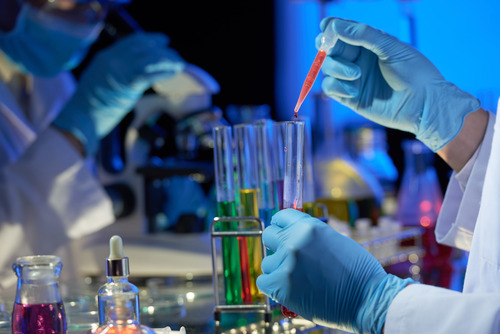
01 May How To Build A Simple React Native App With Expo
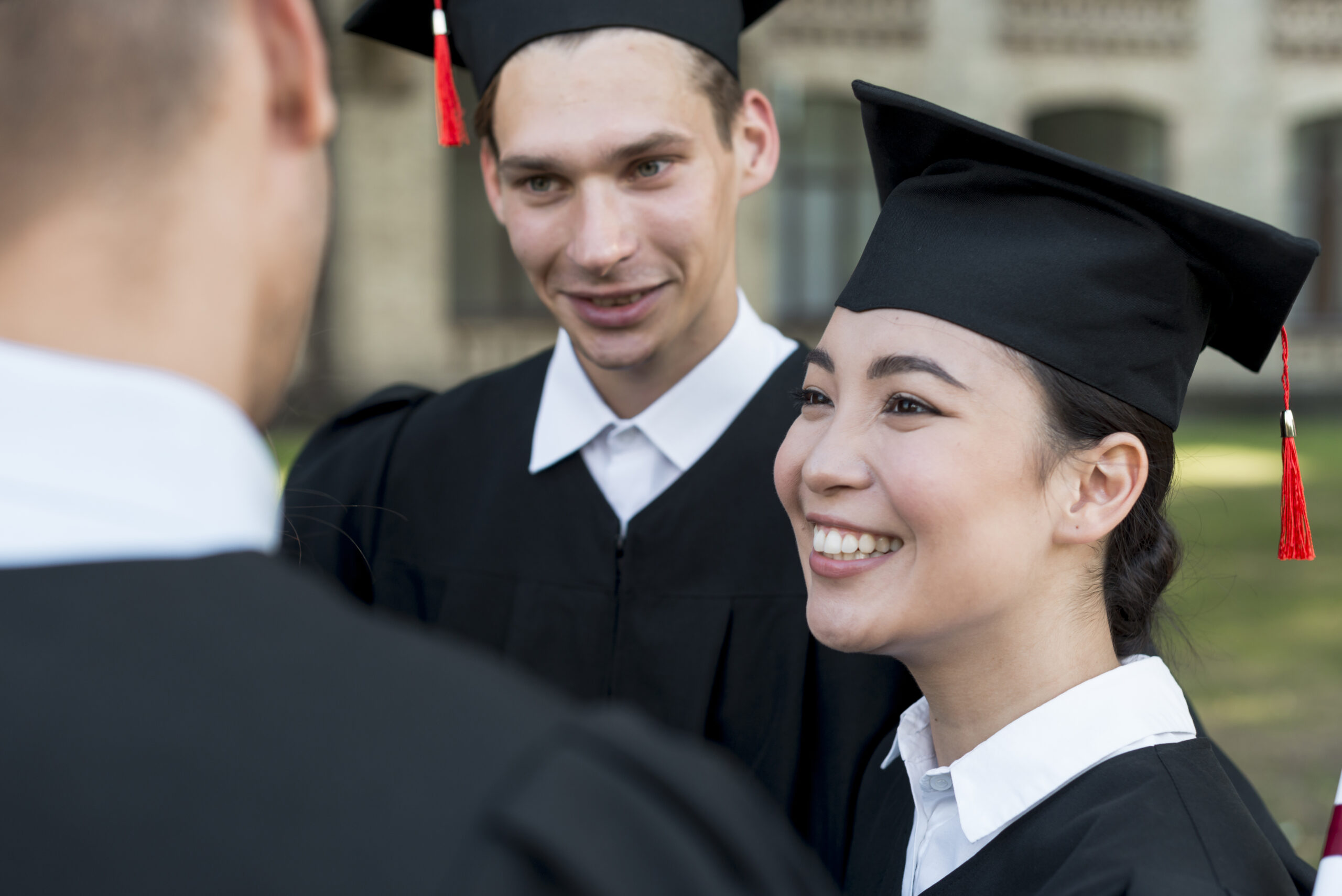
Introduction
React Native is a popular framework for building mobile applications using the React library. Expo is a set of tools and services that makes it easy to develop, build, and deploy React Native applications. In this tutorial, we’ll walk through the process of building a simple React Native app with Expo.
Getting Started
To get started with building a React Native app using Expo, you’ll need to have Node.js and the Expo CLI installed on your computer. You can install Node.js from the official website, and then install the Expo CLI using the following command:
npm install -g expo-cli
Once you have the Expo CLI installed, you can create a new project using the following command:
csharp
expo init my-app
This will create a new React Native project in a directory called my-app
. You can then navigate into the directory and start the development server using the following commands:
cd my-app
expo start
This will start the development server and open a web interface called the “Expo DevTools”. You can use this interface to test your app in a web browser or on your mobile device using the Expo app.
Building the App
Now that we have our project set up, we can start building our app. Let’s start by creating a simple component that displays a message on the screen.
Create a new file called Message.js
in the components
directory with the following code:
import React from 'react';
import { Text } from 'react-native';
const Message = () => {
return (
<Text>Hello, World!</Text>
);
};
export default Message;
This component simply displays the text “Hello, World!” on the screen using the Text
component from the react-native
library.
Now, let’s create a new file called App.js
in the project root directory with the following code:
import React from 'react';
import { StyleSheet, View } from 'react-native';
import Message from './components/Message';
const App = () => {
return (
<View style={styles.container}>
<Message />
</View>
);
};
const styles = StyleSheet.create({
container: {
flex: 1,
alignItems: 'center',
justifyContent: 'center',
},
});
export default App;
This component renders the Message
component in a View
container with some styling using the StyleSheet
API from react-native
.
Finally, let’s update the App.js
file to register the app with Expo and start the development server. Add the following code to the end of the file:
import { StatusBar } from 'expo-status-bar';
import React from 'react';
import { StyleSheet, View } from 'react-native';
import Message from './components/Message';
import { registerRootComponent } from 'expo';
const App = () => {
return (
<View style={styles.container}>
<Message />
<StatusBar style="auto" />
</View>
);
};
const styles = StyleSheet.create({
container: {
flex: 1,
alignItems: 'center',
justifyContent: 'center',
},
});
export default registerRootComponent(App);
The registerRootComponent
function from Expo is used to register the app component as the root component of the app. The StatusBar
component is also added to provide a status bar at the top of the screen.
Testing the App
Jest is a popular testing framework for React Native apps. It is a testing framework that provides a simple API for writing tests and comes with built-in test runners, assertions, and mocks. Enzyme, on the other hand, is a JavaScript testing utility for React that makes it easier to assert, manipulate, and traverse your React components’ output.
To test the app, we will use Jest and Enzyme together. Jest will be used to run the tests, while Enzyme will be used to test React components’ output.
Setting up Jest and Enzyme
Before we can start testing, we need to set up Jest and Enzyme. To do this, we need to install the required dependencies.
npm install --save-dev jest @types/jest enzyme @types/enzyme enzyme-adapter-react-16 @react-native-community/cli
We also need to add a Jest configuration file to the project’s root directory. Create a new file called jest.config.js
with the following contents:
module.exports = {
preset: 'react-native',
setupFilesAfterEnv: ['<rootDir>/src/setupTests.js'],
moduleNameMapper: {
'^~/(.*)$': '<rootDir>/src/$1',
},
};
This configuration tells Jest to use the react-native
preset and specifies the setupTests.js
file to be run before each test. The moduleNameMapper
option allows us to create aliases for our project’s directories.
import Enzyme from 'enzyme';
import Adapter from 'enzyme-adapter-react-16';
import '@testing-library/jest-native/extend-expect';
Enzyme.configure({ adapter: new Adapter() });
This file imports Enzyme and the Enzyme adapter for React 16. It also extends the Jest expect API with the testing-library/jest-native package. Finally, it configures Enzyme to use the adapter.
Writing Tests
Now that we have Jest and Enzyme set up, we can start writing tests. Let’s write a simple test to check if the app’s title is correct. Create a new file called App.test.js
in the src
directory with the following contents:
import React from 'react';
import { Text } from 'react-native';
import { shallow } from 'enzyme';
import App from './App';
describe('App', () => {
it('should render the title', () => {
const wrapper = shallow(<App />);
expect(wrapper.find(Text).contains('My Awesome App')).toBe(true);
});
});
This test imports React, Text component from react-native
, shallow from Enzyme, and the App
component. It then uses the describe and it functions from Jest to define a test suite and a test case. The test case uses Enzyme’s shallow function to create a shallow render of the App
component and then checks if the Text component with the title of the app is rendered.
Running Tests
Now that we have written our test, we can run it using Jest. To run the test, run the following command:
npm test
This will run Jest in watch mode, which will watch for changes in the code and re-run the tests when changes are detected.
Conclusion
In conclusion, building a React Native app with Expo is a great way to quickly create a cross-platform mobile app with a native look and feel. With the help of Expo CLI, we can quickly set up a new project, run it on a simulator, and test it on a device. We have also seen how to use React Navigation to create a navigation stack and navigate between screens.
Testing is an essential part of any software development process, and React Native apps are no exception. We have seen how to set up Jest and Enzyme for testing a React Native app, and how to write a simple test and run it using Jest.
With the knowledge gained from this tutorial, you should be able to build a simple React Native app with Expo, set up a navigation stack, and test your app to ensure it is functioning as expected. By following best practices and keeping up with the latest updates and features, you can create high-quality apps that provide a great user experience.
Latest Topic
-
Cloud-Native Technologies: Best Practices
20 April, 2024 -
Generative AI with Llama 3: Shaping the Future
15 April, 2024 -
Mastering Llama 3: The Ultimate Guide
10 April, 2024
Category
- Assignment Help
- Homework Help
- Programming
- Trending Topics
- C Programming Assignment Help
- Art, Interactive, And Robotics
- Networked Operating Systems Programming
- Knowledge Representation & Reasoning Assignment Help
- Digital Systems Assignment Help
- Computer Design Assignment Help
- Artificial Life And Digital Evolution
- Coding and Fundamentals: Working With Collections
- UML Online Assignment Help
- Prolog Online Assignment Help
- Natural Language Processing Assignment Help
- Julia Assignment Help
- Golang Assignment Help
- Design Implementation Of Network Protocols
- Computer Architecture Assignment Help
- Object-Oriented Languages And Environments
- Coding Early Object and Algorithms: Java Coding Fundamentals
- Deep Learning In Healthcare Assignment Help
- Geometric Deep Learning Assignment Help
- Models Of Computation Assignment Help
- Systems Performance And Concurrent Computing
- Advanced Security Assignment Help
- Typescript Assignment Help
- Computational Media Assignment Help
- Design And Analysis Of Algorithms
- Geometric Modelling Assignment Help
- JavaScript Assignment Help
- MySQL Online Assignment Help
- Programming Practicum Assignment Help
- Public Policy, Legal, And Ethical Issues In Computing, Privacy, And Security
- Computer Vision
- Advanced Complexity Theory Assignment Help
- Big Data Mining Assignment Help
- Parallel Computing And Distributed Computing
- Law And Computer Science Assignment Help
- Engineering Distributed Objects For Cloud Computing
- Building Secure Computer Systems Assignment Help
- Ada Assignment Help
- R Programming Assignment Help
- Oracle Online Assignment Help
- Languages And Automata Assignment Help
- Haskell Assignment Help
- Economics And Computation Assignment Help
- ActionScript Assignment Help
- Audio Programming Assignment Help
- Bash Assignment Help
- Computer Graphics Assignment Help
- Groovy Assignment Help
- Kotlin Assignment Help
- Object Oriented Languages And Environments
- COBOL ASSIGNMENT HELP
- Bayesian Statistical Probabilistic Programming
- Computer Network Assignment Help
- Django Assignment Help
- Lambda Calculus Assignment Help
- Operating System Assignment Help
- Computational Learning Theory
- Delphi Assignment Help
- Concurrent Algorithms And Data Structures Assignment Help
- Machine Learning Assignment Help
- Human Computer Interface Assignment Help
- Foundations Of Data Networking Assignment Help
- Continuous Mathematics Assignment Help
- Compiler Assignment Help
- Computational Biology Assignment Help
- PostgreSQL Online Assignment Help
- Lua Assignment Help
- Human Computer Interaction Assignment Help
- Ethics And Responsible Innovation Assignment Help
- Communication And Ethical Issues In Computing
- Computer Science
- Combinatorial Optimisation Assignment Help
- Ethical Computing In Practice
- HTML Homework Assignment Help
- Linear Algebra Assignment Help
- Perl Assignment Help
- Artificial Intelligence Assignment Help
- Uncategorized
- Ethics And Professionalism Assignment Help
- Human Augmentics Assignment Help
- Linux Assignment Help
- PHP Assignment Help
- Assembly Language Assignment Help
- Dart Assignment Help
- Complete Python Bootcamp From Zero To Hero In Python Corrected Version
- Swift Assignment Help
- Computational Complexity Assignment Help
- Probability And Computing Assignment Help
- MATLAB Programming For Engineers
- Introduction To Statistical Learning
- Database Systems Implementation Assignment Help
- Computational Game Theory Assignment Help
- Database Assignment Help
- Probabilistic Model Checking Assignment Help
- Mathematics For Computer Science And Philosophy
- Introduction To Formal Proof Assignment Help
- Creative Coding Assignment Help
- Foundations Of Self-Programming Agents Assignment Help
- Machine Organization Assignment Help
- Software Design Assignment Help
- Data Communication And Networking Assignment Help
- Computational Biology
- Data Structure Assignment Help
- Foundations Of Software Engineering Assignment Help
- Mathematical Foundations Of Computing
- Principles Of Programming Languages Assignment Help
- Software Engineering Capstone Assignment Help
- Algorithms and Data Structures Assignment Help
No Comments