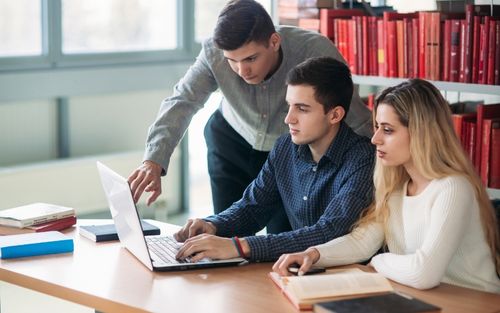
26 Apr How To Build A Full-Stack Web Application With Flask And Vue.Js
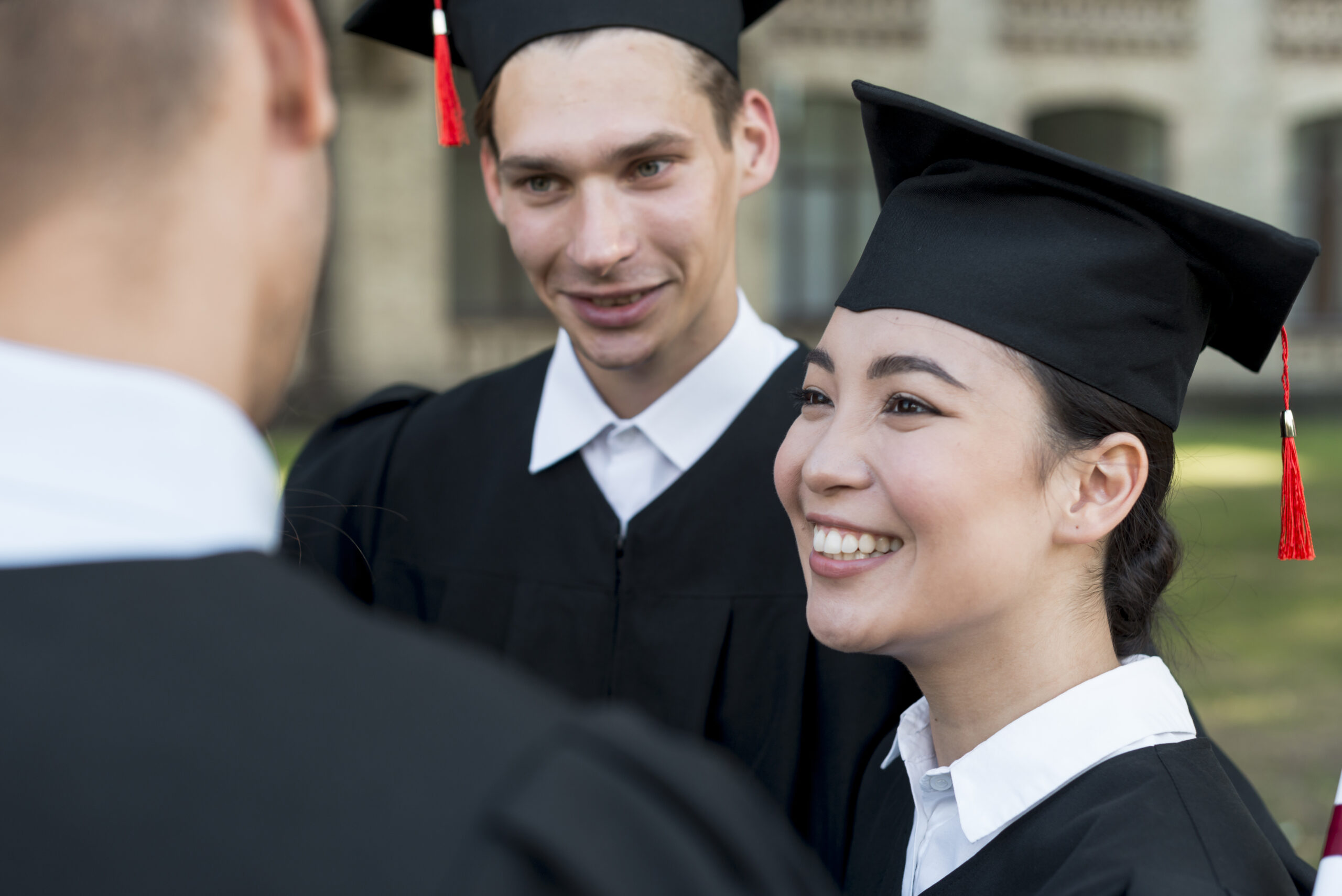
How To Build A Full-Stack Web Application With Flask And Vue.Js
If you’re looking to build a full-stack web application with Python, Flask and Vue.js, you’re in luck! This tutorial will walk you through the entire process step-by-step, from setting up your development environment to deploying your application.
Before we dive into the details of building a full-stack web application with Flask and Vue.js, let’s first define what each of these technologies are and how they work together.
Flask is a micro web framework written in Python. It is lightweight and provides the bare minimum for building web applications. Flask allows developers to focus on writing clean and efficient code, without the added overhead of a full-fledged framework.
Vue.js, on the other hand, is a progressive JavaScript framework for building user interfaces. It is designed to be easy to use and flexible, making it a great choice for building dynamic and interactive web applications.
Together, Flask and Vue.js make a powerful combination for building full-stack web applications. Flask provides the back-end infrastructure for the application, while Vue.js handles the front-end user interface.
Now, let’s get started with building our full-stack web application with Flask and Vue.js.
Setting Up Your Development Environment
Before you can start building your full-stack web application with Flask and Vue.js, you need to set up your development environment. Here are the steps you need to follow to do that:
Install Python
The first step is to install Python. You can download the latest version of Python from the official Python website.
Install Flask
Once you have Python installed, you need to install Flask. You can do this by running the following command in your terminal:
pip install Flask
Install Vue.js
Next, you need to install Vue.js. You can do this by including the Vue.js script in your HTML file, or by using a package manager like npm or Yarn.
Install Flask-CORS
Finally, you need to install Flask-CORS. This package allows for cross-origin resource sharing (CORS), which is necessary when working with Vue.js.
You can install Flask-CORS by running the following command in your terminal:
pip install -U flask-cors
Once you have your development environment set up, you can start building your full-stack web application with Flask and Vue.js.
Building the Back-End with Flask
The first step in building our full-stack web application is to create the back-end with Flask. Here’s how to do it:
Create a Flask App
The first step is to create a Flask app. You can do this by creating a new Python file and importing the Flask module:
from flask import Flask
app = Flask(__name__)
Create Endpoints
Next, you need to create endpoints for your Flask app. These endpoints will handle incoming requests and return responses.
Here’s an example of how to create an endpoint that returns a simple message:
@app.route('/')
def hello_world():
return 'Hello, World!'
Run the Flask App
Finally, you need to run the Flask app. You can do this by adding the following code to the end of your Python file:
if __name__ == '__main__':
app.run(debug=True)
This will start the Flask app and allow you to access it in your web browser at http://localhost:5000.
Building the Front-End with Vue.js
Vue.js is a popular front-end JavaScript framework used for building user interfaces. In this section, we will build the front-end of our full-stack web application using Vue.js.
Installing Vue.js
To get started with Vue.js, we need to install it. We can do this using npm, the Node.js package manager.
First, we need to create a new directory for our Vue.js application. We can do this using the following command:
mkdir my-vue-app
cd my-vue-app
Next, we can initialize a new npm project using the following command:
npm init -y
This will create a package.json
file in our directory. Now, we can install Vue.js by running the following command:
npm install vue
Creating the Vue.js Component
In Vue.js, we create components to build our user interfaces. A component is a reusable piece of code that contains HTML, CSS, and JavaScript. In this section, we will create a simple Vue.js component.
Create a new file called app.vue
in the src
directory of our Vue.js application. In this file, we will define our component:
<template>
<div>
<h1>Hello, World!</h1>
</div>
</template>
Here, we have defined a template for our component. The template contains a single div
element with an h1
element inside.
We also need to define the JavaScript logic for our component. Add the following script tag to app.vue
:
<script>
export default {
name: 'app',
}
</script>
This defines a new Vue.js component called app
. We have also defined the name
property for our component.
Rendering the Vue.js Component
Now that we have defined our Vue.js component, we need to render it in our web application. To do this, we will create a new file called main.js
in the src
directory of our Vue.js application.
In main.js
, we will import Vue.js and our app.vue
component:
import Vue from 'vue'
import App from './App.vue'
Next, we will create a new Vue.js instance and tell it to render our app.vue
component:
new Vue({
render: h => h(App),
}).$mount('#app')
This tells Vue.js to render our App
component and mount it to the #app
element in our HTML file.
Styling the Vue.js Component
We can style our Vue.js component using CSS. To do this, we can add a style
tag to app.vue
:
<style scoped>
h1 {
color: red;
}
</style>
This will style the h1
element in our component with a red color.
Adding the Vue.js Component to the Flask Application
Now that we have created our Vue.js component, we need to add it to our Flask application. We can do this by serving the Vue.js application as a static asset.
In app.py
, add the following route:
@app.route('/')
def index():
return render_template('index.html')
This will render the index.html
template when a user visits the root URL of our web application.
Next, create a new file called index.html
in the templates
directory of our Flask application. Add the following HTML code to index.html
:
<!DOCTYPE html>
<html>
<head>
<title>My Vue.js App</title>
</head>
<body>
<div
Conclusion
In conclusion, building a full-stack web application with Flask and Vue.js can be a rewarding experience for both beginners and experienced developers. The combination of Flask’s flexibility and Vue.js’s reactivity can lead to the creation of powerful and efficient applications that can handle complex data structures and user interactions.
In this blog, we went through the step-by-step process of building a full-stack web application with Flask and Vue.js. We first started by setting up the backend with Flask, defining the data models, and creating the routes to handle HTTP requests. We then moved on to the front-end, where we set up Vue.js, created the necessary components, and used Vuex to manage the state of the application.
Finally, we covered how to deploy the application to a hosting service, making it available to the public. While there are many other aspects to consider when building a full-stack application, this guide should give you a good starting point for your own projects.
Remember, building a full-stack web application requires patience, attention to detail, and a willingness to learn new technologies. By following best practices and keeping an open mind, you can create powerful and efficient applications that meet the needs of your users.
Latest Topic
-
Cloud-Native Technologies: Best Practices
20 April, 2024 -
Generative AI with Llama 3: Shaping the Future
15 April, 2024 -
Mastering Llama 3: The Ultimate Guide
10 April, 2024
Category
- Assignment Help
- Homework Help
- Programming
- Trending Topics
- C Programming Assignment Help
- Art, Interactive, And Robotics
- Networked Operating Systems Programming
- Knowledge Representation & Reasoning Assignment Help
- Digital Systems Assignment Help
- Computer Design Assignment Help
- Artificial Life And Digital Evolution
- Coding and Fundamentals: Working With Collections
- UML Online Assignment Help
- Prolog Online Assignment Help
- Natural Language Processing Assignment Help
- Julia Assignment Help
- Golang Assignment Help
- Design Implementation Of Network Protocols
- Computer Architecture Assignment Help
- Object-Oriented Languages And Environments
- Coding Early Object and Algorithms: Java Coding Fundamentals
- Deep Learning In Healthcare Assignment Help
- Geometric Deep Learning Assignment Help
- Models Of Computation Assignment Help
- Systems Performance And Concurrent Computing
- Advanced Security Assignment Help
- Typescript Assignment Help
- Computational Media Assignment Help
- Design And Analysis Of Algorithms
- Geometric Modelling Assignment Help
- JavaScript Assignment Help
- MySQL Online Assignment Help
- Programming Practicum Assignment Help
- Public Policy, Legal, And Ethical Issues In Computing, Privacy, And Security
- Computer Vision
- Advanced Complexity Theory Assignment Help
- Big Data Mining Assignment Help
- Parallel Computing And Distributed Computing
- Law And Computer Science Assignment Help
- Engineering Distributed Objects For Cloud Computing
- Building Secure Computer Systems Assignment Help
- Ada Assignment Help
- R Programming Assignment Help
- Oracle Online Assignment Help
- Languages And Automata Assignment Help
- Haskell Assignment Help
- Economics And Computation Assignment Help
- ActionScript Assignment Help
- Audio Programming Assignment Help
- Bash Assignment Help
- Computer Graphics Assignment Help
- Groovy Assignment Help
- Kotlin Assignment Help
- Object Oriented Languages And Environments
- COBOL ASSIGNMENT HELP
- Bayesian Statistical Probabilistic Programming
- Computer Network Assignment Help
- Django Assignment Help
- Lambda Calculus Assignment Help
- Operating System Assignment Help
- Computational Learning Theory
- Delphi Assignment Help
- Concurrent Algorithms And Data Structures Assignment Help
- Machine Learning Assignment Help
- Human Computer Interface Assignment Help
- Foundations Of Data Networking Assignment Help
- Continuous Mathematics Assignment Help
- Compiler Assignment Help
- Computational Biology Assignment Help
- PostgreSQL Online Assignment Help
- Lua Assignment Help
- Human Computer Interaction Assignment Help
- Ethics And Responsible Innovation Assignment Help
- Communication And Ethical Issues In Computing
- Computer Science
- Combinatorial Optimisation Assignment Help
- Ethical Computing In Practice
- HTML Homework Assignment Help
- Linear Algebra Assignment Help
- Perl Assignment Help
- Artificial Intelligence Assignment Help
- Uncategorized
- Ethics And Professionalism Assignment Help
- Human Augmentics Assignment Help
- Linux Assignment Help
- PHP Assignment Help
- Assembly Language Assignment Help
- Dart Assignment Help
- Complete Python Bootcamp From Zero To Hero In Python Corrected Version
- Swift Assignment Help
- Computational Complexity Assignment Help
- Probability And Computing Assignment Help
- MATLAB Programming For Engineers
- Introduction To Statistical Learning
- Database Systems Implementation Assignment Help
- Computational Game Theory Assignment Help
- Database Assignment Help
- Probabilistic Model Checking Assignment Help
- Mathematics For Computer Science And Philosophy
- Introduction To Formal Proof Assignment Help
- Creative Coding Assignment Help
- Foundations Of Self-Programming Agents Assignment Help
- Machine Organization Assignment Help
- Software Design Assignment Help
- Data Communication And Networking Assignment Help
- Computational Biology
- Data Structure Assignment Help
- Foundations Of Software Engineering Assignment Help
- Mathematical Foundations Of Computing
- Principles Of Programming Languages Assignment Help
- Software Engineering Capstone Assignment Help
- Algorithms and Data Structures Assignment Help
No Comments