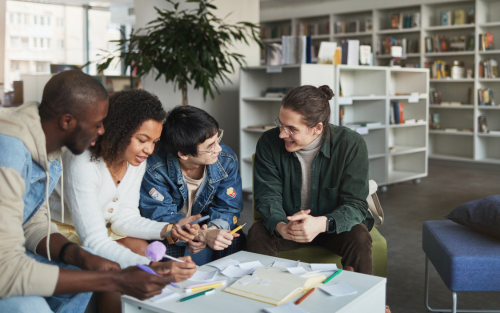
26 Apr How To Build A Simple CRUD App With Laravel
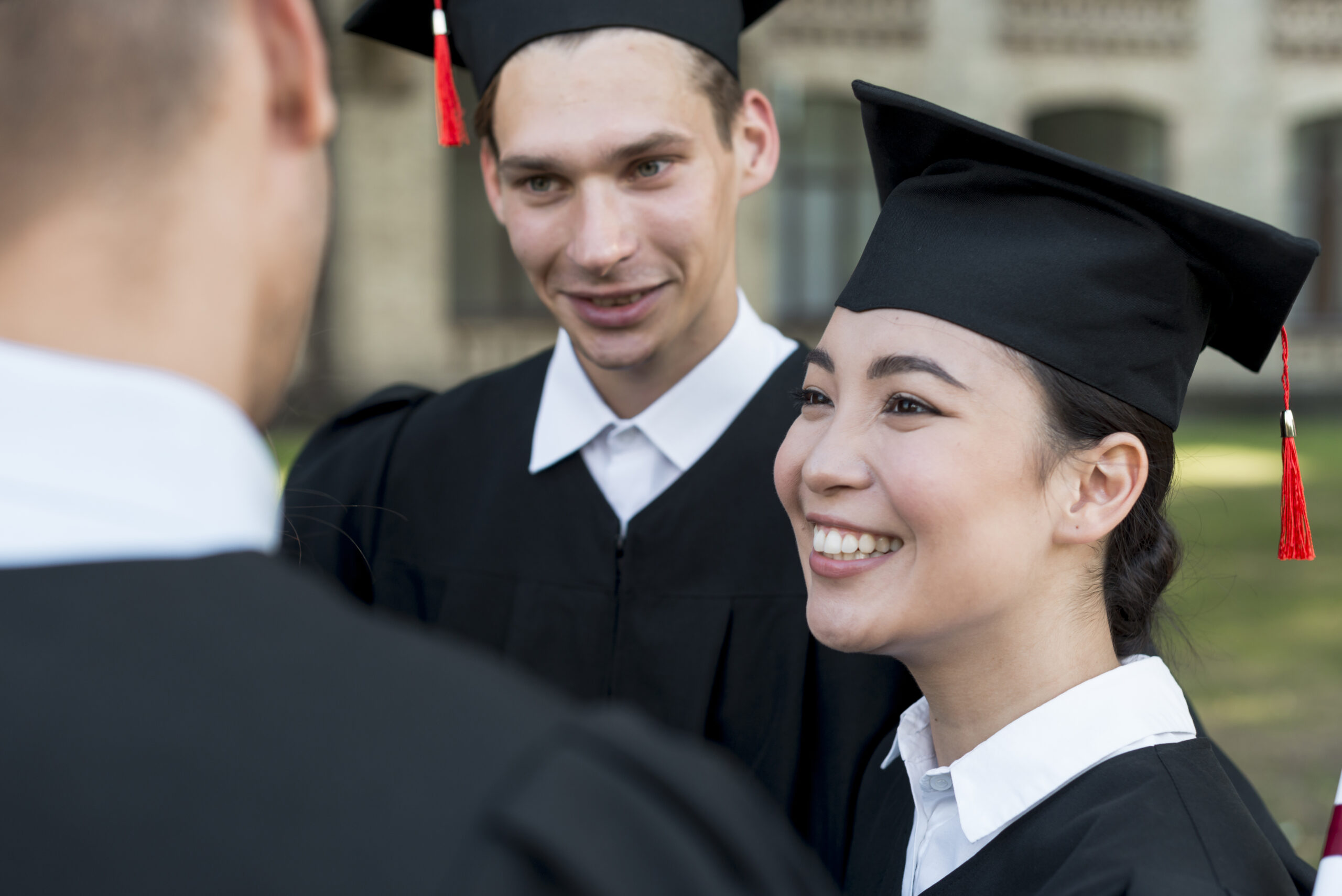
Laravel is a popular PHP web framework that makes it easy to build web applications. It offers a variety of features that make development faster and easier, including routing, middleware, Eloquent ORM, Blade templating engine, and more.
In this article, we’ll walk through the process of building a simple CRUD (Create, Read, Update, Delete) app with Laravel. We’ll start with the basics of setting up a new Laravel project and then move on to creating the database and building the CRUD functionality.
Prerequisites Before we start, make sure you have the following software installed on your computer:
PHP 7.4 or later
Composer
MySQL or any other database management system
Getting Started To create a new Laravel project, open your terminal and navigate to the directory where you want to create the project. Then, run the following command:
composer create-project --prefer-dist laravel/laravel crud-app
This will create a new Laravel project called crud-app in the current directory.
Next, navigate into the project directory and start the development server by running the following command:
php artisan serve
This will start the development server and your Laravel app will be accessible at http://localhost:8000.
Creating the Database Next, let’s create a new database for our app. Open your MySQL client and run the following commands:
CREATE DATABASE crud_app;
This will create a new database called crud_app. Next, let’s create a new table in the database called users. Run the following command:
CREATE TABLE users (
id INT UNSIGNED AUTO_INCREMENT PRIMARY KEY,
name VARCHAR(255) NOT NULL,
email VARCHAR(255) NOT NULL,
password VARCHAR(255) NOT NULL,
created_at TIMESTAMP DEFAULT CURRENT_TIMESTAMP,
updated_at TIMESTAMP DEFAULT CURRENT_TIMESTAMP ON UPDATE CURRENT_TIMESTAMP
);
This will create a new table called users with the following columns:
id: the primary key of the table
name: the name of the user
email: the email address of the user
password: the password of the user
created_at: the timestamp when the user was created
updated_at: the timestamp when the user was last updated
Building the CRUD Functionality
Now that we have the database set up, let’s build the CRUD functionality for our app.
First, let’s create a new controller for the users. Run the following command:
php artisan make:controller UserController --resource
This will create a new controller called UserController with all the necessary methods for CRUD operations.
Next, let’s define the routes for our app. Open the routes/web.php file and add the following code:
Route::resource('users', 'UserController');
This will define the routes for our UserController.
Now, let’s create the views for our app. Create a new folder called users inside the resources/views folder. Inside this folder, create four new Blade templates:
index.blade.php: the view for displaying a list of all users
create.blade.php: the view for creating a new user
edit.blade.php: the view for editing an existing user
show.blade.php: the view for displaying the details of a user
In each of these templates, add the necessary HTML and form elements for displaying and editing the user data.
Finally, let’s implement the CRUD functionality in our UserController. Open the app/Http/Controllers/UserController.php file and add the following code:
public function index()
{
$users = DB::table('users')->get();
return view('users.index', compact('users'));
}
public function create()
{
return view('users.create');
}
public function store(Request
Create a Migration
A migration is a database schema created to manage the database tables and their columns. Laravel provides an easy way to create and manage migrations.
To create a migration, run the following command:
php artisan make:migration create_tasks_table --create=tasks
This command will create a migration file named create_tasks_table
in the database/migrations
directory. The --create
option specifies the name of the table to be created.
Open the create_tasks_table
file and add the following code:
<?php
use Illuminate\Database\Migrations\Migration;
use Illuminate\Database\Schema\Blueprint;
use Illuminate\Support\Facades\Schema;
class CreateTasksTable extends Migration{
/**
* Run the migrations.
*
* @return void
*/
public function up()
{
Schema::create('tasks', function (Blueprint $table) {
$table->id();
$table->string('title');
$table->text('description');
$table->boolean('completed')->default(false);
$table->timestamps();
});
}
/**
* Reverse the migrations.
*
* @return void
*/
public function down()
{
Schema::dropIfExists('tasks');
}
}
In the up
method, we are creating the tasks
table with four columns: id
, title
, description
, and completed
. The id
column is an auto-incrementing primary key. The timestamps
method creates two columns: created_at
and updated_at
.
In the down
method, we are dropping the tasks
table if it exists.
Run Migrations
To run the migration and create the tasks
table in the database, run the following command:
php artisan migrate
You should see a message like the following:
Migrating: 2019_01_01_000000_create_tasks_table
Migrated: 2019_01_01_000000_create_tasks_table
This means that the migration was successful and the tasks
table was created in the database.
Create a Task Model
Now that we have created the tasks
table in the database, we need to create a corresponding model to interact with the table.
To create a model, run the following command:
php artisan make:model Task
This command will create a model file named Task.php
in the app/Models
directory.
Open the Task.php
file and add the following code:
<?php
namespace App\Models;
use Illuminate\Database\Eloquent\Factories\HasFactory;
use Illuminate\Database\Eloquent\Model;
class Task extends Model{
use HasFactory;
protected $fillable = ['title', 'description', 'completed'];
}
We have defined the Task
model class and specified the table name tasks
in the $table
property. We have also defined the fillable attributes: title
, description
, and completed
.
Create Routes
We need to create routes to handle HTTP requests to our application. Open the web.php
file located in the routes
directory and add the following code:
<?php
use Illuminate\Support\Facades\Route;
use App\Http\Controllers\TaskController;
Route::get('/', [TaskController::class, 'index'])->name('tasks.index');
Route::get('/tasks/create', [TaskController::class, 'create'])->name('tasks.create');
Route::post('/tasks', [TaskController::class, 'store'])->name('tasks.store');
Route::get('/tasks/{task}', [TaskController::class, 'show'])->name
Conclusion
In conclusion, Laravel provides a great framework for building robust CRUD applications quickly and easily. It offers a lot of useful features and tools that simplify the development process and allow developers to focus on creating great applications.
By following the steps outlined in this article, you should now have a good understanding of how to build a simple CRUD app with Laravel. You should also be familiar with some of the key concepts and features of Laravel, such as routing, controllers, models, and views.
Of course, there is still a lot more to learn about Laravel and web development in general. However, by building simple CRUD apps like this one and gradually adding more features and complexity, you can gradually build your skills and knowledge.
If you have any questions or comments about this article or about Laravel in general, please feel free to leave a comment below. Good luck with your Laravel development projects!
Latest Topic
-
Cloud-Native Technologies: Best Practices
20 April, 2024 -
Generative AI with Llama 3: Shaping the Future
15 April, 2024 -
Mastering Llama 3: The Ultimate Guide
10 April, 2024
Category
- Assignment Help
- Homework Help
- Programming
- Trending Topics
- C Programming Assignment Help
- Art, Interactive, And Robotics
- Networked Operating Systems Programming
- Knowledge Representation & Reasoning Assignment Help
- Digital Systems Assignment Help
- Computer Design Assignment Help
- Artificial Life And Digital Evolution
- Coding and Fundamentals: Working With Collections
- UML Online Assignment Help
- Prolog Online Assignment Help
- Natural Language Processing Assignment Help
- Julia Assignment Help
- Golang Assignment Help
- Design Implementation Of Network Protocols
- Computer Architecture Assignment Help
- Object-Oriented Languages And Environments
- Coding Early Object and Algorithms: Java Coding Fundamentals
- Deep Learning In Healthcare Assignment Help
- Geometric Deep Learning Assignment Help
- Models Of Computation Assignment Help
- Systems Performance And Concurrent Computing
- Advanced Security Assignment Help
- Typescript Assignment Help
- Computational Media Assignment Help
- Design And Analysis Of Algorithms
- Geometric Modelling Assignment Help
- JavaScript Assignment Help
- MySQL Online Assignment Help
- Programming Practicum Assignment Help
- Public Policy, Legal, And Ethical Issues In Computing, Privacy, And Security
- Computer Vision
- Advanced Complexity Theory Assignment Help
- Big Data Mining Assignment Help
- Parallel Computing And Distributed Computing
- Law And Computer Science Assignment Help
- Engineering Distributed Objects For Cloud Computing
- Building Secure Computer Systems Assignment Help
- Ada Assignment Help
- R Programming Assignment Help
- Oracle Online Assignment Help
- Languages And Automata Assignment Help
- Haskell Assignment Help
- Economics And Computation Assignment Help
- ActionScript Assignment Help
- Audio Programming Assignment Help
- Bash Assignment Help
- Computer Graphics Assignment Help
- Groovy Assignment Help
- Kotlin Assignment Help
- Object Oriented Languages And Environments
- COBOL ASSIGNMENT HELP
- Bayesian Statistical Probabilistic Programming
- Computer Network Assignment Help
- Django Assignment Help
- Lambda Calculus Assignment Help
- Operating System Assignment Help
- Computational Learning Theory
- Delphi Assignment Help
- Concurrent Algorithms And Data Structures Assignment Help
- Machine Learning Assignment Help
- Human Computer Interface Assignment Help
- Foundations Of Data Networking Assignment Help
- Continuous Mathematics Assignment Help
- Compiler Assignment Help
- Computational Biology Assignment Help
- PostgreSQL Online Assignment Help
- Lua Assignment Help
- Human Computer Interaction Assignment Help
- Ethics And Responsible Innovation Assignment Help
- Communication And Ethical Issues In Computing
- Computer Science
- Combinatorial Optimisation Assignment Help
- Ethical Computing In Practice
- HTML Homework Assignment Help
- Linear Algebra Assignment Help
- Perl Assignment Help
- Artificial Intelligence Assignment Help
- Uncategorized
- Ethics And Professionalism Assignment Help
- Human Augmentics Assignment Help
- Linux Assignment Help
- PHP Assignment Help
- Assembly Language Assignment Help
- Dart Assignment Help
- Complete Python Bootcamp From Zero To Hero In Python Corrected Version
- Swift Assignment Help
- Computational Complexity Assignment Help
- Probability And Computing Assignment Help
- MATLAB Programming For Engineers
- Introduction To Statistical Learning
- Database Systems Implementation Assignment Help
- Computational Game Theory Assignment Help
- Database Assignment Help
- Probabilistic Model Checking Assignment Help
- Mathematics For Computer Science And Philosophy
- Introduction To Formal Proof Assignment Help
- Creative Coding Assignment Help
- Foundations Of Self-Programming Agents Assignment Help
- Machine Organization Assignment Help
- Software Design Assignment Help
- Data Communication And Networking Assignment Help
- Computational Biology
- Data Structure Assignment Help
- Foundations Of Software Engineering Assignment Help
- Mathematical Foundations Of Computing
- Principles Of Programming Languages Assignment Help
- Software Engineering Capstone Assignment Help
- Algorithms and Data Structures Assignment Help
No Comments