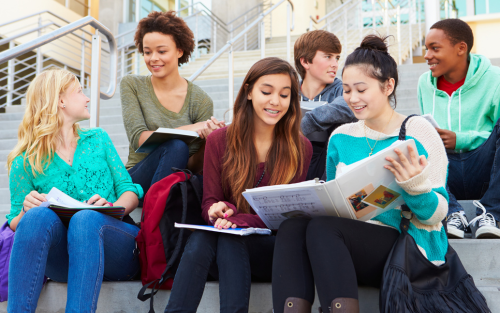
26 Apr Building A Simple E-commerce Website With Django
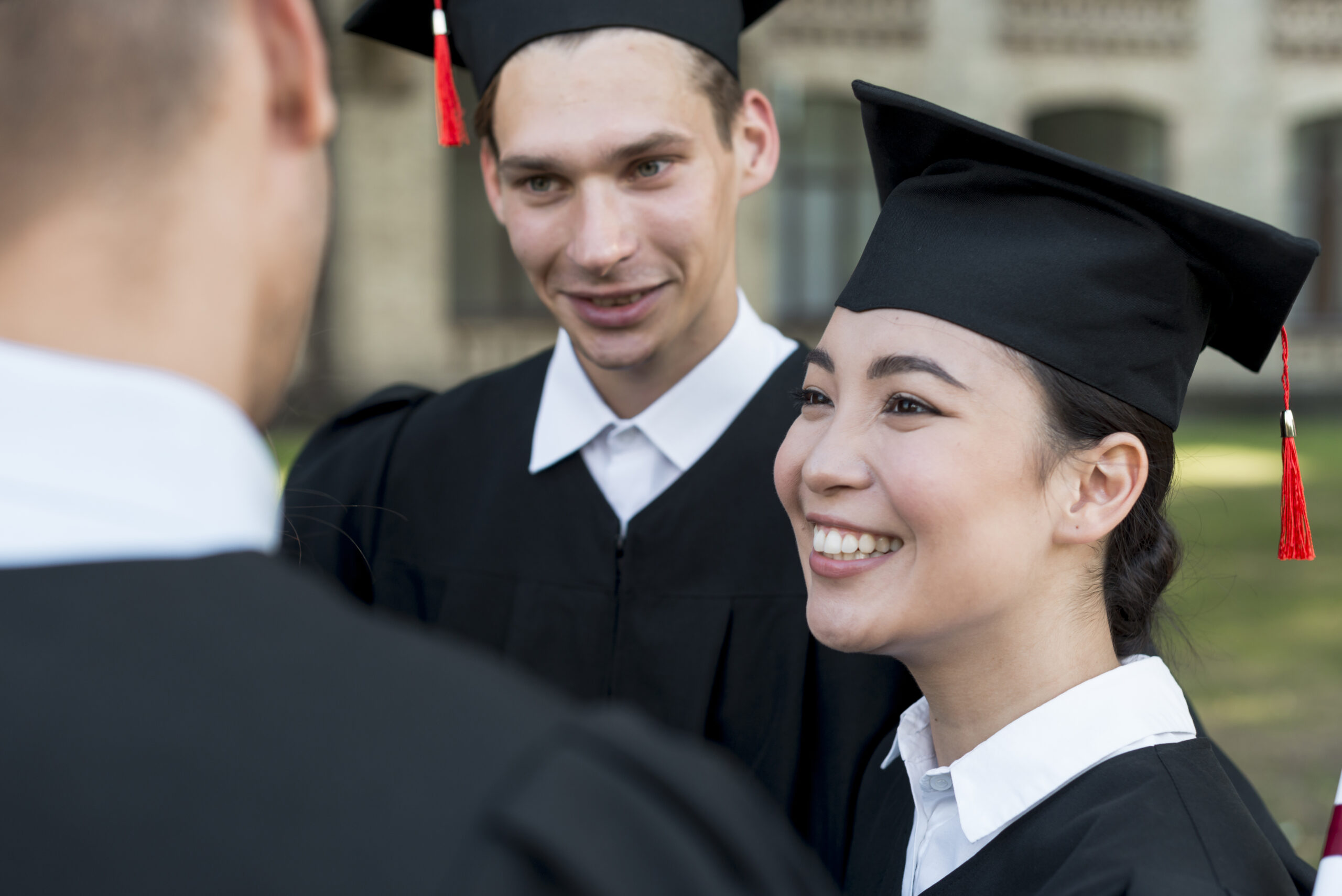
Django is a popular web framework for building scalable and secure web applications. It provides a robust set of tools and features that make it easy to build complex web applications with minimal effort. In this tutorial, we will walk you through the process of building a simple e-commerce website with Django.
Requirements
To follow along with this tutorial, you will need the following software installed on your computer:
- Python 3.x
- Django
- MySQL
Getting Started
First, we need to create a new Django project. Open your terminal or command prompt and run the following command:
django-admin startproject ecommerce
This command will create a new Django project named “ecommerce” in your current directory.
Next, we need to create a new Django app. Run the following command in your terminal:
python manage.py startapp store
This command will create a new Django app named “store” in your Django project.
Database Configuration
Next, we need to configure our database. In this tutorial, we will be using MySQL as our database. You can use any other database of your choice.
Open the settings.py file located in your Django project directory and update the DATABASES setting as shown below:
DATABASES = {
'default': {
'ENGINE': 'django.db.backends.mysql',
'NAME': 'ecommerce',
'USER': 'your_mysql_username',
'PASSWORD': 'your_mysql_password',
'HOST': 'localhost',
'PORT': '3306',
}
}
Replace “your_mysql_username” and “your_mysql_password” with your actual MySQL username and password.
Creating Models
Next, we need to create our models. Models define the structure of our database tables and how data is stored and retrieved from the database.
Open the models.py file located in your store app directory and define the following models:
from django.db import models
class Product(models.Model):
name = models.CharField(max_length=255)
description = models.TextField()
price = models.DecimalField(max_digits=10, decimal_places=2)
image = models.ImageField(upload_to='products/', blank=True, null=True)
class Order(models.Model):
product = models.ForeignKey(Product, on_delete=models.CASCADE)
quantity = models.IntegerField()
total_price = models.DecimalField(max_digits=10, decimal_places=2)
date_created = models.DateTimeField(auto_now_add=True)
In this code, we have defined two models – Product and Order. The Product model represents a product in our e-commerce website and has fields such as name, description, price, and image. The Order model represents an order placed by a customer and has fields such as product, quantity, total_price, and date_created.
Migrating the Database
Next, we need to migrate our models to the database. Run the following command in your terminal:
python manage.py makemigrations
This command will create migration files for our models.
Next, run the following command to apply the migrations to the database:
python manage.py migrate
This command will create the necessary database tables for our models.
Creating Views Next, we need to create our views. Views handle the logic of our web application and render the appropriate templates.
Open the views.py file located in your store app directory and define the following views:
from django.shortcuts import render
from .models import Product
def product_list(request):
products = Product.objects.all()
context = {
'products': products
}
return render(request, 'store/product_list.html', context)
Displaying Products
Now we need to display our products on the website. Let’s create a view for displaying products in our views.py file.
from django.shortcuts import render
from .models import Product
def product_list(request):
products = Product.objects.all()
return render(request, 'store/product_list.html', {'products': products})
Here, we have imported the Product model and defined a function called product_list. Inside the function, we have fetched all the products from the database using the Product.objects.all()
method and stored them in the products
variable. Then, we have passed the products
variable to the template as a context variable.
Next, we need to create a template for displaying the products. Let’s create a new file called product_list.html inside the templates/store/ directory.
{% extends 'store/base.html' %}
{% block content %}
<div class="row">
{% for product in products %}
<div class="col-md-4">
<div class="thumbnail">
<img src="{{ product.image.url }}" alt="{{ product.name }}" class="img-responsive">
<div class="caption">
<h3>{{ product.name }}</h3>
<p>{{ product.description }}</p>
<h4>${{ product.price }}</h4>
<a href="#" class="btn btn-primary" role="button">Add to Cart</a>
</div>
</div>
</div>
{% endfor %}
</div>
{% endblock %}
In the above code, we have extended the base.html template and defined a block called content. Inside the content block, we have created a loop to iterate over all the products and display them using Bootstrap thumbnails.
We have displayed the product name, description, price, and an add to cart button. We have also displayed the product image using the product.image.url
property.
Adding Navigation Links
Now, we need to add navigation links to our website. We will create a navbar that contains links to our home page and the product list page.
Let’s update the base.html template with the following code:
{% load static %}
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>{% block title %}{% endblock %} - Simple E-commerce Website</title>
<link rel="stylesheet" href="{% static 'css/bootstrap.min.css' %}">
</head>
<body>
<nav class="navbar navbar-inverse">
<div class="container-fluid">
<div class="navbar-header">
<a class="navbar-brand" href="{% url 'home' %}">Simple E-commerce Website</a>
</div>
<ul class="nav navbar-nav">
<li><a href="{% url 'product_list' %}">Products</a></li>
</ul>
</div>
</nav>
<div class="container">
{% block content %}
{% endblock %}
</div>
<script src="{% static 'js/jquery.min.js' %}"></script>
<script src="{% static 'js/bootstrap.min.js' %}"></script>
</body>
</html>
In the above code, we have created a navigation bar using Bootstrap’s navbar-inverse class. We have added a navbar-brand that links to our home page, and a Products link that links to our product list page.
Latest Topic
-
Cloud-Native Technologies: Best Practices
20 April, 2024 -
Generative AI with Llama 3: Shaping the Future
15 April, 2024 -
Mastering Llama 3: The Ultimate Guide
10 April, 2024
Category
- Assignment Help
- Homework Help
- Programming
- Trending Topics
- C Programming Assignment Help
- Art, Interactive, And Robotics
- Networked Operating Systems Programming
- Knowledge Representation & Reasoning Assignment Help
- Digital Systems Assignment Help
- Computer Design Assignment Help
- Artificial Life And Digital Evolution
- Coding and Fundamentals: Working With Collections
- UML Online Assignment Help
- Prolog Online Assignment Help
- Natural Language Processing Assignment Help
- Julia Assignment Help
- Golang Assignment Help
- Design Implementation Of Network Protocols
- Computer Architecture Assignment Help
- Object-Oriented Languages And Environments
- Coding Early Object and Algorithms: Java Coding Fundamentals
- Deep Learning In Healthcare Assignment Help
- Geometric Deep Learning Assignment Help
- Models Of Computation Assignment Help
- Systems Performance And Concurrent Computing
- Advanced Security Assignment Help
- Typescript Assignment Help
- Computational Media Assignment Help
- Design And Analysis Of Algorithms
- Geometric Modelling Assignment Help
- JavaScript Assignment Help
- MySQL Online Assignment Help
- Programming Practicum Assignment Help
- Public Policy, Legal, And Ethical Issues In Computing, Privacy, And Security
- Computer Vision
- Advanced Complexity Theory Assignment Help
- Big Data Mining Assignment Help
- Parallel Computing And Distributed Computing
- Law And Computer Science Assignment Help
- Engineering Distributed Objects For Cloud Computing
- Building Secure Computer Systems Assignment Help
- Ada Assignment Help
- R Programming Assignment Help
- Oracle Online Assignment Help
- Languages And Automata Assignment Help
- Haskell Assignment Help
- Economics And Computation Assignment Help
- ActionScript Assignment Help
- Audio Programming Assignment Help
- Bash Assignment Help
- Computer Graphics Assignment Help
- Groovy Assignment Help
- Kotlin Assignment Help
- Object Oriented Languages And Environments
- COBOL ASSIGNMENT HELP
- Bayesian Statistical Probabilistic Programming
- Computer Network Assignment Help
- Django Assignment Help
- Lambda Calculus Assignment Help
- Operating System Assignment Help
- Computational Learning Theory
- Delphi Assignment Help
- Concurrent Algorithms And Data Structures Assignment Help
- Machine Learning Assignment Help
- Human Computer Interface Assignment Help
- Foundations Of Data Networking Assignment Help
- Continuous Mathematics Assignment Help
- Compiler Assignment Help
- Computational Biology Assignment Help
- PostgreSQL Online Assignment Help
- Lua Assignment Help
- Human Computer Interaction Assignment Help
- Ethics And Responsible Innovation Assignment Help
- Communication And Ethical Issues In Computing
- Computer Science
- Combinatorial Optimisation Assignment Help
- Ethical Computing In Practice
- HTML Homework Assignment Help
- Linear Algebra Assignment Help
- Perl Assignment Help
- Artificial Intelligence Assignment Help
- Uncategorized
- Ethics And Professionalism Assignment Help
- Human Augmentics Assignment Help
- Linux Assignment Help
- PHP Assignment Help
- Assembly Language Assignment Help
- Dart Assignment Help
- Complete Python Bootcamp From Zero To Hero In Python Corrected Version
- Swift Assignment Help
- Computational Complexity Assignment Help
- Probability And Computing Assignment Help
- MATLAB Programming For Engineers
- Introduction To Statistical Learning
- Database Systems Implementation Assignment Help
- Computational Game Theory Assignment Help
- Database Assignment Help
- Probabilistic Model Checking Assignment Help
- Mathematics For Computer Science And Philosophy
- Introduction To Formal Proof Assignment Help
- Creative Coding Assignment Help
- Foundations Of Self-Programming Agents Assignment Help
- Machine Organization Assignment Help
- Software Design Assignment Help
- Data Communication And Networking Assignment Help
- Computational Biology
- Data Structure Assignment Help
- Foundations Of Software Engineering Assignment Help
- Mathematical Foundations Of Computing
- Principles Of Programming Languages Assignment Help
- Software Engineering Capstone Assignment Help
- Algorithms and Data Structures Assignment Help
No Comments