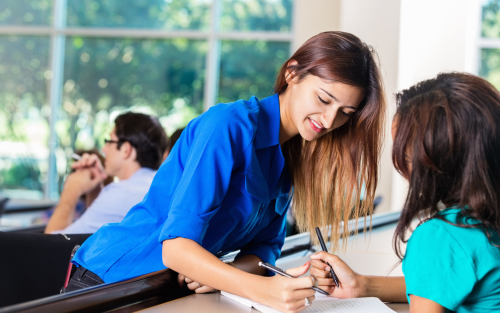
10 May Libraries And Modules In Python
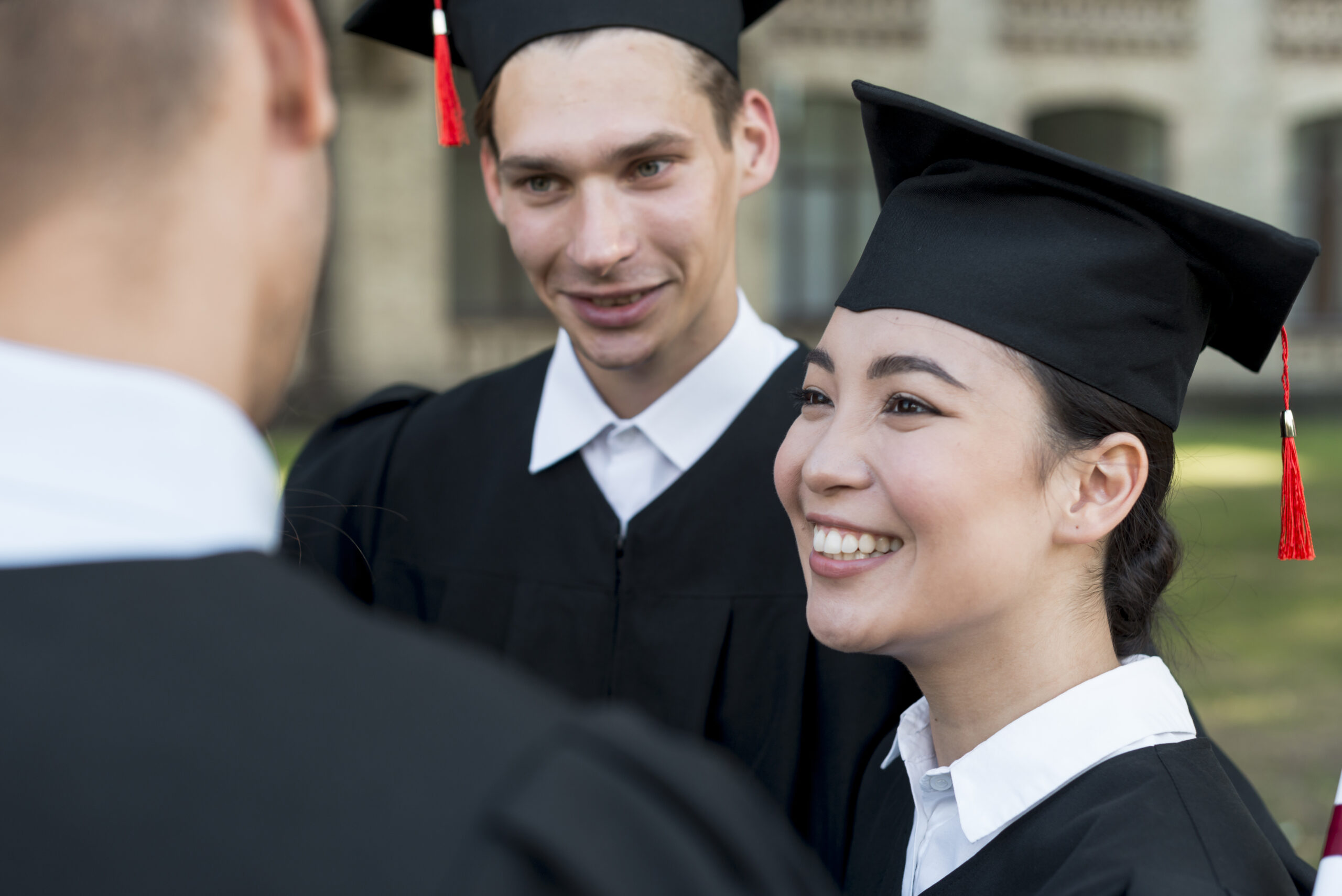
Introduction
Python is a high-level, interpreted programming language that is widely used in various applications such as web development, data analysis, machine learning, and scientific computing. The popularity of Python is due to its simplicity, readability, and versatility, which make it easy to learn and use.
One of the key features that make Python a favorite among developers is its rich library of modules and packages. Python modules are pre-written code that can be imported into a Python script to provide additional functionality. These modules can be used to handle common programming tasks, such as working with files, network communication, and data manipulation.
Explore a wide range of libraries and modules available in Python to enhance your programming capabilities. Python libraries and modules extend the functionality of the language and provide ready-to-use tools for various tasks. Discover popular libraries like NumPy, Pandas, Matplotlib, scikit-learn, TensorFlow, and more for data manipulation, analysis, visualization, machine learning, and deep learning. Dive into modules such as os, datetime, math, and random for system operations, date/time manipulation, mathematical calculations, and generating random numbers. Unlock the power of Python libraries and modules to streamline your development process and accelerate your projects.
In this article, we will explore the basics of libraries and modules in Python, their importance, and how to use them in your Python projects.
What are Libraries and Modules?
In Python, a library is a collection of related modules that provide a set of functionalities for a specific purpose. A module is a file containing Python definitions and statements. A library can contain one or more modules, and a module can be part of one or more libraries.
Python comes with a standard library that provides a wide range of modules for different purposes. The standard library is included with every installation of Python, and it contains modules for handling various tasks such as file input/output, regular expressions, network communication, and more.
Python also has a vast collection of third-party libraries that are available for download and installation from the Python Package Index (PyPI). These libraries can be used to extend the functionality of Python, and they cover a wide range of domains, such as web development, machine learning, data analysis, and more.
Importing Modules in Python
To use a module in your Python code, you need to import it first. The import statement is used to bring a module into your Python script. The syntax for importing a module is as follows:
import module_name
For example, to import the math module, you can use the following statement:
import math
Once you have imported a module, you can use its functions, classes, and variables in your code by prefixing them with the module name. For example, to use the square root function from the math module, you can use the following code:
import math
x = math.sqrt(25)
print(x)
This code will output the value of the square root of 25, which is 5.
You can also import specific functions or classes from a module using the from…import statement. This statement allows you to import only the functions or classes you need from a module, which can help reduce the amount of code you need to write.
from module_name import function_name
For example, to import only the square root function from the math module, you can use the following statement:
from math import sqrt
x = sqrt(25)
print(x)
This code will also output the value of the square root of 25, which is 5.
Using Standard Library Modules
Python’s standard library provides a wide range of modules for handling various tasks. These modules are available in every Python installation, and they can be used for many common programming tasks.
Some of the most commonly used standard library modules in Python include:
Python’s standard library includes a vast collection of modules that provide a wide range of functionalities, from basic data types and structures to advanced scientific computing and web development tools. Here are some of the most commonly used standard library modules in Python:
os
– This module provides a way to interact with the operating system, allowing you to access the file system, environment variables, and other system-level functionalities.
sys
– This module provides access to some variables used or maintained by the interpreter, such as the command-line arguments passed to the script, and the Python interpreter’s version.
math
– This module provides access to some of the mathematical functions like trigonometric, logarithmic, and exponential functions.
datetime
– This module provides classes for working with dates and times, including time zone support, date arithmetic, and parsing and formatting of dates and times.
random
– This module provides functions for generating random numbers, shuffling sequences randomly, and generating random selections from a sequence.
collections
– This module provides specialized container datatypes like deque
, Counter
, defaultdict
, and namedtuple
, which are alternatives to the built-in containers like lists, dictionaries, and tuples.
re
– This module provides regular expression matching operations, allowing you to search and manipulate strings based on patterns.
json
– This module provides functions for encoding and decoding JSON data, which is commonly used for data interchange between web services and applications.
urllib
– This module provides a high-level interface for working with URLs, allowing you to make HTTP requests and retrieve data from the web.
sqlite3
– This module provides a lightweight, serverless database engine that allows you to store and retrieve data from a local SQLite database file.
These are just a few examples of the many modules available in Python’s standard library. Depending on the task at hand, there are many more modules that can be used to accomplish a wide range of tasks, from web development to scientific computing to network programming.
Conclusion
In conclusion, the standard library in Python is a powerful collection of modules and functions that come bundled with the language itself. These modules provide a wide range of functionality, including file input/output, system interactions, mathematical operations, and much more. By leveraging these modules, developers can write Python programs with less effort and in less time.
Some of the most commonly used standard library modules in Python include os
, sys
, math
, datetime
, random
, collections
, re
, json
, urllib
, and sqlite3
. Each of these modules has its own set of functions and methods that can be used to solve specific programming tasks.
In addition to the standard library, Python has a vast ecosystem of third-party modules and libraries that extend the language’s capabilities even further. These modules are available for free and can be installed using Python’s package manager, pip
. Some popular third-party modules include numpy
for scientific computing, pandas
for data analysis, matplotlib
for data visualization, and Django
for web development.
Overall, Python’s standard library and third-party modules make it one of the most versatile and widely used programming languages today. Whether you are a beginner or an experienced developer, Python’s rich collection of modules and libraries provide a powerful toolkit to help you accomplish your programming tasks efficiently and effectively.
Case Study
Using Libraries and Modules in Python for Data Analysis
Let’s take a look at a real-life example of how libraries and modules can be used in Python for data analysis. Suppose we have a large dataset containing information about customers, including their age, gender, income, and spending habits. We want to analyze this data to gain insights into our customers’ behavior and preferences.
To do this, we can use several Python libraries and modules, such as:
Pandas – a powerful library for data manipulation and analysis.
NumPy – a library for numerical computing.
Matplotlib – a library for creating data visualizations.
We can start by importing the necessary libraries and loading our dataset into a Pandas DataFrame:
import pandas as pd
import numpy as np
# Load the dataset into a Pandas DataFrame
df = pd.read_csv(‘customer_data.csv’)
Next, we can use Pandas to clean and manipulate the data, such as removing any null values, renaming columns, and calculating summary statistics:
# Remove any null values from the DataFrame
df.dropna(inplace=True)
# Rename the columns for easier readability
df.rename(columns={‘age’: ‘Age’, ‘gender’: ‘Gender’, ‘income’: ‘Income’, ‘spending’: ‘Spending’}, inplace=True)
# Calculate summary statistics for the data
summary = df.describe()
print(summary)
Finally, we can use Matplotlib to create visualizations that help us understand the data, such as a scatter plot to visualize the relationship between income and spending:
import matplotlib.pyplot as plt
# Create a scatter plot of income vs. spending
plt.scatter(df[‘Income’], df[‘Spending’])
plt.xlabel(‘Income’)
plt.ylabel(‘Spending’)
plt.title(‘Income vs. Spending’)
plt.show()
This example demonstrates how Python libraries and modules can be used together to perform complex data analysis tasks quickly and easily.
FAQs
Q: What are some of the advantages of using libraries and modules in Python?
A: Using libraries and modules can save time and effort when programming, as they provide pre-written code that can be used to perform common tasks quickly and easily. They also allow developers to take advantage of the work of others and build on top of existing code, which can lead to faster development and better code quality.
Q: How do I install third-party libraries and modules in Python?
A: Third-party libraries and modules can be installed using the pip package manager. To install a library or module, simply run the following command in your terminal or command prompt:
pip install library_name
Q: How do I update a library or module in Python? A: To update a library or module, you can use the following command:
pip install --upgrade library_name
Q: Can I create my own libraries and modules in Python?
A: Yes, you can create your own libraries and modules in Python. Simply write the code you want to include in the library or module, save it in a separate file with a .py extension, and then import it into your Python script using the import statement. You can also distribute your libraries and modules to others by uploading them to PyPI or creating your own repository.
Examples
Here are some examples of how these commonly used standard library modules in Python can be used:
os
import os
# Get the current working directory
cwd = os.getcwd()
print(cwd)
# List all files in a directory
files = os.listdir(‘.’)
print(files)
# Create a new directory
os.mkdir(‘new_directory’)
sys
import sys
# Get the command-line arguments
args = sys.argv
print(args)
# Get the Python version
version = sys.version_info
print(version)
math
import math
# Calculate the square root of a number
x = 16
sqrt = math.sqrt(x)
print(sqrt)
# Calculate the sine of an angle
angle = math.pi / 4
sin = math.sin(angle)
print(sin)
datetime
import datetime
# Get the current date and time
now = datetime.datetime.now()
print(now)
# Create a date object
date = datetime.date(2021, 1, 1)
print(date)
# Add one week to a date
one_week = datetime.timedelta(days=7)
new_date = date + one_week
print(new_date)
random
import random
# Generate a random integer between 0 and 10
rand_int = random.randint(0, 10)
print(rand_int)
# Shuffle a list randomly
my_list = [1, 2, 3, 4, 5]
random.shuffle(my_list)
print(my_list)
# Choose a random item from a list
my_list = [‘apple’, ‘banana’, ‘orange’]
random_item = random.choice(my_list)
print(random_item)
collections
from collections import deque, Counter, defaultdict, namedtuple
# Create a deque
my_deque = deque([1, 2, 3])
my_deque.append(4)
print(my_deque)
# Count the occurrences of items in a list
my_list = [1, 2, 2, 3, 3, 3]
my_counts = Counter(my_list)
print(my_counts)
# Create a defaultdict
my_dict = defaultdict(int)
my_dict[‘a’] += 1
print(my_dict)
# Create a named tuple
Person = namedtuple(‘Person’, [‘name’, ‘age’])
person1 = Person(‘Alice’, 30)
print(person1.name)
re
import re
# Search for a pattern in a string
my_string = ‘The quick brown fox jumps over the lazy dog’
pattern = ‘brown’
match = re.search(pattern, my_string)
print(match)
# Replace a pattern in a string
my_string = ‘The quick brown fox jumps over the lazy dog’
pattern = ‘brown’
new_string = re.sub(pattern, ‘red’, my_string)
print(new_string)
json
import json
# Encode a Python object to JSON
my_dict = {‘name’: ‘Alice’, ‘age’: 30}
json_data = json.dumps(my_dict)
print(json_data)
# Decode a JSON string to a Python object
json_string = ‘{“name”: “Alice”, “age”: 30}’
my_dict = json.loads(json_string)
print(my_dict)
urllib
import urllib.request
# Retrieve data from a URL
url = ‘https://www.python.org/’
response = urllib.request.urlopen(url)
html = response.read()
print(html)
Latest Topic
-
Cloud-Native Technologies: Best Practices
20 April, 2024 -
Generative AI with Llama 3: Shaping the Future
15 April, 2024 -
Mastering Llama 3: The Ultimate Guide
10 April, 2024
Category
- Assignment Help
- Homework Help
- Programming
- Trending Topics
- C Programming Assignment Help
- Art, Interactive, And Robotics
- Networked Operating Systems Programming
- Knowledge Representation & Reasoning Assignment Help
- Digital Systems Assignment Help
- Computer Design Assignment Help
- Artificial Life And Digital Evolution
- Coding and Fundamentals: Working With Collections
- UML Online Assignment Help
- Prolog Online Assignment Help
- Natural Language Processing Assignment Help
- Julia Assignment Help
- Golang Assignment Help
- Design Implementation Of Network Protocols
- Computer Architecture Assignment Help
- Object-Oriented Languages And Environments
- Coding Early Object and Algorithms: Java Coding Fundamentals
- Deep Learning In Healthcare Assignment Help
- Geometric Deep Learning Assignment Help
- Models Of Computation Assignment Help
- Systems Performance And Concurrent Computing
- Advanced Security Assignment Help
- Typescript Assignment Help
- Computational Media Assignment Help
- Design And Analysis Of Algorithms
- Geometric Modelling Assignment Help
- JavaScript Assignment Help
- MySQL Online Assignment Help
- Programming Practicum Assignment Help
- Public Policy, Legal, And Ethical Issues In Computing, Privacy, And Security
- Computer Vision
- Advanced Complexity Theory Assignment Help
- Big Data Mining Assignment Help
- Parallel Computing And Distributed Computing
- Law And Computer Science Assignment Help
- Engineering Distributed Objects For Cloud Computing
- Building Secure Computer Systems Assignment Help
- Ada Assignment Help
- R Programming Assignment Help
- Oracle Online Assignment Help
- Languages And Automata Assignment Help
- Haskell Assignment Help
- Economics And Computation Assignment Help
- ActionScript Assignment Help
- Audio Programming Assignment Help
- Bash Assignment Help
- Computer Graphics Assignment Help
- Groovy Assignment Help
- Kotlin Assignment Help
- Object Oriented Languages And Environments
- COBOL ASSIGNMENT HELP
- Bayesian Statistical Probabilistic Programming
- Computer Network Assignment Help
- Django Assignment Help
- Lambda Calculus Assignment Help
- Operating System Assignment Help
- Computational Learning Theory
- Delphi Assignment Help
- Concurrent Algorithms And Data Structures Assignment Help
- Machine Learning Assignment Help
- Human Computer Interface Assignment Help
- Foundations Of Data Networking Assignment Help
- Continuous Mathematics Assignment Help
- Compiler Assignment Help
- Computational Biology Assignment Help
- PostgreSQL Online Assignment Help
- Lua Assignment Help
- Human Computer Interaction Assignment Help
- Ethics And Responsible Innovation Assignment Help
- Communication And Ethical Issues In Computing
- Computer Science
- Combinatorial Optimisation Assignment Help
- Ethical Computing In Practice
- HTML Homework Assignment Help
- Linear Algebra Assignment Help
- Perl Assignment Help
- Artificial Intelligence Assignment Help
- Uncategorized
- Ethics And Professionalism Assignment Help
- Human Augmentics Assignment Help
- Linux Assignment Help
- PHP Assignment Help
- Assembly Language Assignment Help
- Dart Assignment Help
- Complete Python Bootcamp From Zero To Hero In Python Corrected Version
- Swift Assignment Help
- Computational Complexity Assignment Help
- Probability And Computing Assignment Help
- MATLAB Programming For Engineers
- Introduction To Statistical Learning
- Database Systems Implementation Assignment Help
- Computational Game Theory Assignment Help
- Database Assignment Help
- Probabilistic Model Checking Assignment Help
- Mathematics For Computer Science And Philosophy
- Introduction To Formal Proof Assignment Help
- Creative Coding Assignment Help
- Foundations Of Self-Programming Agents Assignment Help
- Machine Organization Assignment Help
- Software Design Assignment Help
- Data Communication And Networking Assignment Help
- Computational Biology
- Data Structure Assignment Help
- Foundations Of Software Engineering Assignment Help
- Mathematical Foundations Of Computing
- Principles Of Programming Languages Assignment Help
- Software Engineering Capstone Assignment Help
- Algorithms and Data Structures Assignment Help
No Comments