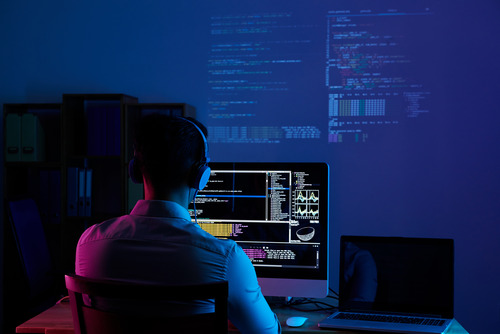
29 Apr Data Visualization – Plotting Temperatures
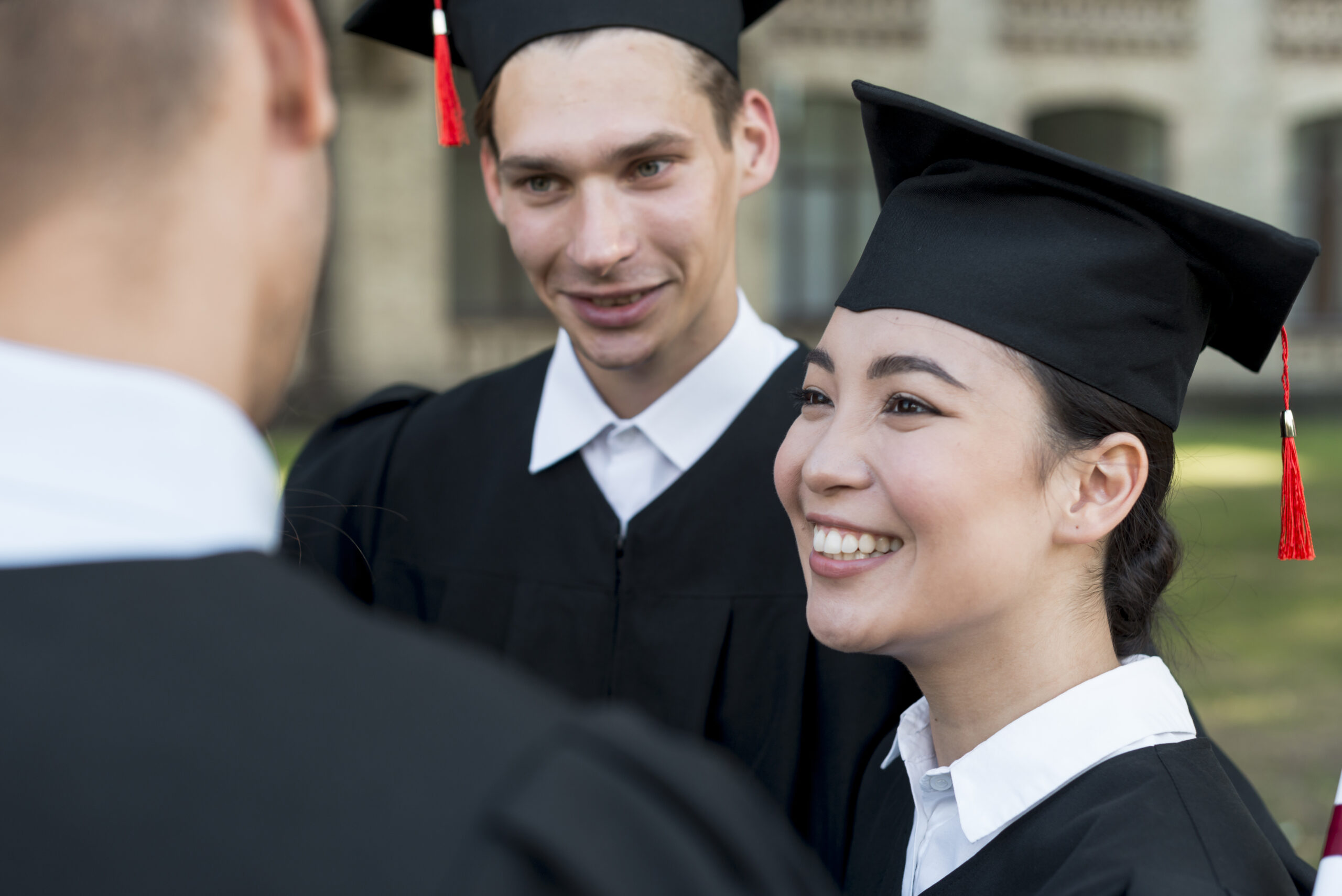
Data visualization is an important aspect of data analysis as it allows us to understand complex data sets by presenting them in a visual format. In this article, we will discuss how to plot temperature data using Python’s matplotlib library. We will begin by discussing the data set we will be working with, then move on to the steps required to create the visualization.
Data Set:
The data set we will be using is a collection of temperature readings for a particular location over the course of a year. The data is in CSV format and contains two columns: date and temperature. The date column contains the date of the temperature reading, while the temperature column contains the temperature in degrees Celsius.
Steps:
Step 1: Importing the Libraries
To begin, we need to import the required libraries. We will be using the pandas library to read in the data, and the matplotlib library to create the visualization. We can import the libraries using the following code:
import pandas as pd
import matplotlib.pyplot as plt
Step 2: Reading in the Data
Next, we need to read in the data using the pandas library. We can do this using the read_csv() function. We will read in the data and store it in a pandas DataFrame:
df = pd.read_csv('temperature_data.csv')
Step 3: Cleaning the Data
Before we can create the visualization, we need to clean the data. This involves removing any missing values and converting the temperature column to Fahrenheit. We can do this using the following code:
# Removing missing values
df = df.dropna()
# Converting temperature to Fahrenheit
df[‘temperature’] = df[‘temperature’] * 1.8 + 32
Step 4: Creating the Visualization
Now that the data is cleaned, we can create the visualization. We will be creating a line plot of the temperature over time. We can do this using the following code:
# Creating the plot
plt.plot(df['date'], df['temperature'])
# Adding labels and title
plt.xlabel(‘Date’)
plt.ylabel(‘Temperature (F)’)
plt.title(‘Temperature over Time’)
# Displaying the plot
plt.show()
Conclusion
In this article, we have discussed how to plot temperature data using Python’s matplotlib library. We began by discussing the data set we will be working with, then moved on to the steps required to create the visualization. By following these steps, we can create an informative and visually appealing plot of the temperature over time.
Latest Topic
-
Cloud-Native Technologies: Best Practices
20 April, 2024 -
Generative AI with Llama 3: Shaping the Future
15 April, 2024 -
Mastering Llama 3: The Ultimate Guide
10 April, 2024
Category
- Assignment Help
- Homework Help
- Programming
- Trending Topics
- C Programming Assignment Help
- Art, Interactive, And Robotics
- Networked Operating Systems Programming
- Knowledge Representation & Reasoning Assignment Help
- Digital Systems Assignment Help
- Computer Design Assignment Help
- Artificial Life And Digital Evolution
- Coding and Fundamentals: Working With Collections
- UML Online Assignment Help
- Prolog Online Assignment Help
- Natural Language Processing Assignment Help
- Julia Assignment Help
- Golang Assignment Help
- Design Implementation Of Network Protocols
- Computer Architecture Assignment Help
- Object-Oriented Languages And Environments
- Coding Early Object and Algorithms: Java Coding Fundamentals
- Deep Learning In Healthcare Assignment Help
- Geometric Deep Learning Assignment Help
- Models Of Computation Assignment Help
- Systems Performance And Concurrent Computing
- Advanced Security Assignment Help
- Typescript Assignment Help
- Computational Media Assignment Help
- Design And Analysis Of Algorithms
- Geometric Modelling Assignment Help
- JavaScript Assignment Help
- MySQL Online Assignment Help
- Programming Practicum Assignment Help
- Public Policy, Legal, And Ethical Issues In Computing, Privacy, And Security
- Computer Vision
- Advanced Complexity Theory Assignment Help
- Big Data Mining Assignment Help
- Parallel Computing And Distributed Computing
- Law And Computer Science Assignment Help
- Engineering Distributed Objects For Cloud Computing
- Building Secure Computer Systems Assignment Help
- Ada Assignment Help
- R Programming Assignment Help
- Oracle Online Assignment Help
- Languages And Automata Assignment Help
- Haskell Assignment Help
- Economics And Computation Assignment Help
- ActionScript Assignment Help
- Audio Programming Assignment Help
- Bash Assignment Help
- Computer Graphics Assignment Help
- Groovy Assignment Help
- Kotlin Assignment Help
- Object Oriented Languages And Environments
- COBOL ASSIGNMENT HELP
- Bayesian Statistical Probabilistic Programming
- Computer Network Assignment Help
- Django Assignment Help
- Lambda Calculus Assignment Help
- Operating System Assignment Help
- Computational Learning Theory
- Delphi Assignment Help
- Concurrent Algorithms And Data Structures Assignment Help
- Machine Learning Assignment Help
- Human Computer Interface Assignment Help
- Foundations Of Data Networking Assignment Help
- Continuous Mathematics Assignment Help
- Compiler Assignment Help
- Computational Biology Assignment Help
- PostgreSQL Online Assignment Help
- Lua Assignment Help
- Human Computer Interaction Assignment Help
- Ethics And Responsible Innovation Assignment Help
- Communication And Ethical Issues In Computing
- Computer Science
- Combinatorial Optimisation Assignment Help
- Ethical Computing In Practice
- HTML Homework Assignment Help
- Linear Algebra Assignment Help
- Perl Assignment Help
- Artificial Intelligence Assignment Help
- Uncategorized
- Ethics And Professionalism Assignment Help
- Human Augmentics Assignment Help
- Linux Assignment Help
- PHP Assignment Help
- Assembly Language Assignment Help
- Dart Assignment Help
- Complete Python Bootcamp From Zero To Hero In Python Corrected Version
- Swift Assignment Help
- Computational Complexity Assignment Help
- Probability And Computing Assignment Help
- MATLAB Programming For Engineers
- Introduction To Statistical Learning
- Database Systems Implementation Assignment Help
- Computational Game Theory Assignment Help
- Database Assignment Help
- Probabilistic Model Checking Assignment Help
- Mathematics For Computer Science And Philosophy
- Introduction To Formal Proof Assignment Help
- Creative Coding Assignment Help
- Foundations Of Self-Programming Agents Assignment Help
- Machine Organization Assignment Help
- Software Design Assignment Help
- Data Communication And Networking Assignment Help
- Computational Biology
- Data Structure Assignment Help
- Foundations Of Software Engineering Assignment Help
- Mathematical Foundations Of Computing
- Principles Of Programming Languages Assignment Help
- Software Engineering Capstone Assignment Help
- Algorithms and Data Structures Assignment Help
No Comments