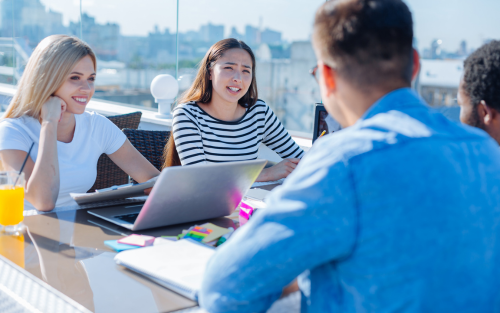
09 May File Handling In Python
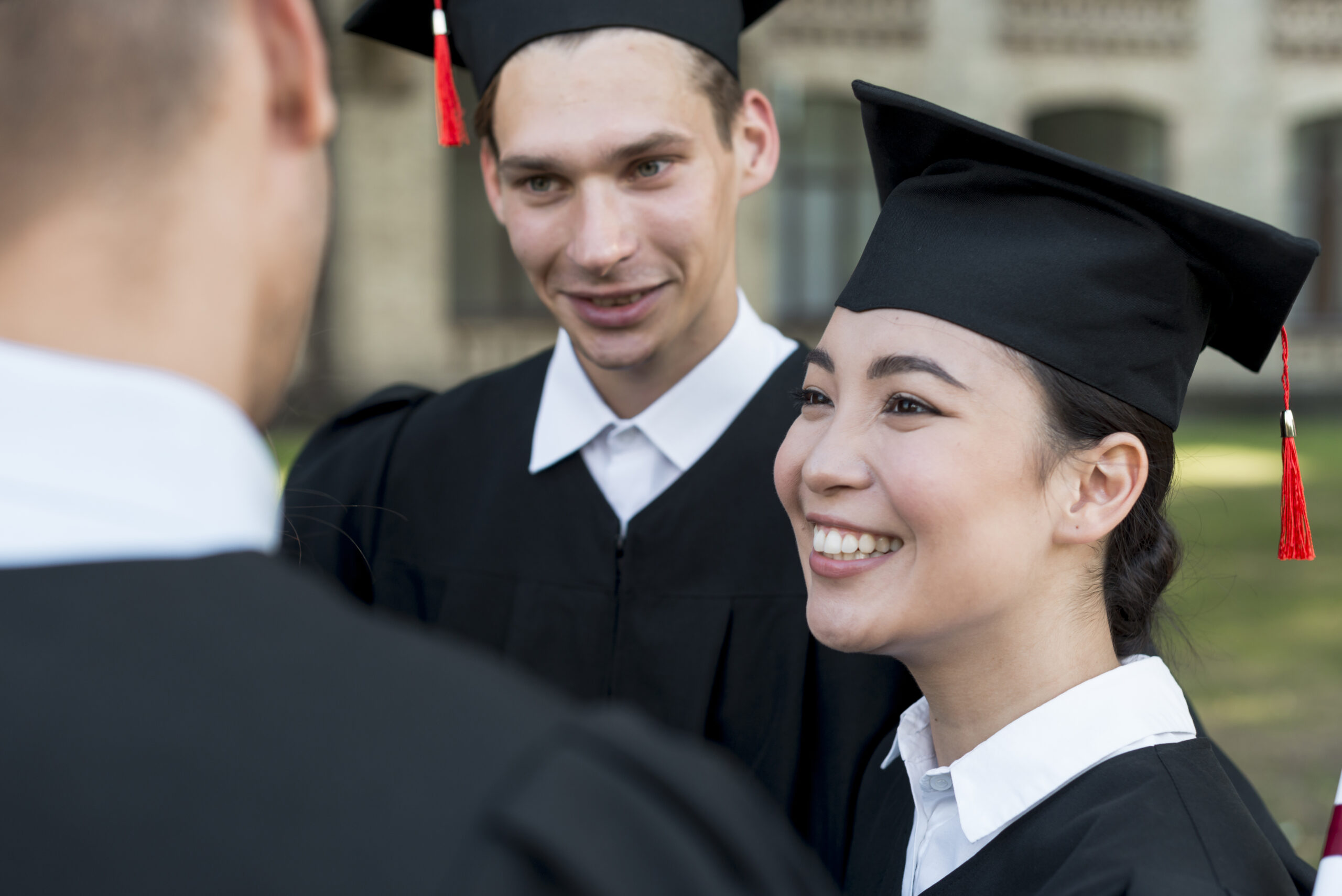
Introduction
File handling is an essential part of any programming language, including Python. Python provides a variety of functions and modules that make it easy to work with files. In this article, we’ll take a look at the basics of file handling in Python and explore some of the most commonly used functions and modules.
Master file handling in Python and efficiently manipulate files for data input/output operations. File handling in Python allows you to read from and write to files, process data, and perform various file-related operations. Learn how to open, read, write, and close files using Python’s built-in file handling functions and methods. Explore techniques for file navigation, directory operations, and file manipulation. Unlock the power of Python for file handling and efficiently manage your data files and file-based operations.
Opening and Closing Files
Before we can work with a file in Python, we need to open it. To open a file, we use the open()
function, which takes two arguments: the path to the file and the mode in which we want to open it. Here’s an example:
file = open("myfile.txt", "r")
In this example, we’re opening a file called myfile.txt
in read mode ("r"
). The open()
function returns a file object that we can use to read from or write to the file.
Once we’re done working with a file, we need to close it using the close()
method. Here’s an example:
file.close()
Reading from Files
To read from a file in Python, we can use the read()
method of the file object. Here’s an example:
file = open("myfile.txt", "r")
content = file.read()
print(content)
file.close()
In this example, we’re opening myfile.txt
in read mode, reading its contents using the read()
method, and printing the content to the console.
We can also read a file line by line using the readline()
method. Here’s an example:
file = open("myfile.txt", "r")
line = file.readline()
while line:
print(line)
line = file.readline()
file.close()
In this example, we’re opening myfile.txt
in read mode, reading its contents line by line using the readline()
method, and printing each line to the console.
Writing to Files
To write to a file in Python, we can use the write()
method of the file object. Here’s an example:
file = open("myfile.txt", "w")
file.write("Hello, World!")
file.close()
In this example, we’re opening myfile.txt
in write mode ("w"
) and writing the string "Hello, World!"
to the file using the write()
method.
We can also write to a file line by line using the writelines()
method. Here’s an example:
file = open("myfile.txt", "w")
lines = ["Line 1\n", "Line 2\n", "Line 3\n"]
file.writelines(lines)
file.close()
In this example, we’re opening myfile.txt
in write mode and writing a list of lines to the file using the writelines()
method.
Working with Binary Files
In addition to text files, Python can also work with binary files, such as images and videos. To work with binary files, we need to open them in binary mode ("b"
). Here’s an example:
file = open("myimage.jpg", "rb")
content = file.read()
file.close()
In this example, we’re opening a binary file called myimage.jpg
in read mode and reading its contents using the read()
method.
File Handling Modules
In addition to the built-in file handling functions, Python provides several modules that can make working with files even easier. Here are some of the most commonly used modules:
–os: Provides functions for working with files and directories, such as creating and deleting files, and changing file permissions.
-shutil
Provides functions for working with file archives, such as compressing
-glob
: Provides functions for working with file patterns, such as finding all files in a directory that match a certain pattern.
-csv
: Provides functions for working with CSV files, such as reading and writing data in CSV format.
-pickle
: Provides functions for working with Python objects, such as saving and loading objects to and from files.
Here are some examples of how to use these modules:
import os
# Create a new directory
os.mkdir(“mydir”)
# Delete a file
os.remove(“myfile.txt”)
# Rename a file
os.rename(“oldfile.txt”, “newfile.txt”)
In this example, we’re using the os
module to create a new directory, delete a file, and rename a file.
import shutil
# Compress a file
shutil.make_archive(“myarchive”, “zip”, “mydir”)
# Extract a file
shutil.unpack_archive(“myarchive.zip”, “mydir”)
In this example, we’re using the shutil
module to compress a directory into a ZIP file and extract the contents of a ZIP file into a directory.
import glob
# Find all text files in a directory
files = glob.glob(“*.txt”)
print(files)
In this example, we’re using the glob
module to find all files in the current directory that have a .txt
extension.
import csv
# Read data from a CSV file
with open(“mydata.csv”, “r”) as csvfile:
reader = csv.reader(csvfile)
for row in reader:
print(row)
# Write data to a CSV file
with open(“mydata.csv”, “w”) as csvfile:
writer = csv.writer(csvfile)
writer.writerow([“Name”, “Age”])
writer.writerow([“John”, 30])
writer.writerow([“Jane”, 25])
In this example, we’re using the csv
module to read data from a CSV file and write data to a CSV file.
import pickle
# Save an object to a file
myobj = {“name”: “John”, “age”: 30}
with open(“myobj.pickle”, “wb”) as file:
pickle.dump(myobj, file)
# Load an object from a file
with open(“myobj.pickle”, “rb”) as file:
myobj = pickle.load(file)
print(myobj)
In this example, we’re using the pickle
module to save a Python object to a file and load it from a file.
Case Study
Let’s consider a real-world scenario where file handling can be useful. Suppose you are working on a project where you need to store and analyze data from multiple sources. You have data coming in from CSV files, text files, and JSON files. You need to read the data from these files, perform some operations on it, and write the results to a new file.
In this case, Python’s file handling capabilities would be invaluable. You can use the csv
, json
, and os
modules to read and write data from these different file types. You can also use the os
module to create directories, move files, and delete files as needed.
Example
Let’s look at an example of how to read data from a text file and write it to a new file.
with open('input.txt', 'r') as file:
data = file.read()
with open(‘output.txt’, ‘w’) as file:
file.write(data)
In this example, we’re using the open
function to open a file in read mode, read the contents of the file into the data
variable, and then open another file in write mode and write the contents of data
to the file.
Example 2:
Let’s look at an example of how to read data from a CSV file and perform some calculations on it.
Suppose we have a CSV file that contains the following data:
Name, Age, Height
John, 30, 180
Jane, 25, 165
We want to calculate the average height of the people in the file. We can do this using the csv
module and the statistics
module.
import csv
import statistics
with open(‘data.csv’, ‘r’) as file:
reader = csv.reader(file)
next(reader) # skip the header row
heights = [int(row[2]) for row in reader]
average_height = statistics.mean(heights)
print(f’The average height is {average_height} cm.’)
In this example, we’re using the csv
module to read the data from the CSV file and the statistics
module to calculate the average height.
FAQs
Q: How do I check if a file exists in Python?
A: You can use the os.path.exists
function to check if a file exists. For example:
import os
if os.path.exists(‘myfile.txt’):
print(‘The file exists.’)
else:
print(‘The file does not exist.’)
Q: How do I delete a file in Python?
A: You can use the os.remove
function to delete a file. For example:
import os
os.remove(‘myfile.txt’)
Q: How do I create a directory in Python?
A: You can use the os.mkdir
function to create a directory. For example:
import os
os.mkdir(‘mydir’)
Q: How do I list all files in a directory in Python?
A: You can use the os.listdir
function to list all files in a directory. For example:
import os
files = os.listdir(‘.’)
print(files)
Conclusion
In conclusion, file handling is an essential part of any programming language, and Python provides a rich set of file handling capabilities. The open
function is used to open files in different modes, and we can use various modules like csv
, json
, and os
to read and write data to different file types. We’ve also explored some advanced topics like file handling modes, file system navigation, and file permissions.
Python’s file handling capabilities are particularly useful in data science and machine learning projects, where data is often stored in different formats like CSV, JSON, and XML. With Python’s file handling capabilities, you can easily read, manipulate, and analyze data from these different sources.
In summary, mastering file handling in Python is crucial for any developer who needs to work with files and data in their projects. It is an essential skill that will enable you to handle data more efficiently, automate repetitive tasks, and build powerful applications.
Latest Topic
-
Cloud-Native Technologies: Best Practices
20 April, 2024 -
Generative AI with Llama 3: Shaping the Future
15 April, 2024 -
Mastering Llama 3: The Ultimate Guide
10 April, 2024
Category
- Assignment Help
- Homework Help
- Programming
- Trending Topics
- C Programming Assignment Help
- Art, Interactive, And Robotics
- Networked Operating Systems Programming
- Knowledge Representation & Reasoning Assignment Help
- Digital Systems Assignment Help
- Computer Design Assignment Help
- Artificial Life And Digital Evolution
- Coding and Fundamentals: Working With Collections
- UML Online Assignment Help
- Prolog Online Assignment Help
- Natural Language Processing Assignment Help
- Julia Assignment Help
- Golang Assignment Help
- Design Implementation Of Network Protocols
- Computer Architecture Assignment Help
- Object-Oriented Languages And Environments
- Coding Early Object and Algorithms: Java Coding Fundamentals
- Deep Learning In Healthcare Assignment Help
- Geometric Deep Learning Assignment Help
- Models Of Computation Assignment Help
- Systems Performance And Concurrent Computing
- Advanced Security Assignment Help
- Typescript Assignment Help
- Computational Media Assignment Help
- Design And Analysis Of Algorithms
- Geometric Modelling Assignment Help
- JavaScript Assignment Help
- MySQL Online Assignment Help
- Programming Practicum Assignment Help
- Public Policy, Legal, And Ethical Issues In Computing, Privacy, And Security
- Computer Vision
- Advanced Complexity Theory Assignment Help
- Big Data Mining Assignment Help
- Parallel Computing And Distributed Computing
- Law And Computer Science Assignment Help
- Engineering Distributed Objects For Cloud Computing
- Building Secure Computer Systems Assignment Help
- Ada Assignment Help
- R Programming Assignment Help
- Oracle Online Assignment Help
- Languages And Automata Assignment Help
- Haskell Assignment Help
- Economics And Computation Assignment Help
- ActionScript Assignment Help
- Audio Programming Assignment Help
- Bash Assignment Help
- Computer Graphics Assignment Help
- Groovy Assignment Help
- Kotlin Assignment Help
- Object Oriented Languages And Environments
- COBOL ASSIGNMENT HELP
- Bayesian Statistical Probabilistic Programming
- Computer Network Assignment Help
- Django Assignment Help
- Lambda Calculus Assignment Help
- Operating System Assignment Help
- Computational Learning Theory
- Delphi Assignment Help
- Concurrent Algorithms And Data Structures Assignment Help
- Machine Learning Assignment Help
- Human Computer Interface Assignment Help
- Foundations Of Data Networking Assignment Help
- Continuous Mathematics Assignment Help
- Compiler Assignment Help
- Computational Biology Assignment Help
- PostgreSQL Online Assignment Help
- Lua Assignment Help
- Human Computer Interaction Assignment Help
- Ethics And Responsible Innovation Assignment Help
- Communication And Ethical Issues In Computing
- Computer Science
- Combinatorial Optimisation Assignment Help
- Ethical Computing In Practice
- HTML Homework Assignment Help
- Linear Algebra Assignment Help
- Perl Assignment Help
- Artificial Intelligence Assignment Help
- Uncategorized
- Ethics And Professionalism Assignment Help
- Human Augmentics Assignment Help
- Linux Assignment Help
- PHP Assignment Help
- Assembly Language Assignment Help
- Dart Assignment Help
- Complete Python Bootcamp From Zero To Hero In Python Corrected Version
- Swift Assignment Help
- Computational Complexity Assignment Help
- Probability And Computing Assignment Help
- MATLAB Programming For Engineers
- Introduction To Statistical Learning
- Database Systems Implementation Assignment Help
- Computational Game Theory Assignment Help
- Database Assignment Help
- Probabilistic Model Checking Assignment Help
- Mathematics For Computer Science And Philosophy
- Introduction To Formal Proof Assignment Help
- Creative Coding Assignment Help
- Foundations Of Self-Programming Agents Assignment Help
- Machine Organization Assignment Help
- Software Design Assignment Help
- Data Communication And Networking Assignment Help
- Computational Biology
- Data Structure Assignment Help
- Foundations Of Software Engineering Assignment Help
- Mathematical Foundations Of Computing
- Principles Of Programming Languages Assignment Help
- Software Engineering Capstone Assignment Help
- Algorithms and Data Structures Assignment Help
No Comments