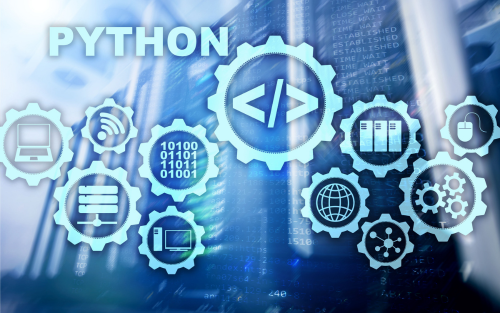
18 Apr How Do I Work With Databases In Python?
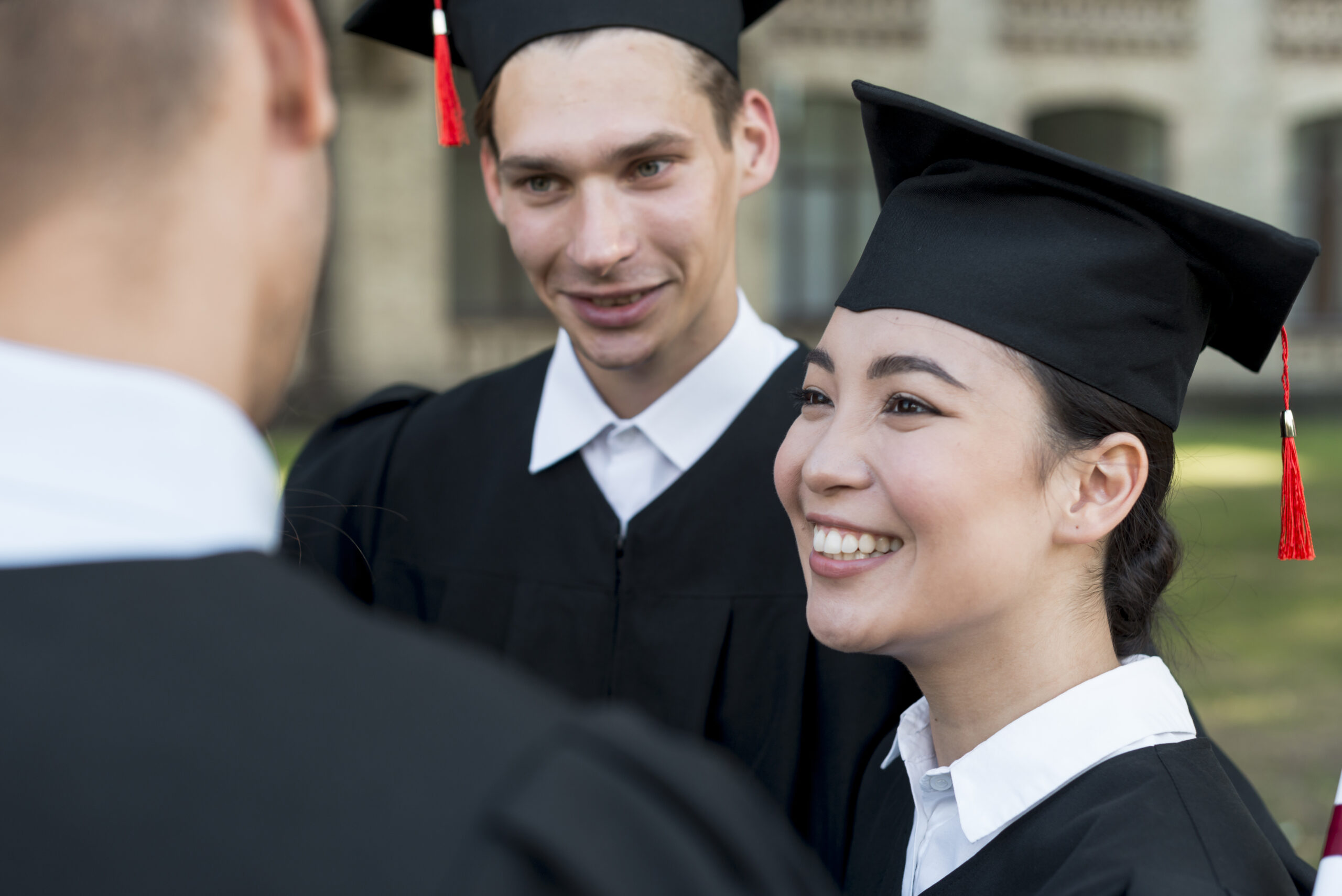
Python provides a wide range of libraries and modules for working with databases. In this article, we will discuss how to work with databases in Python using some popular libraries.
- SQLite3
SQLite3 is a built-in module in Python that provides a simple way to work with SQLite databases. SQLite is a lightweight database that is widely used for small to medium-sized applications. To work with SQLite3, you need to import the sqlite3
module and create a connection to the database using the connect()
method. Once you have a connection, you can execute SQL commands using the execute()
method.
import sqlite3
# create a connection to the database
conn = sqlite3.connect('example.db')
# create a cursor object
cursor = conn.cursor()
# execute a query
cursor.execute("CREATE TABLE users (id INTEGER PRIMARY KEY, name TEXT, email TEXT)")
# commit the changes
conn.commit()
# close the connection
conn.close()
- MySQL
MySQL is a popular open-source relational database management system. To work with MySQL databases in Python, you can use the mysql-connector-python
module. First, you need to install the module using pip. Once you have installed the module, you can create a connection to the database using the connect()
method.
import mysql.connector
# create a connection to the database
conn = mysql.connector.connect(
host="localhost",
user="username",
password="password",
database="database_name"
)
# create a cursor object
cursor = conn.cursor()
# execute a query
cursor.execute("CREATE TABLE users (id INT AUTO_INCREMENT PRIMARY KEY, name VARCHAR(255), email VARCHAR(255))")
# commit the changes
conn.commit()
# close the connection
conn.close()
- PostgreSQL PostgreSQL is a powerful open-source relational database management system. To work with PostgreSQL databases in Python, you can use the
psycopg2
module. First, you need to install the module using pip. Once you have installed the module, you can create a connection to the database using theconnect()
method.
import psycopg2
# create a connection to the database
conn = psycopg2.connect(
host="localhost",
user="username",
password="password",
database="database_name"
)
# create a cursor object
cursor = conn.cursor()
# execute a query
cursor.execute("CREATE TABLE users (id SERIAL PRIMARY KEY, name VARCHAR(255), email VARCHAR(255))")
# commit the changes
conn.commit()
# close the connection
conn.close()
- SQLAlchemy SQLAlchemy is a popular Python library for working with relational databases. SQLAlchemy provides a high-level API for interacting with databases, allowing you to write database-agnostic code. SQLAlchemy supports a wide range of databases, including MySQL, PostgreSQL, SQLite, and Oracle. Here is an example of how to use SQLAlchemy to create a table in a MySQL database:
from sqlalchemy import create_engine, Column, Integer, String
from sqlalchemy.ext.declarative import declarative_base
# create an engine to connect to the database
engine = create_engine('mysql+mysqlconnector://username:password@localhost:3306/database_name')
# create a base class
Base = declarative_base()
# create a class for the users table
class User(Base):
__tablename__ = 'users'
id = Column(Integer, primary_key=True)
name = Column(String(255))
email = Column(String(255))
# create the table
Base.metadata.create_all(engine)
Conclusion
Python provides a wide range of libraries and modules for working with databases. By mastering these libraries, you can become a more effective data analyst or developer and work
with different types of databases. SQLite3 is a simple and lightweight database that is built into Python. MySQL is a popular open-source relational database that can be accessed using the mysql-connector-python module. PostgreSQL is a powerful open-source relational database that can be accessed using the psycopg2 module. SQLAlchemy is a high-level API for interacting with relational databases that supports a wide range of databases, including MySQL, PostgreSQL, SQLite, and Oracle.
When working with databases in Python, it is important to follow best practices to ensure that your code is secure and efficient. Here are some best practices to keep in mind:
Always use parameterized queries to avoid SQL injection attacks.
Close your database connections when you are finished with them to avoid resource leaks.
Use transactions when working with databases to ensure data integrity and consistency.
Use indexes to optimize query performance.
Use an ORM (Object-Relational Mapping) tool like SQLAlchemy to simplify database interactions and reduce the risk of errors.
By following these best practices, you can create robust, secure, and efficient Python applications that work seamlessly with databases.
Latest Topic
-
Cloud-Native Technologies: Best Practices
20 April, 2024 -
Generative AI with Llama 3: Shaping the Future
15 April, 2024 -
Mastering Llama 3: The Ultimate Guide
10 April, 2024
Category
- Assignment Help
- Homework Help
- Programming
- Trending Topics
- C Programming Assignment Help
- Art, Interactive, And Robotics
- Networked Operating Systems Programming
- Knowledge Representation & Reasoning Assignment Help
- Digital Systems Assignment Help
- Computer Design Assignment Help
- Artificial Life And Digital Evolution
- Coding and Fundamentals: Working With Collections
- UML Online Assignment Help
- Prolog Online Assignment Help
- Natural Language Processing Assignment Help
- Julia Assignment Help
- Golang Assignment Help
- Design Implementation Of Network Protocols
- Computer Architecture Assignment Help
- Object-Oriented Languages And Environments
- Coding Early Object and Algorithms: Java Coding Fundamentals
- Deep Learning In Healthcare Assignment Help
- Geometric Deep Learning Assignment Help
- Models Of Computation Assignment Help
- Systems Performance And Concurrent Computing
- Advanced Security Assignment Help
- Typescript Assignment Help
- Computational Media Assignment Help
- Design And Analysis Of Algorithms
- Geometric Modelling Assignment Help
- JavaScript Assignment Help
- MySQL Online Assignment Help
- Programming Practicum Assignment Help
- Public Policy, Legal, And Ethical Issues In Computing, Privacy, And Security
- Computer Vision
- Advanced Complexity Theory Assignment Help
- Big Data Mining Assignment Help
- Parallel Computing And Distributed Computing
- Law And Computer Science Assignment Help
- Engineering Distributed Objects For Cloud Computing
- Building Secure Computer Systems Assignment Help
- Ada Assignment Help
- R Programming Assignment Help
- Oracle Online Assignment Help
- Languages And Automata Assignment Help
- Haskell Assignment Help
- Economics And Computation Assignment Help
- ActionScript Assignment Help
- Audio Programming Assignment Help
- Bash Assignment Help
- Computer Graphics Assignment Help
- Groovy Assignment Help
- Kotlin Assignment Help
- Object Oriented Languages And Environments
- COBOL ASSIGNMENT HELP
- Bayesian Statistical Probabilistic Programming
- Computer Network Assignment Help
- Django Assignment Help
- Lambda Calculus Assignment Help
- Operating System Assignment Help
- Computational Learning Theory
- Delphi Assignment Help
- Concurrent Algorithms And Data Structures Assignment Help
- Machine Learning Assignment Help
- Human Computer Interface Assignment Help
- Foundations Of Data Networking Assignment Help
- Continuous Mathematics Assignment Help
- Compiler Assignment Help
- Computational Biology Assignment Help
- PostgreSQL Online Assignment Help
- Lua Assignment Help
- Human Computer Interaction Assignment Help
- Ethics And Responsible Innovation Assignment Help
- Communication And Ethical Issues In Computing
- Computer Science
- Combinatorial Optimisation Assignment Help
- Ethical Computing In Practice
- HTML Homework Assignment Help
- Linear Algebra Assignment Help
- Perl Assignment Help
- Artificial Intelligence Assignment Help
- Uncategorized
- Ethics And Professionalism Assignment Help
- Human Augmentics Assignment Help
- Linux Assignment Help
- PHP Assignment Help
- Assembly Language Assignment Help
- Dart Assignment Help
- Complete Python Bootcamp From Zero To Hero In Python Corrected Version
- Swift Assignment Help
- Computational Complexity Assignment Help
- Probability And Computing Assignment Help
- MATLAB Programming For Engineers
- Introduction To Statistical Learning
- Database Systems Implementation Assignment Help
- Computational Game Theory Assignment Help
- Database Assignment Help
- Probabilistic Model Checking Assignment Help
- Mathematics For Computer Science And Philosophy
- Introduction To Formal Proof Assignment Help
- Creative Coding Assignment Help
- Foundations Of Self-Programming Agents Assignment Help
- Machine Organization Assignment Help
- Software Design Assignment Help
- Data Communication And Networking Assignment Help
- Computational Biology
- Data Structure Assignment Help
- Foundations Of Software Engineering Assignment Help
- Mathematical Foundations Of Computing
- Principles Of Programming Languages Assignment Help
- Software Engineering Capstone Assignment Help
- Algorithms and Data Structures Assignment Help
No Comments