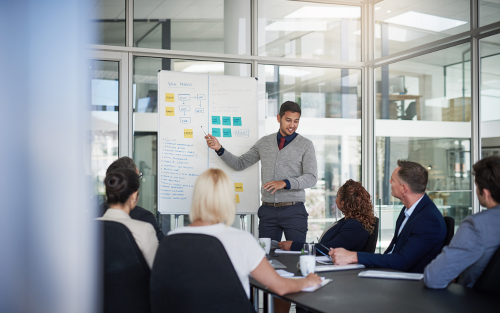
02 May Building A Simple Weather App With React Native
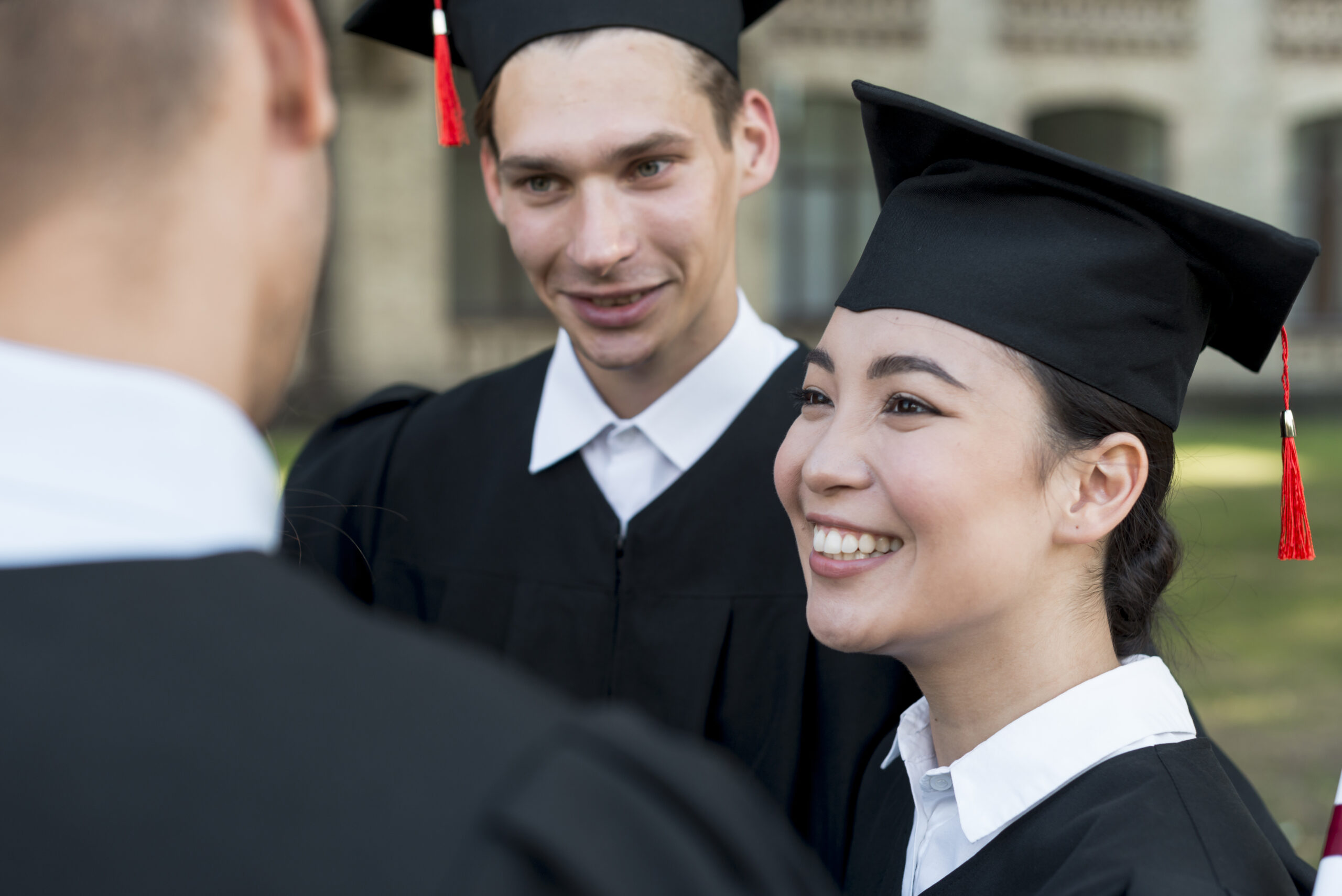
Introduction
In recent years, mobile app development has gained immense popularity due to the increasing number of mobile users. React Native, a JavaScript framework, has emerged as a popular choice for building mobile apps due to its simplicity, cross-platform compatibility, and the ability to reuse code across multiple platforms. In this article, we will build a simple weather app using React Native.
Building a weather app with React Native allows you to create a mobile application that runs on multiple platforms using a single codebase. This tutorial will guide you through the step-by-step process of building a simple weather app.
You’ll start by setting up a React Native project and creating the necessary components for your weather app. You’ll learn how to manage the app’s state, handle user interactions, and make API requests to fetch weather data. The tutorial will cover UI design principles and show you how to create a visually appealing interface for displaying weather information.
Throughout the tutorial, you’ll gain insights into React Native best practices and techniques for efficient app development. You’ll discover how to test your app using simulators or real devices, and learn about the deployment process for both iOS and Android platforms.
By the end of the tutorial, you’ll have a functional weather app that users can install on their devices and use to check the current weather conditions. This project will provide you with a solid foundation in React Native app development, empowering you to create more complex and feature-rich applications in the future.
Building a simple weather app with React Native demonstrates the power and versatility of this framework for mobile app development. Whether you’re a beginner or an experienced developer, this tutorial will equip you with the knowledge and skills to create cross-platform mobile apps using React Native.
Requirements
To build a weather app with React Native, we will need the following:
-A code editor (such as VS Code)
-Node.js and npm installed on the system
-A mobile emulator or a physical device for testing the app
Getting Started
Let’s start by creating a new React Native project using the Expo CLI. The Expo CLI is a set of tools built around React Native and makes it easy to develop, build, and publish React Native apps. To install the Expo CLI, run the following command in the terminal:
npm install -g expo-cli
Once the Expo CLI is installed, create a new project using the following command:
expo init WeatherApp
This will create a new directory called “WeatherApp” and set up a basic React Native project inside it. Navigate to the project directory and start the development server using the following commands:
cd WeatherApp
npm start
This will start the development server and open the Expo Developer Tools in the browser. From here, you can choose to run the app on an Android or iOS emulator or scan the QR code to run the app on a physical device using the Expo client app.
Designing the Weather App
Before we start writing the code, let’s design the layout of the weather app. We will use the “react-native-elements” library to quickly create a beautiful UI. Open the App.js file and replace the contents with the following code:
import React from 'react';
import { StyleSheet, View } from 'react-native';
import { Header, Icon, Input, Button, Text } from 'react-native-elements';
export default function App() {
return (
<View style={styles.container}>
<Header
backgroundColor=“#1E90FF”
centerComponent={{ text: ‘Weather App‘, style: { color: ‘#fff‘ } }}
/>
<View style={styles.content}>
<Input
placeholder=‘Enter City Name’
leftIcon={<Icon name=‘search’ size={24} color=‘#1E90FF’ />}
style={styles.input}
/>
<Button
title=‘Get Weather’
buttonStyle={styles.button}
/>
<View style={styles.result}>
<Text h4>Weather Information</Text>
</View>
</View>
</View>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
},
content: {
flex: 1,
justifyContent: ‘center’,
alignItems: ‘center’,
padding: 20,
},
input: {
marginTop: 20,
marginBottom: 20,
},
button: {
backgroundColor: ‘#1E90FF’,
},
result: {
marginTop: 50,
alignItems: ‘center’,
},
});
This code sets up a basic layout for the weather app with a header, input field, button, and a result container.
Fetching Weather Data
To fetch weather data, we will use the fetch
function provided by JavaScript. Here’s an example code snippet that fetches weather data from the OpenWeatherMap API:
const API_KEY = 'YOUR_API_KEY_HERE';
const API_URL = `https://api.openweathermap.org/data/2.5/weather?q=${city}&appid=${API_KEY}`;
fetch(API_URL)
.then(response => response.json())
.then(data => {
// Do something with the data
})
.catch(error => {
// Handle errors
});
In the above code snippet, we first define our API key and API URL, and then use the fetch
function to make a GET request to the API URL. We then use the json
method to parse the response data into a JavaScript object, and handle any errors that may occur.
Note that in the API URL, we include the city
parameter which specifies the name of the city for which we want to fetch weather data. We can dynamically set the value of this parameter based on the user’s input.
Once we have fetched the weather data, we can use it to display the weather information in our app. We can also use this data to implement additional features, such as displaying a different background image depending on the weather conditions.
Text
and Image
, to display the weather information.Let’s start by creating a new component called WeatherInfo
that will display the weather information. Here’s an example code snippet:
import React from 'react';
import { View, Text, Image, StyleSheet } from 'react-native';
const WeatherInfo = ({ weatherData }) => {
const { main, weather } = weatherData;
return (
<View style={styles.container}>
<Text style={styles.temp}>{Math.round(main.temp)}°C</Text>
<Image
style={styles.icon}
source={{ uri: `https://openweathermap.org/img/w/${weather[0].icon}.png` }}
/>
<Text style={styles.description}>{weather[0].description}</Text>
</View>
);
};
const styles = StyleSheet.create({
container: {
alignItems: ‘center’,
},
temp: {
fontSize: 64,
fontWeight: ‘bold’,
},
icon: {
width: 100,
height: 100,
},
description: {
textTransform: ‘capitalize’,
fontSize: 24,
},
});
export default WeatherInfo;
In the above code snippet, we define the WeatherInfo
component that takes in the weatherData
object as a prop. This object contains the weather information that we fetched from the API.
We then use various React Native components to display the weather information. The Text
component is used to display the temperature in Celsius, while the Image
component is used to display the weather icon. Note that we use the uri
prop to specify the URL of the weather icon, which we construct using the weather[0].icon
property from the weatherData
object.
Finally, we use the Text
component again to display the weather description, which we capitalize using the textTransform
style property.
Now that we have created the WeatherInfo
component, we can use it in our main app component to display the weather information. Here’s an example code snippet that demonstrates how to use the WeatherInfo
component:
import React, { useState } from 'react';
import { View, TextInput, Button, StyleSheet } from 'react-native';
import WeatherInfo from './WeatherInfo';
const App = () => {
const [city, setCity] = useState(”);
const [weatherData, setWeatherData] = useState(null);
const fetchWeatherData = async () => {
const API_KEY = ‘YOUR_API_KEY_HERE’;
const API_URL = `https://api.openweathermap.org/data/2.5/weather?q=${city}&units=metric&appid=${API_KEY}`;
try {
const response = await fetch(API_URL);
const data = await response.json();
setWeatherData(data);
} catch (error) {
console.error(error);
}
};
return (
<View style={styles.container}>
<TextInput
style={styles.input}
placeholder=“Enter a city”
value={city}
onChangeText={text => setCity(text)}
/>
<Button title=“Get Weather” onPress={fetchWeatherData} />
{weatherData && <WeatherInfo weatherData={weatherData} />}
</View>
);
};
const styles = StyleSheet.create({
container: {
flex: 1,
alignItems: ‘center’,
justifyContent: ‘center’,
backgroundColor: ‘#fff’,
},
input: {
borderWidth
conclusion
In conclusion, building a simple weather app with React Native is a great way to get started with mobile app development using a popular framework. By using the OpenWeatherMap API, we were able to fetch real-time weather data and display it in our app using various React Native components.
We started by setting up our development environment and creating a new React Native project. Then, we installed the necessary dependencies and set up the API call to fetch weather data from OpenWeatherMap. Next, we created a custom component to display the weather information, including the current temperature, weather description, and icon.
We also added user input to allow the user to enter a city or location of their choice, and we handled errors and loading states to provide a better user experience.
Overall, building a simple weather app with React Native is a great way to learn the basics of the framework and get started with mobile app development. With React Native, we can build high-quality apps for both iOS and Android platforms using a single codebase, which saves time and effort. We hope this tutorial has been helpful, and we encourage you to keep exploring the possibilities of React Native and mobile app development.
Latest Topic
-
Cloud-Native Technologies: Best Practices
20 April, 2024 -
Generative AI with Llama 3: Shaping the Future
15 April, 2024 -
Mastering Llama 3: The Ultimate Guide
10 April, 2024
Category
- Assignment Help
- Homework Help
- Programming
- Trending Topics
- C Programming Assignment Help
- Art, Interactive, And Robotics
- Networked Operating Systems Programming
- Knowledge Representation & Reasoning Assignment Help
- Digital Systems Assignment Help
- Computer Design Assignment Help
- Artificial Life And Digital Evolution
- Coding and Fundamentals: Working With Collections
- UML Online Assignment Help
- Prolog Online Assignment Help
- Natural Language Processing Assignment Help
- Julia Assignment Help
- Golang Assignment Help
- Design Implementation Of Network Protocols
- Computer Architecture Assignment Help
- Object-Oriented Languages And Environments
- Coding Early Object and Algorithms: Java Coding Fundamentals
- Deep Learning In Healthcare Assignment Help
- Geometric Deep Learning Assignment Help
- Models Of Computation Assignment Help
- Systems Performance And Concurrent Computing
- Advanced Security Assignment Help
- Typescript Assignment Help
- Computational Media Assignment Help
- Design And Analysis Of Algorithms
- Geometric Modelling Assignment Help
- JavaScript Assignment Help
- MySQL Online Assignment Help
- Programming Practicum Assignment Help
- Public Policy, Legal, And Ethical Issues In Computing, Privacy, And Security
- Computer Vision
- Advanced Complexity Theory Assignment Help
- Big Data Mining Assignment Help
- Parallel Computing And Distributed Computing
- Law And Computer Science Assignment Help
- Engineering Distributed Objects For Cloud Computing
- Building Secure Computer Systems Assignment Help
- Ada Assignment Help
- R Programming Assignment Help
- Oracle Online Assignment Help
- Languages And Automata Assignment Help
- Haskell Assignment Help
- Economics And Computation Assignment Help
- ActionScript Assignment Help
- Audio Programming Assignment Help
- Bash Assignment Help
- Computer Graphics Assignment Help
- Groovy Assignment Help
- Kotlin Assignment Help
- Object Oriented Languages And Environments
- COBOL ASSIGNMENT HELP
- Bayesian Statistical Probabilistic Programming
- Computer Network Assignment Help
- Django Assignment Help
- Lambda Calculus Assignment Help
- Operating System Assignment Help
- Computational Learning Theory
- Delphi Assignment Help
- Concurrent Algorithms And Data Structures Assignment Help
- Machine Learning Assignment Help
- Human Computer Interface Assignment Help
- Foundations Of Data Networking Assignment Help
- Continuous Mathematics Assignment Help
- Compiler Assignment Help
- Computational Biology Assignment Help
- PostgreSQL Online Assignment Help
- Lua Assignment Help
- Human Computer Interaction Assignment Help
- Ethics And Responsible Innovation Assignment Help
- Communication And Ethical Issues In Computing
- Computer Science
- Combinatorial Optimisation Assignment Help
- Ethical Computing In Practice
- HTML Homework Assignment Help
- Linear Algebra Assignment Help
- Perl Assignment Help
- Artificial Intelligence Assignment Help
- Uncategorized
- Ethics And Professionalism Assignment Help
- Human Augmentics Assignment Help
- Linux Assignment Help
- PHP Assignment Help
- Assembly Language Assignment Help
- Dart Assignment Help
- Complete Python Bootcamp From Zero To Hero In Python Corrected Version
- Swift Assignment Help
- Computational Complexity Assignment Help
- Probability And Computing Assignment Help
- MATLAB Programming For Engineers
- Introduction To Statistical Learning
- Database Systems Implementation Assignment Help
- Computational Game Theory Assignment Help
- Database Assignment Help
- Probabilistic Model Checking Assignment Help
- Mathematics For Computer Science And Philosophy
- Introduction To Formal Proof Assignment Help
- Creative Coding Assignment Help
- Foundations Of Self-Programming Agents Assignment Help
- Machine Organization Assignment Help
- Software Design Assignment Help
- Data Communication And Networking Assignment Help
- Computational Biology
- Data Structure Assignment Help
- Foundations Of Software Engineering Assignment Help
- Mathematical Foundations Of Computing
- Principles Of Programming Languages Assignment Help
- Software Engineering Capstone Assignment Help
- Algorithms and Data Structures Assignment Help
No Comments