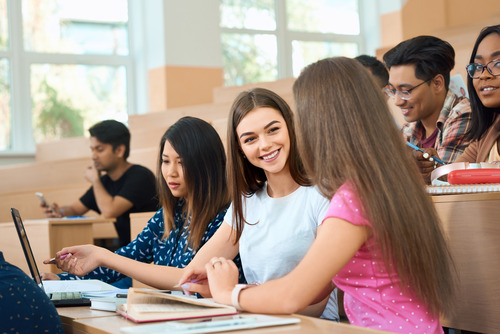
29 Apr Data Structures in C++
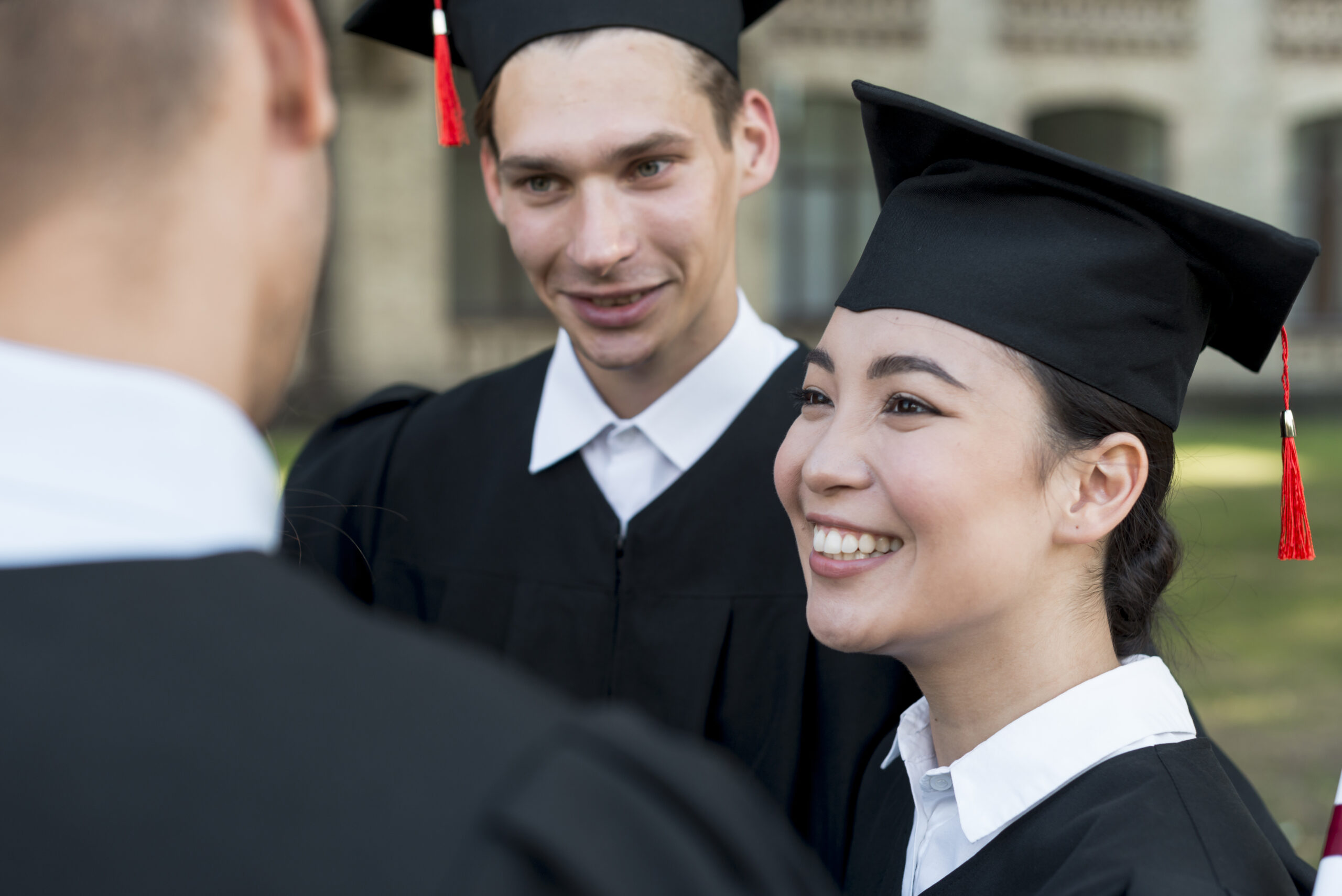
Data Structures are important for programming and computational problem solving. They are the basic building blocks of any program or application that deals with data. Data Structures are used to organize and manipulate data in a way that allows for efficient and effective processing. C++ is a popular programming language that is often used for implementing Data Structures.
There are many different types of Data Structures, each with their own unique properties and advantages. Some of the most commonly used Data Structures in C++ include:
Arrays – An array is a collection of elements of the same type that are stored in contiguous memory locations. Arrays are commonly used for storing and manipulating data in a program.
Linked Lists – A linked list is a collection of nodes, where each node contains a value and a pointer to the next node in the list. Linked lists are commonly used for implementing dynamic data structures.
Stacks – A stack is a data structure that follows the Last-In-First-Out (LIFO) principle. It can be thought of as a pile of books, where the last book added is the first book removed.
Queues – A queue is a data structure that follows the First-In-First-Out (FIFO) principle. It can be thought of as a line of people waiting to enter a movie theater, where the person who arrived first is the first to enter.
Trees – A tree is a hierarchical data structure that consists of nodes connected by edges. Each node contains a value and pointers to its child nodes. Trees are commonly used for representing hierarchical relationships in data.
Graphs – A graph is a collection of vertices connected by edges. Graphs are commonly used for representing complex relationships between data.
C++ provides a number of built-in data types, such as int, float, char, etc., but these are not sufficient for solving many real-world problems. In order to efficiently solve such problems, we need to use specialized data structures.
Implementing Data Structures in C++ involves defining classes or structures that hold the data and functions to manipulate that data. For example, a linked list can be implemented using a class that defines the structure of each node and functions to insert, delete, and traverse the list.
One of the main advantages of using Data Structures is that they allow for efficient processing of data. For example, searching, inserting, and deleting elements in a sorted array can be done in O(log n) time using binary search. Similarly, inserting and deleting elements in a heap can be done in O(log n) time.
In conclusion, Data Structures are an essential tool for any programmer or computational problem solver. They allow for efficient and effective processing of data, and are a key component in the development of many algorithms and applications. C++ provides a powerful set of tools for implementing Data Structures, making it a popular language for programming in this area.
At Programming Homework Tutors, we believe in providing our students with practical, real-world examples of how to apply the concepts they learn in class. That’s why we’ve developed a variety of sample projects to help you see how our courses can be used to create impactful solutions in your field of study.
Please typeset your answers to the following homework problems (you may save this file as week8hwk_your_lastname.doc and then directly type your answers after each problem). Submit this assignment on Blackboard after you have finished by the due time.
The Fibonacci sequence is the series of integers
0, 1, 1, 2, 3, 5, 8, 13, 21, 34, 55, 89, …
See the pattern? Each element in the series is the sum of the preceding two items. There is a recursive formula for calculating the n-th number of the sequence (the 0-th number if Fibonacci(0)=0):
Fibonacci(n) = n, if n = 0 or 1, and
Fibonacci(n) = Fibonacci(n-2) + Fibonacci(n-1), if n > 1
Write a recursive version of the C++ function Fibonacci.
Every budding computer scientist must grapple with certain classic problems. The tower of Hanoi (see the Figure below) is one of the most famous of these. Legend has it that in a temple in the Far East, priests are attempting to move a stack of disks from on peg to another. The initial stack had 64 disks threaded onto one peg and arranged from bottom to top by decreasing size. The priests are attempting to move the stack from this peg to a second peg under the constraints that exactly one disk is moved at a time, and at no time may a larger disk be placed above a smaller disk. A third peg is available for temporarily holding disks. Supposedly, the world will end when the priests complete their tasks, so there is little incentive for us to facilitate their efforts.
Figure: The Tower of Hanoi for the case with three disks.
Let us assume that the priests are attempting to move the disks from peg 1 to peg 3. We wish to develop an algorithm that will print the precise sequence of peg-to-peg disk transfers.
If we were to approach this problem with conventional methods, we would rapidly find ourselves hopelessly knotted up in managing the disks. Instead, if we attack the problem with recursion in mind, it immediately becomes tractable. Moving n disks can be viewed in terms of moving only n-1 disks (hence, the recursion), as follows:
Move n-1 disks from peg 1 to peg 2, using peg 3 as a temporary holding area.
Move the last disk (the largest) from peg 1 to peg 3.
Move the n-1 disks from peg 2 to peg 3, using peg 1 as a temporary holding area.
The process ends when the last task involves moving n=1 disk, i.e., the base case. This is accomplished by trivially moving the disk without the need for a temporary holding area.
Write a program to solve the Towers of Hanoi problem and show your testing results for the case of moving a stack of five disks from peg 1 to peg 3. Use a recursive function (i.e., the function calls itself in the function body) with four parameters:
(a) The number of disks to be moved.
(b) The peg on which these disks are initially threaded.
(c) The peg on which this stack of disks is to be moved.
(d) The peg to be used as a temporary holding area.
Your program should print the precise instructions it will take to move the disks from the starting peg to the destination peg. For example, to move a stack of three disks from peg 1 to peg 3, your program should print the following series of moves;
1 –> 3 (This means move one disk from peg 1 to peg 3)
1 –> 2
3 –> 2
1 –> 3
2 –> 1
2 –> 3
1 –> 3
Mark the following statements as true or false.
A binary tree must be nonempty.
The level of the root node is 0.
If a tree has only one node, the height of this tree is 0 because the number of levels is 0.
The inorder traversal of a binary tree always outputs the data in ascending order.
Problems 4-9 will be using the following binary tree.
Find LA, the node in the left subtree of A.
Find RA, the node in the right subtree of A.
Find RB, the node in the left subtree of B.
List the nodes of this binary tree in an inorder sequence.
List the nodes of this binary tree in a preorder sequence.
List the nodes of this binary tree in a postorder sequence.
Problems 10-14 will be using the following binary search tree.
List the path from the node with info 80 to the node with info 79.
A node with info 35 is to be inserted in the tree. List the nodes that are visited by the function insert to insert 35. Redraw the tree after inserting 35. (You may draw on a paper and then scan it or take a picture of it and insert the picture in your solutions file.)
Delete the node 52 and redraw the binary tree. (You may draw on a paper and then scan it or take a picture of it and insert the picture in your solutions file.)
Delete the node 40 and redraw the binary tree. (You may draw on a paper and then scan it or take a picture of it and insert the picture in your solutions file.)
Delete nodes 80 and 58 in that order. Redraw the binary tree after each deletion. (You may draw on a paper and then scan it or take a picture of it and insert the picture in your solutions file.)
Disclaimer
The sample projects provided on our website are intended to be used as a guide and reference for educational purposes only. While we have made every effort to ensure that the projects are accurate and up-to-date, we do not guarantee their accuracy or completeness. The projects should be used at your own discretion, and we are not responsible for any loss or damage that may result from their use.
At Programming Homework Tutors, we are dedicated to helping students and educators achieve their goals by providing them with the resources they need to succeed. Our website offers a variety of tools and resources that can help you with the project mentioned above.
Whether you need help with research, project management, or technical support, our team of experts is here to assist you every step of the way. We offer online courses, tutorials, and community forums where you can connect with other learners and get the support you need to succeed.
If you’re looking to take your skills to the next level and make an impact in your field, we invite you to explore our website and see how we can help you achieve your goals.
Latest Topic
-
Cloud-Native Technologies: Best Practices
20 April, 2024 -
Generative AI with Llama 3: Shaping the Future
15 April, 2024 -
Mastering Llama 3: The Ultimate Guide
10 April, 2024
Category
- Assignment Help
- Homework Help
- Programming
- Trending Topics
- C Programming Assignment Help
- Art, Interactive, And Robotics
- Networked Operating Systems Programming
- Knowledge Representation & Reasoning Assignment Help
- Digital Systems Assignment Help
- Computer Design Assignment Help
- Artificial Life And Digital Evolution
- Coding and Fundamentals: Working With Collections
- UML Online Assignment Help
- Prolog Online Assignment Help
- Natural Language Processing Assignment Help
- Julia Assignment Help
- Golang Assignment Help
- Design Implementation Of Network Protocols
- Computer Architecture Assignment Help
- Object-Oriented Languages And Environments
- Coding Early Object and Algorithms: Java Coding Fundamentals
- Deep Learning In Healthcare Assignment Help
- Geometric Deep Learning Assignment Help
- Models Of Computation Assignment Help
- Systems Performance And Concurrent Computing
- Advanced Security Assignment Help
- Typescript Assignment Help
- Computational Media Assignment Help
- Design And Analysis Of Algorithms
- Geometric Modelling Assignment Help
- JavaScript Assignment Help
- MySQL Online Assignment Help
- Programming Practicum Assignment Help
- Public Policy, Legal, And Ethical Issues In Computing, Privacy, And Security
- Computer Vision
- Advanced Complexity Theory Assignment Help
- Big Data Mining Assignment Help
- Parallel Computing And Distributed Computing
- Law And Computer Science Assignment Help
- Engineering Distributed Objects For Cloud Computing
- Building Secure Computer Systems Assignment Help
- Ada Assignment Help
- R Programming Assignment Help
- Oracle Online Assignment Help
- Languages And Automata Assignment Help
- Haskell Assignment Help
- Economics And Computation Assignment Help
- ActionScript Assignment Help
- Audio Programming Assignment Help
- Bash Assignment Help
- Computer Graphics Assignment Help
- Groovy Assignment Help
- Kotlin Assignment Help
- Object Oriented Languages And Environments
- COBOL ASSIGNMENT HELP
- Bayesian Statistical Probabilistic Programming
- Computer Network Assignment Help
- Django Assignment Help
- Lambda Calculus Assignment Help
- Operating System Assignment Help
- Computational Learning Theory
- Delphi Assignment Help
- Concurrent Algorithms And Data Structures Assignment Help
- Machine Learning Assignment Help
- Human Computer Interface Assignment Help
- Foundations Of Data Networking Assignment Help
- Continuous Mathematics Assignment Help
- Compiler Assignment Help
- Computational Biology Assignment Help
- PostgreSQL Online Assignment Help
- Lua Assignment Help
- Human Computer Interaction Assignment Help
- Ethics And Responsible Innovation Assignment Help
- Communication And Ethical Issues In Computing
- Computer Science
- Combinatorial Optimisation Assignment Help
- Ethical Computing In Practice
- HTML Homework Assignment Help
- Linear Algebra Assignment Help
- Perl Assignment Help
- Artificial Intelligence Assignment Help
- Uncategorized
- Ethics And Professionalism Assignment Help
- Human Augmentics Assignment Help
- Linux Assignment Help
- PHP Assignment Help
- Assembly Language Assignment Help
- Dart Assignment Help
- Complete Python Bootcamp From Zero To Hero In Python Corrected Version
- Swift Assignment Help
- Computational Complexity Assignment Help
- Probability And Computing Assignment Help
- MATLAB Programming For Engineers
- Introduction To Statistical Learning
- Database Systems Implementation Assignment Help
- Computational Game Theory Assignment Help
- Database Assignment Help
- Probabilistic Model Checking Assignment Help
- Mathematics For Computer Science And Philosophy
- Introduction To Formal Proof Assignment Help
- Creative Coding Assignment Help
- Foundations Of Self-Programming Agents Assignment Help
- Machine Organization Assignment Help
- Software Design Assignment Help
- Data Communication And Networking Assignment Help
- Computational Biology
- Data Structure Assignment Help
- Foundations Of Software Engineering Assignment Help
- Mathematical Foundations Of Computing
- Principles Of Programming Languages Assignment Help
- Software Engineering Capstone Assignment Help
- Algorithms and Data Structures Assignment Help
No Comments