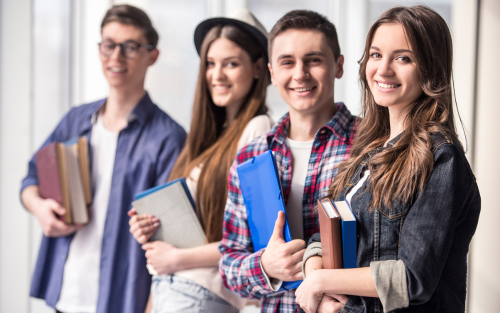
13 May Python Algorithm Design And Analysis Assistance
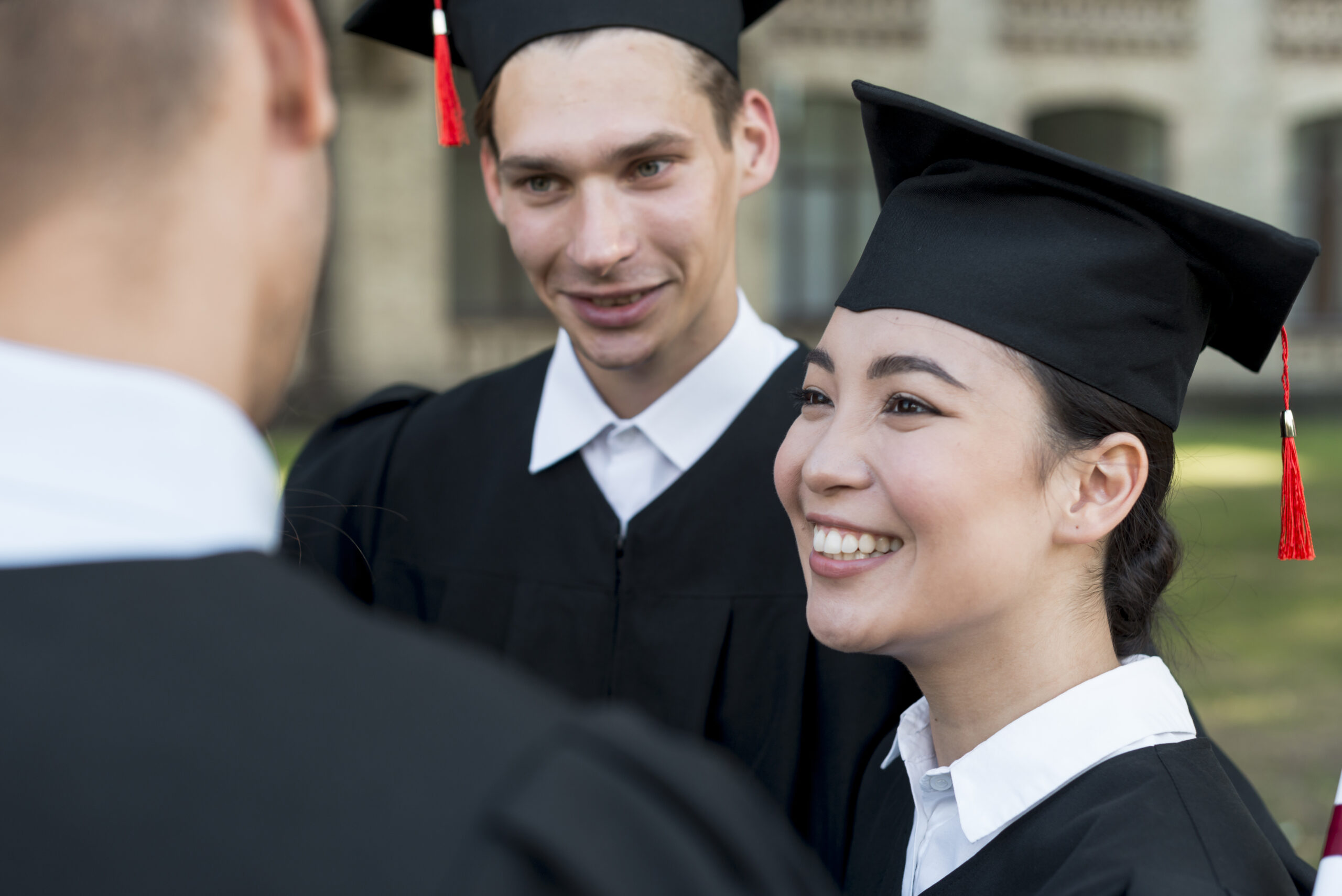
Introduction
Python is a popular programming language used in a variety of industries, including software development, scientific computing, and data analysis. One of the key benefits of using Python is its extensive library of algorithms and data structures, which can be used to solve complex problems efficiently. However, designing and analyzing algorithms can be a challenging task, especially for beginners. In this article, we will discuss the basics of Python algorithm design and analysis and provide guidance on how to approach algorithmic problems.
Need assistance with Python algorithm design and analysis? Get expert guidance and support with our comprehensive Python Algorithm Design and Analysis Assistance services. Our team of experienced programmers and data scientists will help you optimize your code, improve algorithm efficiency, and solve complex problems. Whether you’re a beginner or an advanced programmer, our services will enhance your Python skills and enable you to tackle any algorithmic challenge. Try our Python Algorithm Design and Analysis Assistance today and elevate your programming capabilities.
Understanding Algorithm Design
Algorithm design is the process of creating a step-by-step procedure or set of instructions for solving a particular problem. Algorithms can be expressed in pseudocode or a programming language, and they are used in a variety of fields, such as computer science, engineering, mathematics, and data analysis.
When designing an algorithm, it is important to break down the problem into smaller subproblems, which are easier to solve. Then, each subproblem is solved, and the solutions are combined to produce a final solution for the original problem. The process of breaking down a problem into smaller subproblems is called decomposition, and it is a key aspect of algorithm design.
There are several algorithmic strategies that can be used when designing algorithms. These strategies include brute force, divide and conquer, dynamic programming, and greedy algorithms.
Brute Force: Brute force is the simplest algorithmic strategy, which involves checking every possible solution to a problem. Although this strategy can be effective for small problems, it becomes impractical for larger problems due to its high time and space complexity.
Divide and Conquer: Divide and conquer is an algorithmic strategy that involves breaking down a problem into smaller subproblems, solving each subproblem independently, and then combining the solutions to produce a final solution. This strategy can be highly effective for problems that can be divided into subproblems that are similar in nature.
Dynamic Programming: Dynamic programming is an algorithmic strategy that involves breaking down a problem into smaller subproblems and solving each subproblem only once. This strategy can be highly effective for problems that have overlapping subproblems.
Greedy Algorithms: Greedy algorithms are a class of algorithms that make locally optimal choices at each step in the hope of finding a global optimum. This strategy can be highly effective for problems that can be solved in a greedy manner.
When designing an algorithm, it is also important to consider several factors, including time complexity, space complexity, and correctness. Time complexity refers to the amount of time an algorithm takes to solve a problem, usually expressed in terms of the input size. Space complexity refers to the amount of memory an algorithm requires to solve a problem, also usually expressed in terms of the input size. Correctness refers to whether an algorithm produces the correct output for all possible inputs.
Algorithm analysis is the process of determining the time and space complexity of an algorithm. This involves analyzing the performance of an algorithm as a function of the input size. The goal of algorithm analysis is to identify algorithms that have the best performance characteristics for a given problem.
In summary, algorithm design is a crucial aspect of programming, and understanding the different algorithmic strategies can help in designing efficient algorithms. It is also important to consider time complexity, space complexity, and correctness when designing algorithms. Algorithm analysis is necessary to determine the performance of an algorithm, and it is important to choose the best algorithm for a given problem based on its performance characteristics.
Python Algorithm Analysis
Python algorithm analysis involves analyzing the time and space complexity of algorithms written in the Python programming language. Time complexity refers to the amount of time an algorithm takes to execute, while space complexity refers to the amount of memory an algorithm requires to execute.
There are several tools and techniques available for analyzing Python algorithms, including asymptotic analysis, profiling, and benchmarking.
Asymptotic Analysis: Asymptotic analysis is a technique for analyzing the time complexity of an algorithm. It involves determining the algorithm’s growth rate as the input size increases. The two most commonly used notations for asymptotic analysis are Big O notation and Theta notation.
Big O notation is used to express the upper bound of the algorithm’s time complexity. It provides an estimate of the worst-case scenario for the algorithm’s execution time. Theta notation, on the other hand, provides an estimate of the algorithm’s average-case execution time.
For example, consider the following Python function that calculates the sum of all elements in a list:
def sum_list(lst):
total = 0
for i in lst:
total += i
return total
The time complexity of this function is O(n), where n is the length of the input list. This means that the execution time of the function grows linearly with the input size.
Profiling: Profiling is a technique for analyzing the runtime behavior of an algorithm. It involves measuring the time taken by each line of code in an algorithm and identifying any bottlenecks or performance issues.
Python provides a built-in profiling module called cProfile, which can be used to profile Python programs. cProfile provides detailed information on the number of function calls, the execution time, and the memory usage of each function in a program.
For example, to profile the sum_list() function from the previous example, the following code can be used:
import cProfile
lst = [1, 2, 3, 4, 5]
cProfile.run(‘sum_list(lst)’)
This will output a detailed report on the function’s runtime behavior, including the total number of function calls, the total execution time, and the memory usage.
Benchmarking: Benchmarking is a technique for comparing the performance of different algorithms or implementations. It involves running each algorithm with the same input size and measuring their execution time and memory usage.
Python provides a built-in benchmarking module called timeit, which can be used to benchmark Python programs. timeit provides a simple way to measure the execution time of a small piece of code repeatedly.
For example, to benchmark the sum_list() function from the previous example, the following code can be used:
import timeit
lst = [1, 2, 3, 4, 5]
timeit.timeit(‘sum_list(lst)’, globals=globals(), number=1000000)
This will output the execution time of the function in seconds, averaged over 1,000,000 runs.
In summary, Python algorithm analysis is an important aspect of algorithm design and optimization. Asymptotic analysis, profiling, and benchmarking are all useful techniques for analyzing the time and space complexity of Python algorithms. By analyzing the performance of an algorithm, it is possible to optimize it for better efficiency and scalability.
Python Algorithmic Strategies
Python algorithmic strategies refer to the various techniques and approaches that can be used to design and optimize algorithms in the Python programming language. These strategies include:
Brute Force: The brute force approach involves trying every possible solution to a problem until the correct one is found. While this approach is not always the most efficient, it is often the easiest to implement and can be useful for solving small-scale problems.
For example, consider the problem of finding the maximum value in a list of integers. A brute force solution to this problem would involve iterating over the list and comparing each value to the current maximum value:
def max_value(lst):
max_val = lst[0]
for val in lst:
if val > max_val:
max_val = val
return max_val
While this solution is straightforward, it has a time complexity of O(n), where n is the length of the input list. This means that as the size of the input list grows, the algorithm’s performance will degrade.
Divide and Conquer: The divide and conquer approach involves breaking down a problem into smaller sub-problems, solving each sub-problem independently, and then combining the results to obtain the final solution. This approach is often used for problems that can be broken down into independent parts.
For example, consider the problem of sorting a list of integers. A divide and conquer solution to this problem would involve recursively dividing the list into smaller sub-lists, sorting each sub-list independently, and then merging the sorted sub-lists:
def merge_sort(lst):
if len(lst) <= 1:
return lst
mid = len(lst) // 2
left = merge_sort(lst[:mid])
right = merge_sort(lst[mid:])
return merge(left, right)
def merge(left, right):
result = []
i, j = 0, 0
while i < len(left) and j < len(right):
if left[i] < right[j]:
result.append(left[i])
i += 1
else:
result.append(right[j])
j += 1
result += left[i:]
result += right[j:]
return result
This solution has a time complexity of O(n log n), where n is the length of the input list. This means that as the size of the input list grows, the algorithm’s performance will remain relatively stable.
Dynamic Programming: Dynamic programming is a technique for solving problems by breaking them down into smaller sub-problems and solving each sub-problem only once. The solutions to the sub-problems are then stored and used to solve larger sub-problems until the final solution is obtained.
For example, consider the problem of finding the longest increasing subsequence in a list of integers. A dynamic programming solution to this problem would involve keeping track of the length of the longest increasing subsequence ending at each index in the list:
def longest_increasing_subsequence(lst):
n = len(lst)
dp = [1] * n
for i in range(1, n):
for j in range(i):
if lst[j] < lst[i]:
dp[i] = max(dp[i], dp[j] + 1)
return max(dp)
This solution has a time complexity of O(n^2), where n is the length of the input list. However, there are more efficient algorithms for solving this problem using dynamic programming, such as the O(n log n) Patience sorting algorithm.
Python Algorithmic Libraries
Python provides many powerful algorithmic libraries that can be used to simplify the design and analysis of algorithms. These libraries offer a wide range of functionalities that can be used for tasks such as data analysis, machine learning, scientific computing, and more. In this section, we will discuss some of the most popular Python algorithmic libraries.
NumPy: NumPy is a Python library that is used for scientific computing. It provides a powerful N-dimensional array object that can be used to store and manipulate large arrays of homogeneous data efficiently. NumPy also provides many useful mathematical functions and algorithms that can be used for tasks such as linear algebra, Fourier analysis, and random number generation.
For example, consider the following code that uses NumPy to generate a random 3×3 matrix and compute its inverse:
import numpy as np
# Generate a random 3×3 matrix
A = np.random.rand(3, 3)
# Compute the inverse of the matrix
A_inv = np.linalg.inv(A)
print(A)
print(A_inv)
SciPy: SciPy is a Python library that builds on NumPy and provides additional functionality for scientific computing. It provides a wide range of algorithms for tasks such as optimization, integration, signal processing, and more. SciPy also includes many sub-packages, such as SciPy.stats for statistical analysis, SciPy.spatial for spatial algorithms, and more.
For example, consider the following code that uses SciPy to perform a linear regression on a set of data:
import numpy as np
from scipy import stats
# Generate some sample data
x = np.array([1, 2, 3, 4, 5])
y = np.array([2, 3, 5, 6, 8])
# Perform a linear regression
slope, intercept, r_value, p_value, std_err = stats.linregress(x, y)
print(slope, intercept, r_value, p_value, std_err)
Pandas: Pandas is a Python library that is used for data manipulation and analysis. It provides a powerful DataFrame object that can be used to store and manipulate tabular data efficiently. Pandas also includes many useful algorithms for tasks such as data cleaning, data filtering, data aggregation, and more.
For example, consider the following code that uses Pandas to load a CSV file into a DataFrame and compute some summary statistics:
import pandas as pd
# Load the CSV file into a DataFrame
df = pd.read_csv(“data.csv”)
# Compute some summary statistics
print(df.describe())
Scikit-learn: Scikit-learn is a Python library that is used for machine learning. It provides a wide range of algorithms for tasks such as classification, regression, clustering, and more. Scikit-learn also includes many useful tools for tasks such as model selection, feature extraction, and data preprocessing.
For example, consider the following code that uses Scikit-learn to perform a classification task using the popular Iris dataset:
from sklearn.datasets import load_iris
from sklearn.tree import DecisionTreeClassifier
from sklearn.model_selection import train_test_split
# Load the Iris dataset
iris = load_iris()
# Split the data into training and testing sets
X_train, X_test, y_train, y_test = train_test_split(iris.data, iris.target, test_size=0.2)
# Train a decision tree classifier
clf = DecisionTreeClassifier()
clf.fit(X_train, y_train)
# Evaluate the classifier on the test set
score = clf.score(X_test, y_test)
print(score)
Case Study
One of the most prominent applications of Python algorithmic libraries is in the field of machine learning. For example, consider the case of a company that wants to build a model to predict customer churn. The company has a large dataset of customer information and wants to use machine learning to identify the factors that are most predictive of churn and build a model to predict which customers are most likely to churn in the future.
To accomplish this task, the company can use a combination of Python algorithmic libraries such as Pandas for data manipulation, Scikit-learn for machine learning, and Matplotlib for visualization. With these tools, the company can quickly and efficiently explore the dataset, identify the most important features, train and validate a machine learning model, and visualize the results.
Examples
Here are a few more examples of how Python algorithmic libraries can be used in different applications:
Financial Analysis: Python algorithmic libraries such as NumPy and Pandas can be used to analyze financial data such as stock prices, company financials, and economic indicators. These libraries can help financial analysts to quickly identify trends, patterns, and anomalies in the data and make informed investment decisions.
Image Processing: Python algorithmic libraries such as OpenCV and Pillow can be used for image processing tasks such as image filtering, feature detection, and image recognition. These libraries can help computer vision engineers to build robust image processing pipelines for applications such as object detection, facial recognition, and more.
Natural Language Processing: Python algorithmic libraries such as NLTK and SpaCy can be used for natural language processing tasks such as text classification, sentiment analysis, and named entity recognition. These libraries can help data scientists to extract insights from large volumes of text data and build intelligent text-based applications such as chatbots and virtual assistants.
FAQs
What is the best Python algorithmic library for machine learning?
Scikit-learn is widely considered to be one of the best Python algorithmic libraries for machine learning. It provides a wide range of algorithms and tools for tasks such as classification, regression, clustering, and more.
Can Python algorithmic libraries be used for data analysis?
Yes, Python algorithmic libraries such as NumPy and Pandas are widely used for data analysis. These libraries provide powerful tools for tasks such as data manipulation, data filtering, and data aggregation.
How can I choose the right Python algorithmic library for my project?
Choosing the right Python algorithmic library depends on the specific requirements of your project. Some factors to consider include the type of data you are working with, the complexity of the algorithms you need to use, and the level of customization and control you require.
Are Python algorithmic libraries difficult to learn?
Python algorithmic libraries can be challenging to learn, especially for beginners. However, there are many resources available such as online tutorials, documentation, and community forums that can help you get started. Additionally, many Python algorithmic libraries provide easy-to-use APIs that can simplify the learning process.
Conclusion
Python algorithmic libraries such as Scikit-learn, NumPy, Pandas, OpenCV, NLTK, and SpaCy have become an industry standard and are widely used in many different industries. These libraries have a large and active community of developers, which means that there are many resources available for learning and troubleshooting.
Overall, the versatility and ease of use of Python algorithmic libraries make them a powerful tool for anyone working with data or building intelligent applications. By leveraging these libraries, developers and data scientists can save time and effort, and focus on the creative aspects of their work.
Latest Topic
-
Cloud-Native Technologies: Best Practices
20 April, 2024 -
Generative AI with Llama 3: Shaping the Future
15 April, 2024 -
Mastering Llama 3: The Ultimate Guide
10 April, 2024
Category
- Assignment Help
- Homework Help
- Programming
- Trending Topics
- C Programming Assignment Help
- Art, Interactive, And Robotics
- Networked Operating Systems Programming
- Knowledge Representation & Reasoning Assignment Help
- Digital Systems Assignment Help
- Computer Design Assignment Help
- Artificial Life And Digital Evolution
- Coding and Fundamentals: Working With Collections
- UML Online Assignment Help
- Prolog Online Assignment Help
- Natural Language Processing Assignment Help
- Julia Assignment Help
- Golang Assignment Help
- Design Implementation Of Network Protocols
- Computer Architecture Assignment Help
- Object-Oriented Languages And Environments
- Coding Early Object and Algorithms: Java Coding Fundamentals
- Deep Learning In Healthcare Assignment Help
- Geometric Deep Learning Assignment Help
- Models Of Computation Assignment Help
- Systems Performance And Concurrent Computing
- Advanced Security Assignment Help
- Typescript Assignment Help
- Computational Media Assignment Help
- Design And Analysis Of Algorithms
- Geometric Modelling Assignment Help
- JavaScript Assignment Help
- MySQL Online Assignment Help
- Programming Practicum Assignment Help
- Public Policy, Legal, And Ethical Issues In Computing, Privacy, And Security
- Computer Vision
- Advanced Complexity Theory Assignment Help
- Big Data Mining Assignment Help
- Parallel Computing And Distributed Computing
- Law And Computer Science Assignment Help
- Engineering Distributed Objects For Cloud Computing
- Building Secure Computer Systems Assignment Help
- Ada Assignment Help
- R Programming Assignment Help
- Oracle Online Assignment Help
- Languages And Automata Assignment Help
- Haskell Assignment Help
- Economics And Computation Assignment Help
- ActionScript Assignment Help
- Audio Programming Assignment Help
- Bash Assignment Help
- Computer Graphics Assignment Help
- Groovy Assignment Help
- Kotlin Assignment Help
- Object Oriented Languages And Environments
- COBOL ASSIGNMENT HELP
- Bayesian Statistical Probabilistic Programming
- Computer Network Assignment Help
- Django Assignment Help
- Lambda Calculus Assignment Help
- Operating System Assignment Help
- Computational Learning Theory
- Delphi Assignment Help
- Concurrent Algorithms And Data Structures Assignment Help
- Machine Learning Assignment Help
- Human Computer Interface Assignment Help
- Foundations Of Data Networking Assignment Help
- Continuous Mathematics Assignment Help
- Compiler Assignment Help
- Computational Biology Assignment Help
- PostgreSQL Online Assignment Help
- Lua Assignment Help
- Human Computer Interaction Assignment Help
- Ethics And Responsible Innovation Assignment Help
- Communication And Ethical Issues In Computing
- Computer Science
- Combinatorial Optimisation Assignment Help
- Ethical Computing In Practice
- HTML Homework Assignment Help
- Linear Algebra Assignment Help
- Perl Assignment Help
- Artificial Intelligence Assignment Help
- Uncategorized
- Ethics And Professionalism Assignment Help
- Human Augmentics Assignment Help
- Linux Assignment Help
- PHP Assignment Help
- Assembly Language Assignment Help
- Dart Assignment Help
- Complete Python Bootcamp From Zero To Hero In Python Corrected Version
- Swift Assignment Help
- Computational Complexity Assignment Help
- Probability And Computing Assignment Help
- MATLAB Programming For Engineers
- Introduction To Statistical Learning
- Database Systems Implementation Assignment Help
- Computational Game Theory Assignment Help
- Database Assignment Help
- Probabilistic Model Checking Assignment Help
- Mathematics For Computer Science And Philosophy
- Introduction To Formal Proof Assignment Help
- Creative Coding Assignment Help
- Foundations Of Self-Programming Agents Assignment Help
- Machine Organization Assignment Help
- Software Design Assignment Help
- Data Communication And Networking Assignment Help
- Computational Biology
- Data Structure Assignment Help
- Foundations Of Software Engineering Assignment Help
- Mathematical Foundations Of Computing
- Principles Of Programming Languages Assignment Help
- Software Engineering Capstone Assignment Help
- Algorithms and Data Structures Assignment Help
No Comments