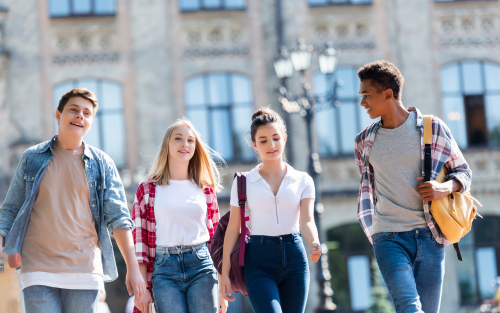
26 Apr How To Build A Single-Page Application With Angular
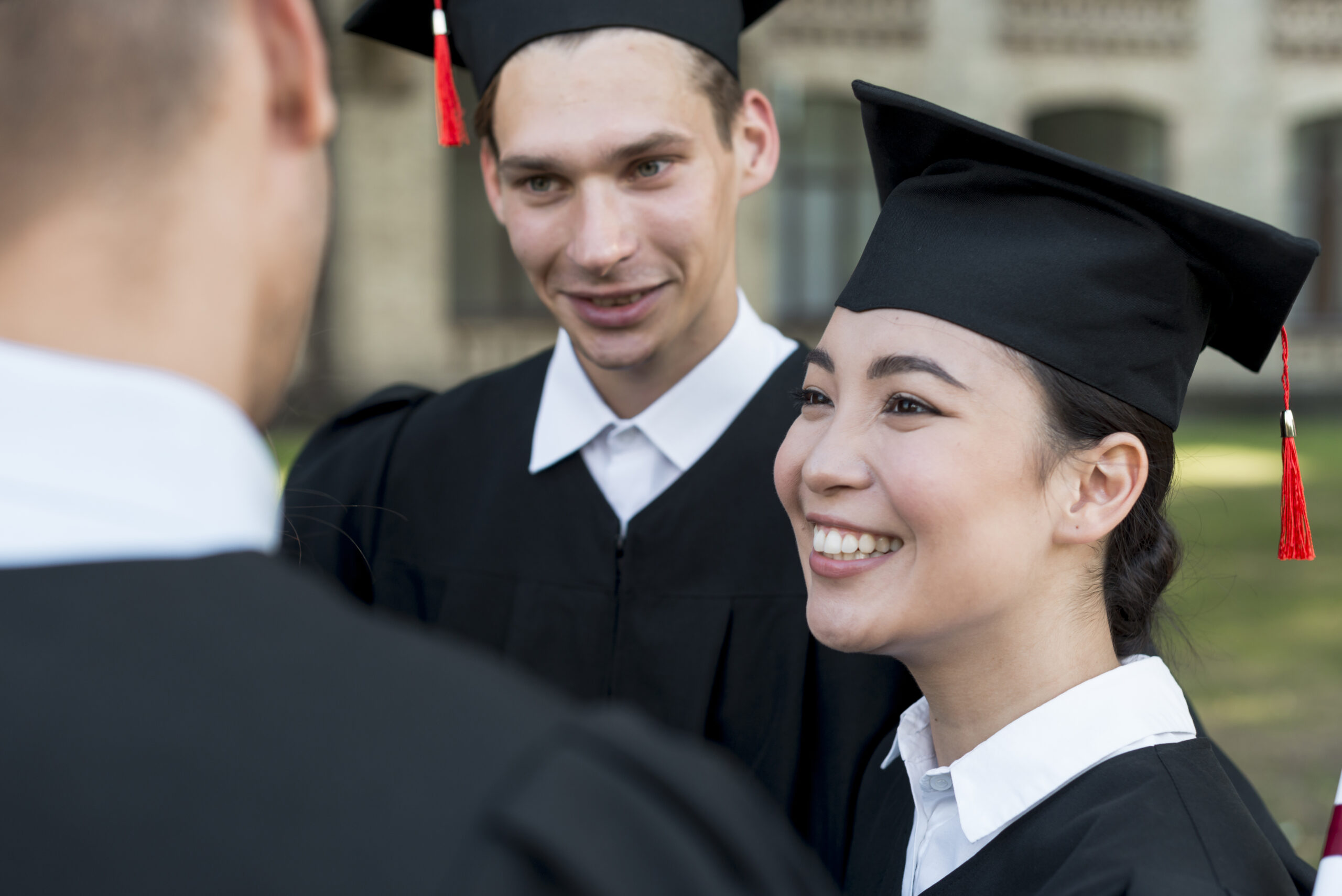
Angular is a powerful JavaScript framework that is widely used for building dynamic, single-page applications (SPAs). SPAs are web applications that load a single HTML page and dynamically update the content based on user interaction, without requiring a page refresh. In this blog, we will walk through the steps involved in building a single-page application with Angular.
Setting Up the Environment
To start building an Angular application, we need to set up the development environment. First, we need to install Node.js and npm (Node Package Manager) on our machine. Then, we can use npm to install the Angular CLI (Command Line Interface) globally by running the following command:
npm install -g @angular/cli
Once the installation is complete, we can create a new Angular project using the CLI by running the following command:
ng new my-app
This command generates a new Angular project in a directory named “my-app” with all the necessary files and configurations.
Creating Components
In Angular, components are the building blocks of an application. They are responsible for managing the view and the logic associated with it. To create a new component, we can use the CLI by running the following command:
ng generate component my-component
This command generates a new component in a directory named “my-component” with all the necessary files, including a TypeScript file, an HTML template, and a CSS stylesheet.
Defining Routes
In an SPA, the content is dynamically updated based on user interaction, which requires defining routes that map to different components. To define routes in Angular, we can use the RouterModule, which is a built-in module that provides a mechanism for defining routes.
First, we need to import the RouterModule and Routes modules in our app.module.ts file:
import { RouterModule, Routes } from '@angular/router';
Then, we can define our routes as an array of Route objects:
const routes: Routes = [
{ path: '', component: HomeComponent },
{ path: 'about', component: AboutComponent },
{ path: 'contact', component: ContactComponent },
];
In this example, we defined three routes: an empty path that maps to the HomeComponent, a path named “about” that maps to the AboutComponent, and a path named “contact” that maps to the ContactComponent.
Creating Services
Services are used to encapsulate reusable functionality that can be shared across different components. In Angular, we can create a new service using the CLI by running the following command:
ng generate service my-service
This command generates a new service in a directory named “my-service” with a TypeScript file that we can use to define our service.
Retrieving Data from a Server
In an SPA, we often need to retrieve data from a server to display it to the user. In Angular, we can use the HttpClient module to make HTTP requests to a server.
First, we need to import the HttpClientModule in our app.module.ts file:
import { HttpClientModule } from '@angular/common/http';
Then, we can use the HttpClient service in our component or service to make HTTP requests. For example, to retrieve data from a server, we can use the following code:
import { HttpClient } from '@angular/common/http';
constructor(private http: HttpClient) {}
getData() {
return this.http.get('/api/data');
}
Building and Deploying the Application
Once we have developed our Angular application, we need to build it for production and deploy it to a server. To build the application, we can use the CLI by running the following command:
ng build --prod
Adding Routing
In order to navigate between different components in the single-page application, we need to add routing. Angular provides a powerful routing module that allows us to define routes for different components and handle navigation between them.
First, let’s import the RouterModule and Routes from the @angular/router module in the app.module.ts file.
import { RouterModule, Routes } from '@angular/router';
Next, let’s define the routes for our application in a constant array called appRoutes. In this example, we will have two routes: one for the home page and one for the about page.
const appRoutes: Routes = [
{ path: '', component: HomeComponent },
{ path: 'about', component: AboutComponent }
];
The first route maps the root URL (”) to the HomeComponent, and the second route maps the ‘/about’ URL to the AboutComponent.
Now we need to import the appRoutes and RouterModule into our app.module.ts file and add them to the imports array.
imports: [
BrowserModule,
RouterModule.forRoot(appRoutes)
],
We have now added routing to our application. However, we still need to add the router outlet to our app.component.html file. The router outlet is a placeholder that Angular uses to insert the component that matches the current route.
<router-outlet></router-outlet>
Now if we navigate to the root URL (‘/’) or the ‘/about’ URL, Angular will load the corresponding component into the router outlet.
Creating a Navigation Menu
To make it easier for users to navigate between different pages in our single-page application, we can create a navigation menu that uses the Angular router to navigate to different components.
In our app.component.html file, let’s add a navigation bar with links to the home and about pages.
<nav>
<a routerLink="/">Home</a>
<a routerLink="/about">About</a>
</nav>
<router-outlet></router-outlet>
The routerLink directive is used to navigate to the corresponding route when the link is clicked. We have also added the router outlet to the end of the template to display the appropriate component for each route.
Conclusion
In this tutorial, we have learned how to build a single-page application with Angular. We covered the basics of Angular components, templates, and services, and demonstrated how to use them to create a simple application. We also learned how to add routing to the application, which allows us to navigate between different components. Finally, we created a navigation menu to make it easier for users to navigate between different pages in the application.
Angular is a powerful framework for building single-page applications, and with its robust set of features and easy-to-use syntax, it is an excellent choice for developing complex applications. By following the principles and best practices outlined in this tutorial, you can build scalable and maintainable applications that will delight your users.
Latest Topic
-
Cloud-Native Technologies: Best Practices
20 April, 2024 -
Generative AI with Llama 3: Shaping the Future
15 April, 2024 -
Mastering Llama 3: The Ultimate Guide
10 April, 2024
Category
- Assignment Help
- Homework Help
- Programming
- Trending Topics
- C Programming Assignment Help
- Art, Interactive, And Robotics
- Networked Operating Systems Programming
- Knowledge Representation & Reasoning Assignment Help
- Digital Systems Assignment Help
- Computer Design Assignment Help
- Artificial Life And Digital Evolution
- Coding and Fundamentals: Working With Collections
- UML Online Assignment Help
- Prolog Online Assignment Help
- Natural Language Processing Assignment Help
- Julia Assignment Help
- Golang Assignment Help
- Design Implementation Of Network Protocols
- Computer Architecture Assignment Help
- Object-Oriented Languages And Environments
- Coding Early Object and Algorithms: Java Coding Fundamentals
- Deep Learning In Healthcare Assignment Help
- Geometric Deep Learning Assignment Help
- Models Of Computation Assignment Help
- Systems Performance And Concurrent Computing
- Advanced Security Assignment Help
- Typescript Assignment Help
- Computational Media Assignment Help
- Design And Analysis Of Algorithms
- Geometric Modelling Assignment Help
- JavaScript Assignment Help
- MySQL Online Assignment Help
- Programming Practicum Assignment Help
- Public Policy, Legal, And Ethical Issues In Computing, Privacy, And Security
- Computer Vision
- Advanced Complexity Theory Assignment Help
- Big Data Mining Assignment Help
- Parallel Computing And Distributed Computing
- Law And Computer Science Assignment Help
- Engineering Distributed Objects For Cloud Computing
- Building Secure Computer Systems Assignment Help
- Ada Assignment Help
- R Programming Assignment Help
- Oracle Online Assignment Help
- Languages And Automata Assignment Help
- Haskell Assignment Help
- Economics And Computation Assignment Help
- ActionScript Assignment Help
- Audio Programming Assignment Help
- Bash Assignment Help
- Computer Graphics Assignment Help
- Groovy Assignment Help
- Kotlin Assignment Help
- Object Oriented Languages And Environments
- COBOL ASSIGNMENT HELP
- Bayesian Statistical Probabilistic Programming
- Computer Network Assignment Help
- Django Assignment Help
- Lambda Calculus Assignment Help
- Operating System Assignment Help
- Computational Learning Theory
- Delphi Assignment Help
- Concurrent Algorithms And Data Structures Assignment Help
- Machine Learning Assignment Help
- Human Computer Interface Assignment Help
- Foundations Of Data Networking Assignment Help
- Continuous Mathematics Assignment Help
- Compiler Assignment Help
- Computational Biology Assignment Help
- PostgreSQL Online Assignment Help
- Lua Assignment Help
- Human Computer Interaction Assignment Help
- Ethics And Responsible Innovation Assignment Help
- Communication And Ethical Issues In Computing
- Computer Science
- Combinatorial Optimisation Assignment Help
- Ethical Computing In Practice
- HTML Homework Assignment Help
- Linear Algebra Assignment Help
- Perl Assignment Help
- Artificial Intelligence Assignment Help
- Uncategorized
- Ethics And Professionalism Assignment Help
- Human Augmentics Assignment Help
- Linux Assignment Help
- PHP Assignment Help
- Assembly Language Assignment Help
- Dart Assignment Help
- Complete Python Bootcamp From Zero To Hero In Python Corrected Version
- Swift Assignment Help
- Computational Complexity Assignment Help
- Probability And Computing Assignment Help
- MATLAB Programming For Engineers
- Introduction To Statistical Learning
- Database Systems Implementation Assignment Help
- Computational Game Theory Assignment Help
- Database Assignment Help
- Probabilistic Model Checking Assignment Help
- Mathematics For Computer Science And Philosophy
- Introduction To Formal Proof Assignment Help
- Creative Coding Assignment Help
- Foundations Of Self-Programming Agents Assignment Help
- Machine Organization Assignment Help
- Software Design Assignment Help
- Data Communication And Networking Assignment Help
- Computational Biology
- Data Structure Assignment Help
- Foundations Of Software Engineering Assignment Help
- Mathematical Foundations Of Computing
- Principles Of Programming Languages Assignment Help
- Software Engineering Capstone Assignment Help
- Algorithms and Data Structures Assignment Help
No Comments