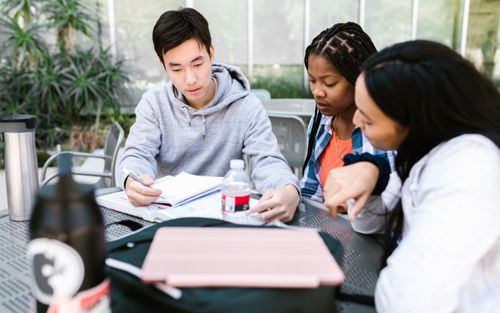
01 May How To Build A Simple Web Scraping Tool With Python
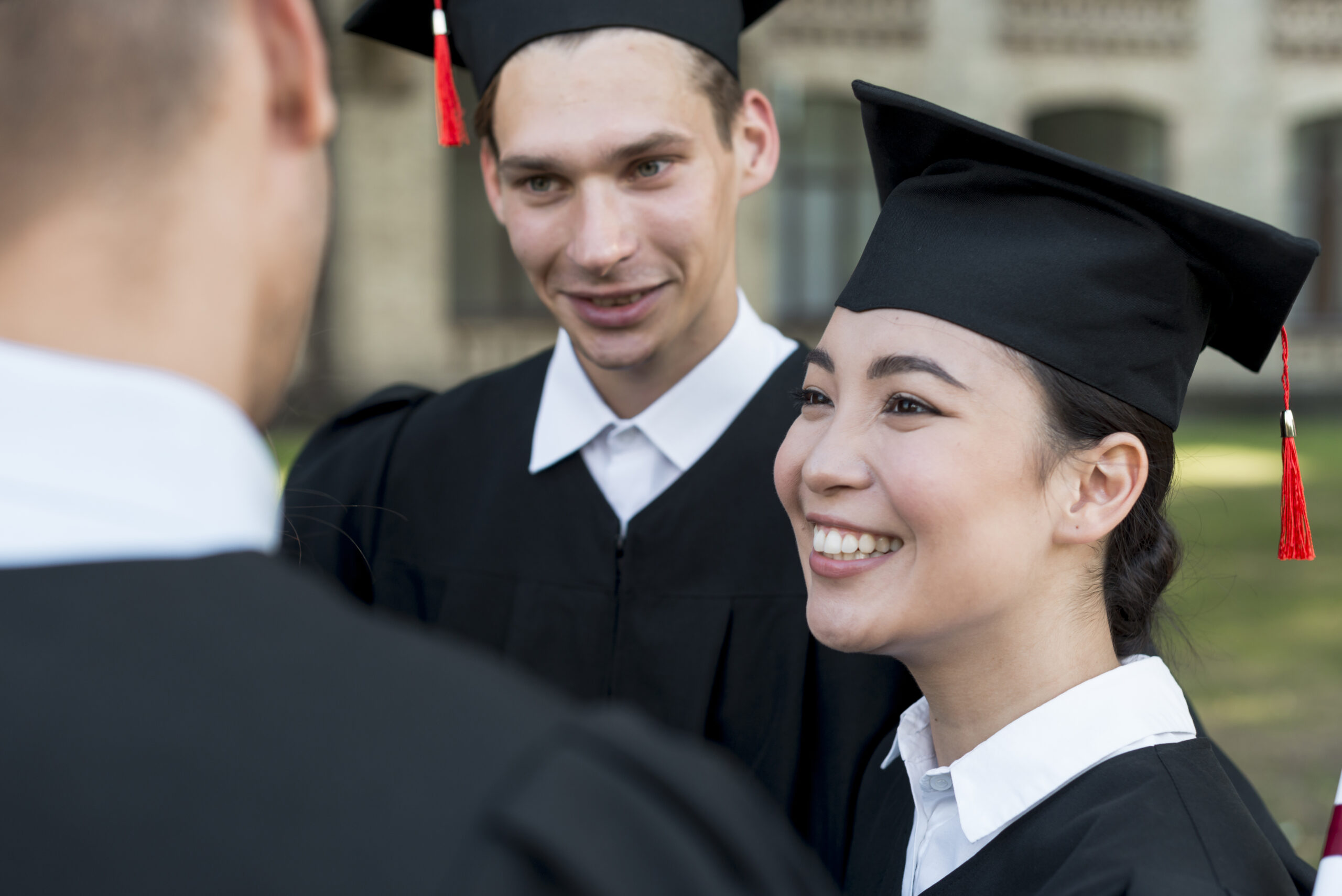
Introduction
Web scraping is a powerful tool that allows developers to extract information from websites in a structured and automated way. Python is a popular language for web scraping due to its ease of use and the abundance of libraries available for it. In this article, we will go through the steps involved in building a simple web scraping tool with Python.
What is Web Scraping?
Web scraping is the process of extracting data from websites. This data can be in the form of text, images, or other media. The purpose of web scraping is to extract data in a structured and automated way, allowing developers to access and use the information for a variety of purposes.
Web scraping is used in a variety of industries, including finance, marketing, and e-commerce. Some common use cases for web scraping include market research, data analysis, and competitor analysis.
Why Python for Web Scraping?
Python is a popular language for web scraping due to its ease of use and the abundance of libraries available for it. Python’s syntax is simple and straightforward, making it easy for beginners to learn. Additionally, Python has a large community of developers who have created libraries specifically for web scraping.
Some of the most popular Python libraries for web scraping include Beautiful Soup, Scrapy, and Requests. These libraries make it easy to extract data from websites and save it in a structured format.
Steps to Build a Simple Web Scraping Tool with Python
Step 1: Install the Required Libraries
Before we can start building our web scraping tool, we need to install the required libraries. For this tutorial, we will be using Beautiful Soup and Requests. You can install these libraries using pip, the package installer for Python:
pip install beautifulsoup4 requests
Step 2: Inspect the Website
The next step is to inspect the website that we want to scrape. Inspecting the website allows us to understand the structure of the HTML code and identify the elements that we want to extract.
To inspect a website, simply right-click on the page and select “Inspect” from the context menu. This will open the browser’s developer tools, which allow you to inspect the HTML code of the page.
Step 3: Send a Request to the Website
Once we have identified the elements that we want to extract, we can send a request to the website using the Requests library. The request allows us to retrieve the HTML code of the page, which we can then parse using Beautiful Soup.
Here’s an example of how to send a request using the Requests library:
import requests
url = "https://example.com"
response = requests.get(url)
print(response.content)
Step 4: Parse the HTML Code with Beautiful Soup
Once we have retrieved the HTML code of the page, we can parse it using Beautiful Soup. Beautiful Soup allows us to extract specific elements from the HTML code, such as links, images, and text.
Here’s an example of how to parse HTML code using Beautiful Soup:
from bs4 import BeautifulSoup
soup = BeautifulSoup(response.content, "html.parser")
# Find all the links on the page
links = soup.find_all("a")
# Find all the images on the page
images = soup.find_all("img")
# Find the title of the page
title = soup.title.string
Step 5: Save the Extracted Data
Once we have extracted the data from the website, we can save it in a structured format. For example, we could save the data in a CSV file or a database.
Here’s an example of how to save the data in a CSV file:
# Create a CSV writer
writer = csv.writer(file)
# Write the headers to the file
writer.writerow(headers)
# Loop through the links and images and write them to the file
for link, image in zip(links, images):
writer.writerow([title, link.get("href"), image.get("src")])
Conclusion
In this article, we have gone through the steps involved in building a simple web scraping tool with Python. We started by installing the required libraries, then inspected the website that we wanted to scrape. We then sent a request to the website using the Requests library, parsed the HTML code with Beautiful Soup, and saved the extracted data in a structured format.
Web scraping is a powerful tool that can be used for a variety of purposes, such as market research, data analysis, and competitor analysis. Python is a popular language for web scraping due to its ease of use and the abundance of libraries available for it.
By following the steps outlined in this article, you should now have a good understanding of how to build a simple web scraping tool with Python. From here, you can explore more advanced techniques and libraries to further enhance your web scraping skills.
Latest Topic
-
Cloud-Native Technologies: Best Practices
20 April, 2024 -
Generative AI with Llama 3: Shaping the Future
15 April, 2024 -
Mastering Llama 3: The Ultimate Guide
10 April, 2024
Category
- Assignment Help
- Homework Help
- Programming
- Trending Topics
- C Programming Assignment Help
- Art, Interactive, And Robotics
- Networked Operating Systems Programming
- Knowledge Representation & Reasoning Assignment Help
- Digital Systems Assignment Help
- Computer Design Assignment Help
- Artificial Life And Digital Evolution
- Coding and Fundamentals: Working With Collections
- UML Online Assignment Help
- Prolog Online Assignment Help
- Natural Language Processing Assignment Help
- Julia Assignment Help
- Golang Assignment Help
- Design Implementation Of Network Protocols
- Computer Architecture Assignment Help
- Object-Oriented Languages And Environments
- Coding Early Object and Algorithms: Java Coding Fundamentals
- Deep Learning In Healthcare Assignment Help
- Geometric Deep Learning Assignment Help
- Models Of Computation Assignment Help
- Systems Performance And Concurrent Computing
- Advanced Security Assignment Help
- Typescript Assignment Help
- Computational Media Assignment Help
- Design And Analysis Of Algorithms
- Geometric Modelling Assignment Help
- JavaScript Assignment Help
- MySQL Online Assignment Help
- Programming Practicum Assignment Help
- Public Policy, Legal, And Ethical Issues In Computing, Privacy, And Security
- Computer Vision
- Advanced Complexity Theory Assignment Help
- Big Data Mining Assignment Help
- Parallel Computing And Distributed Computing
- Law And Computer Science Assignment Help
- Engineering Distributed Objects For Cloud Computing
- Building Secure Computer Systems Assignment Help
- Ada Assignment Help
- R Programming Assignment Help
- Oracle Online Assignment Help
- Languages And Automata Assignment Help
- Haskell Assignment Help
- Economics And Computation Assignment Help
- ActionScript Assignment Help
- Audio Programming Assignment Help
- Bash Assignment Help
- Computer Graphics Assignment Help
- Groovy Assignment Help
- Kotlin Assignment Help
- Object Oriented Languages And Environments
- COBOL ASSIGNMENT HELP
- Bayesian Statistical Probabilistic Programming
- Computer Network Assignment Help
- Django Assignment Help
- Lambda Calculus Assignment Help
- Operating System Assignment Help
- Computational Learning Theory
- Delphi Assignment Help
- Concurrent Algorithms And Data Structures Assignment Help
- Machine Learning Assignment Help
- Human Computer Interface Assignment Help
- Foundations Of Data Networking Assignment Help
- Continuous Mathematics Assignment Help
- Compiler Assignment Help
- Computational Biology Assignment Help
- PostgreSQL Online Assignment Help
- Lua Assignment Help
- Human Computer Interaction Assignment Help
- Ethics And Responsible Innovation Assignment Help
- Communication And Ethical Issues In Computing
- Computer Science
- Combinatorial Optimisation Assignment Help
- Ethical Computing In Practice
- HTML Homework Assignment Help
- Linear Algebra Assignment Help
- Perl Assignment Help
- Artificial Intelligence Assignment Help
- Uncategorized
- Ethics And Professionalism Assignment Help
- Human Augmentics Assignment Help
- Linux Assignment Help
- PHP Assignment Help
- Assembly Language Assignment Help
- Dart Assignment Help
- Complete Python Bootcamp From Zero To Hero In Python Corrected Version
- Swift Assignment Help
- Computational Complexity Assignment Help
- Probability And Computing Assignment Help
- MATLAB Programming For Engineers
- Introduction To Statistical Learning
- Database Systems Implementation Assignment Help
- Computational Game Theory Assignment Help
- Database Assignment Help
- Probabilistic Model Checking Assignment Help
- Mathematics For Computer Science And Philosophy
- Introduction To Formal Proof Assignment Help
- Creative Coding Assignment Help
- Foundations Of Self-Programming Agents Assignment Help
- Machine Organization Assignment Help
- Software Design Assignment Help
- Data Communication And Networking Assignment Help
- Computational Biology
- Data Structure Assignment Help
- Foundations Of Software Engineering Assignment Help
- Mathematical Foundations Of Computing
- Principles Of Programming Languages Assignment Help
- Software Engineering Capstone Assignment Help
- Algorithms and Data Structures Assignment Help
No Comments