer Science
- Combinatorial Optimisation Assignment Help
- Ethical Computing In Practice
- HTML Homework Assignment Help
- Linear Algebra Assignment Help
- Perl Assignment Help
- Artificial Intelligence Assignment Help
- Uncategorized
- Ethics And Professionalism Assignment Help
- Human Augmentics Assignment Help
- Linux Assignment Help
- PHP Assignment Help
- Assembly Language Assignment Help
- Dart Assignment Help
- Complete Python Bootcamp From Zero To Hero In Python Corrected Version
- Swift Assignment Help
- Computational Complexity Assignment Help
- Probability And Computing Assignment Help
- MATLAB Programming For Engineers
- Introduction To Statistical Learning
- Database Systems Implementation Assignment Help
- Computational Game Theory Assignment Help
- Database Assignment Help
- Probabilistic Model Checking Assignment Help
- Mathematics For Computer Science And Philosophy
- Introduction To Formal Proof Assignment Help
- Creative Coding Assignment Help
- Foundations Of Self-Programming Agents Assignment Help
- Machine Organization Assignment Help
- Software Design Assignment Help
- Data Communication And Networking Assignment Help
- Computational Biology
- Data Structure Assignment Help
- Foundations Of Software Engineering Assignment Help
- Mathematical Foundations Of Computing
- Principles Of Programming Languages Assignment Help
- Software Engineering Capstone Assignment Help
- Algorithms and Data Structures Assignment Help
No Comments