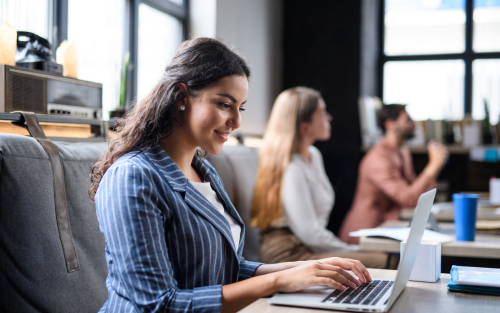
12 May Python Coding Help
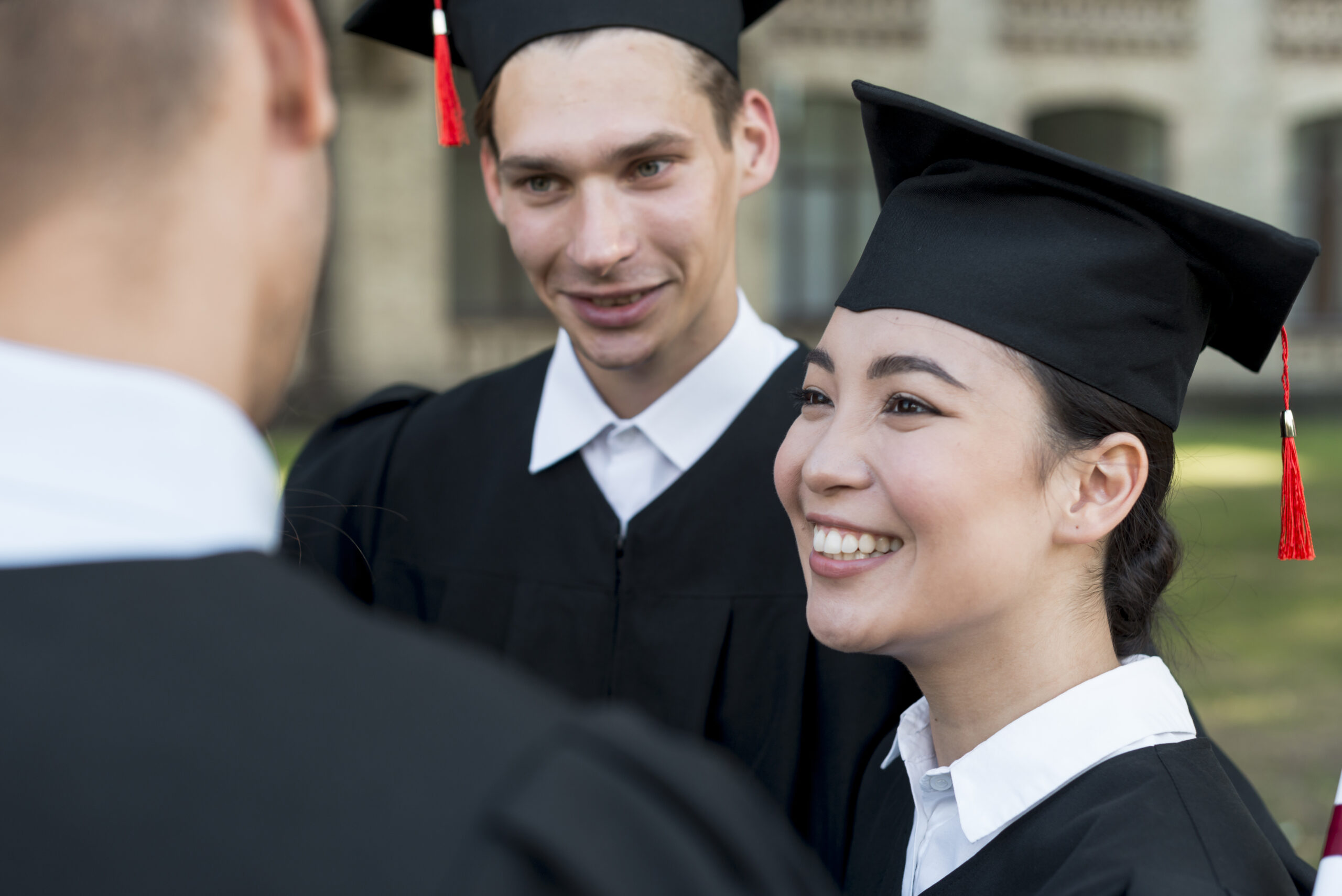
Introduction
Python is a high-level programming language that has become increasingly popular due to its simplicity, versatility, and ease of use. Python is used in a wide range of applications, from web development to scientific computing and machine learning. Despite its widespread adoption, however, many people still find programming in Python to be a challenge. Whether you’re a beginner or an experienced programmer, there are times when you may need Python coding help. In this article, we’ll cover some of the most common issues people face when coding in Python and provide tips for overcoming them.
Understanding Syntax Errors
Syntax errors are one of the most common issues people face when coding in Python. These occur when the interpreter encounters code that violates the rules of the language. Syntax errors can be caused by a variety of mistakes, such as typos, missing parentheses or quotes, or using the wrong type of operator.
One of the best ways to avoid syntax errors is to familiarize yourself with the basic syntax of Python. This includes understanding the use of whitespace, parentheses, and quotes. For example, Python uses indentation to delimit blocks of code, so it’s important to make sure that your code is properly indented.
It’s also a good idea to use a code editor or integrated development environment (IDE) that provides syntax highlighting and error checking. This can help you catch syntax errors before you even run your code. For example, many code editors will highlight syntax errors in red and provide suggestions for how to fix them.
When you encounter a syntax error, Python will usually provide an error message that includes the line number and a description of the error. For example, if you forget to close a quote in a string, Python might give you an error message like this:
SyntaxError: EOL while scanning string literal
This error message indicates that the end of the string was encountered while scanning for the end of the line (EOL).
To fix syntax errors, it’s important to carefully review your code and look for any mistakes. This may involve checking your spelling, making sure that you’re using the correct syntax for operators and statements, and verifying that your code is properly indented. It’s also a good idea to test your code frequently as you write it, as this can help you catch syntax errors early on.
Debugging Your Code
Debugging is the process of identifying and fixing errors in your code. It’s a crucial skill for any programmer, as even the most experienced developers make mistakes. When you encounter an error in your code, it’s important to take a systematic approach to debugging.
The first step in debugging your code is to identify the problem. This can be done by examining error messages, using print statements to check the value of variables, and stepping through your code line by line using a debugger. The goal is to isolate the problem and determine its root cause.
Once you’ve identified the problem, you can start to fix it. This may involve rewriting a section of code, changing the order of operations, or using a different data structure. It’s important to be patient and methodical when debugging your code, and to test your changes thoroughly to make sure that they don’t introduce new errors.
Here are some tips for effective debugging:
Use print statements: One of the simplest ways to debug your code is to use print statements to check the value of variables at different points in your code. This can help you identify where the problem is occurring and what might be causing it.
Use a debugger: A debugger is a tool that allows you to step through your code line by line and examine the value of variables at each step. This can be a powerful way to identify errors and understand how your code is executing.
Read error messages carefully: When you encounter an error message, read it carefully to understand what the problem is. The error message will often provide clues about where the problem is occurring and what might be causing it.
Test your code frequently: It’s important to test your code frequently as you write it, as this can help you catch errors early on. You should also test your code thoroughly after making changes to ensure that they don’t introduce new errors.
Don’t be afraid to ask for help: Debugging can be a frustrating process, and it’s not always easy to solve problems on your own. Don’t be afraid to ask for help from more experienced programmers or online communities.
Take breaks: Debugging can be mentally taxing, so it’s important to take breaks and step away from your code when you’re feeling frustrated or stuck. This can help you come back to the problem with fresh eyes and a renewed sense of focus.
Understanding Object-Oriented Programming (OOP)
Object-oriented programming (OOP) is a programming paradigm that is based on the concept of objects. An object is an instance of a class, which is a blueprint for creating objects with a specific set of properties and behaviors.
The key concepts of OOP include:
Encapsulation: Encapsulation is the process of hiding implementation details and exposing only the necessary information. In OOP, objects have attributes (properties) and methods (behaviors), which are used to encapsulate data and functionality.
Inheritance: Inheritance is the process of creating a new class from an existing class. The new class inherits all the properties and behaviors of the existing class, and can also add new properties and behaviors of its own.
Polymorphism: Polymorphism is the ability of objects to take on different forms. In OOP, polymorphism is achieved through method overloading and method overriding. Method overloading is when multiple methods have the same name but different parameters, while method overriding is when a subclass provides a different implementation of a method that is already defined in its superclass.
Abstraction: Abstraction is the process of reducing complexity by hiding unnecessary details. In OOP, abstraction is achieved through abstract classes and interfaces, which provide a common interface for a set of related classes.
OOP is a popular programming paradigm because it allows for modular, reusable, and maintainable code. By encapsulating data and functionality in objects, code can be organized more efficiently and reused across multiple projects. Inheritance and polymorphism also allow for more flexible and extensible code, as new classes can be created from existing classes and methods can be overridden to provide different functionality.
Some examples of OOP languages include Java, C++, and Python. In Python, classes are defined using the class keyword and objects are created using the class constructor. Here is an example of a simple class in Python:
class Car:
def __init__(self, make, model, year):
self.make = make
self.model = model
self.year = year
def start(self):
print(“The car has started.”)
def stop(self):
print(“The car has stopped.”)
In this example, the Car class has three attributes (make, model, and year) and two methods (start and stop). Objects of this class can be created by calling the constructor and passing in values for the make, model, and year attributes:
my_car = Car("Toyota", "Corolla", 2021)
Once an object has been created, its methods can be called using dot notation:
my_car.start() # prints "The car has started."
my_car.stop() # prints "The car has stopped."
Using Libraries and Modules
What are Libraries and Modules?
A library is a collection of pre-written code that can be used by other programs. Libraries often contain modules, which are smaller units of code that perform a specific task. For example, the Python Standard Library contains many modules that provide common functionality such as file input/output, networking, and regular expressions.
Modules are essentially just Python files that contain functions, classes, and variables. They can be imported into other Python scripts to make use of their functionality. Modules can be created by anyone and can be shared and reused by others.
How to Use Libraries and Modules in Python
Python makes it easy to use libraries and modules. To use a library or module, you first need to import it into your Python script. There are several ways to do this:
Importing a module by name:
import math
This will import the math module, which contains many mathematical functions.
Importing a module and giving it a different name:
import math as m
This will import the math module, but give it the name “m” instead of “math”.
Importing specific functions from a module:
from math import sqrt
This will import only the sqrt function from the math module.
Once a module has been imported, you can use its functions and variables in your code. For example, to use the sqrt function from the math module:
import math
x = math.sqrt(25)
print(x)
This will output “5.0”.
Tips for Using Libraries and Modules
Read the Documentation: When using a library or module, it’s important to read the documentation to understand how to use it correctly. Most libraries and modules have extensive documentation that explains how to use their functionality and provides examples.
Check Dependencies: Some libraries and modules may have dependencies on other libraries or modules. Make sure you have all the required dependencies installed before using a library or module.
Use Virtual Environments: Virtual environments allow you to create a separate environment for each project, with its own set of dependencies. This can help prevent conflicts between different projects and make it easier to manage dependencies.
Be Selective: While libraries and modules can save time and improve code quality, it’s important to be selective about which ones to use. Using too many libraries and modules can make your code harder to maintain and understand, and can also increase the risk of conflicts and compatibility issues.
Case Studies
Pandas Library: Pandas is a popular library for data manipulation and analysis in Python. It provides data structures and functions for working with structured data, such as data frames and series. Many data scientists and analysts use Pandas for data cleaning, exploration, and visualization.
TensorFlow Library: TensorFlow is an open-source machine learning library developed by Google. It provides functions and tools for building and training machine learning models, including deep learning models. TensorFlow is widely used in industry and academia for various applications, such as image recognition, natural language processing, and robotics.
Examples
Using the random module to generate random numbers:
import random
# Generate a random integer between 0 and 9
x = random.randint(0, 9)
print(x)
# Generate a random float between 0 and 1
y = random.random()
print(y)
Using the requests module to make HTTP requests:
import requests
# Make a GET request to a URL
response = requests.get(‘https://www.google.com’)
print(response.status_code)
print(response.content)
FAQs
Q: What is the difference between a library and a module?
A: A library is a collection of related modules, while a module is a single file containing Python code.
Q: How do I know which library or module to use for a specific task?
A: It’s best to research and compare different libraries and modules based on their documentation, community support, performance, and ease of use. You can also ask for recommendations from experienced Python developers.
Q: Can I create my own modules and libraries in Python?
A: Yes, you can create your own modules and libraries in Python by organizing your code into reusable functions, classes, and variables, and packaging them into modules and libraries that can be imported into other Python scripts. There are also tools and frameworks, such as setuptools and PyPI, that can help you distribute and share your Python packages.
Q: What are some best practices for using libraries and modules in Python?
A: Some best practices include reading the documentation, checking dependencies, using virtual environments, being selective about which libraries and modules to use, and updating them regularly to ensure security and compatibility. It’s also important to follow the PEP 8 style guide for Python code, which includes guidelines for importing and using modules and libraries.
Conclusion
In conclusion, using libraries and modules is an essential aspect of programming in Python. Libraries and modules provide pre-written code that can save time and effort, increase efficiency, and improve the overall quality of the code. They can be used for a wide range of tasks, such as data analysis, machine learning, web development, and more. It’s important to research and compare different libraries and modules based on their functionality, documentation, community support, and performance before incorporating them into your code. By following best practices and staying up-to-date with the latest developments in the Python ecosystem, developers can leverage the power of libraries and modules to build robust and scalable applications.
Latest Topic
-
Cloud-Native Technologies: Best Practices
20 April, 2024 -
Generative AI with Llama 3: Shaping the Future
15 April, 2024 -
Mastering Llama 3: The Ultimate Guide
10 April, 2024
Category
- Assignment Help
- Homework Help
- Programming
- Trending Topics
- C Programming Assignment Help
- Art, Interactive, And Robotics
- Networked Operating Systems Programming
- Knowledge Representation & Reasoning Assignment Help
- Digital Systems Assignment Help
- Computer Design Assignment Help
- Artificial Life And Digital Evolution
- Coding and Fundamentals: Working With Collections
- UML Online Assignment Help
- Prolog Online Assignment Help
- Natural Language Processing Assignment Help
- Julia Assignment Help
- Golang Assignment Help
- Design Implementation Of Network Protocols
- Computer Architecture Assignment Help
- Object-Oriented Languages And Environments
- Coding Early Object and Algorithms: Java Coding Fundamentals
- Deep Learning In Healthcare Assignment Help
- Geometric Deep Learning Assignment Help
- Models Of Computation Assignment Help
- Systems Performance And Concurrent Computing
- Advanced Security Assignment Help
- Typescript Assignment Help
- Computational Media Assignment Help
- Design And Analysis Of Algorithms
- Geometric Modelling Assignment Help
- JavaScript Assignment Help
- MySQL Online Assignment Help
- Programming Practicum Assignment Help
- Public Policy, Legal, And Ethical Issues In Computing, Privacy, And Security
- Computer Vision
- Advanced Complexity Theory Assignment Help
- Big Data Mining Assignment Help
- Parallel Computing And Distributed Computing
- Law And Computer Science Assignment Help
- Engineering Distributed Objects For Cloud Computing
- Building Secure Computer Systems Assignment Help
- Ada Assignment Help
- R Programming Assignment Help
- Oracle Online Assignment Help
- Languages And Automata Assignment Help
- Haskell Assignment Help
- Economics And Computation Assignment Help
- ActionScript Assignment Help
- Audio Programming Assignment Help
- Bash Assignment Help
- Computer Graphics Assignment Help
- Groovy Assignment Help
- Kotlin Assignment Help
- Object Oriented Languages And Environments
- COBOL ASSIGNMENT HELP
- Bayesian Statistical Probabilistic Programming
- Computer Network Assignment Help
- Django Assignment Help
- Lambda Calculus Assignment Help
- Operating System Assignment Help
- Computational Learning Theory
- Delphi Assignment Help
- Concurrent Algorithms And Data Structures Assignment Help
- Machine Learning Assignment Help
- Human Computer Interface Assignment Help
- Foundations Of Data Networking Assignment Help
- Continuous Mathematics Assignment Help
- Compiler Assignment Help
- Computational Biology Assignment Help
- PostgreSQL Online Assignment Help
- Lua Assignment Help
- Human Computer Interaction Assignment Help
- Ethics And Responsible Innovation Assignment Help
- Communication And Ethical Issues In Computing
- Computer Science
- Combinatorial Optimisation Assignment Help
- Ethical Computing In Practice
- HTML Homework Assignment Help
- Linear Algebra Assignment Help
- Perl Assignment Help
- Artificial Intelligence Assignment Help
- Uncategorized
- Ethics And Professionalism Assignment Help
- Human Augmentics Assignment Help
- Linux Assignment Help
- PHP Assignment Help
- Assembly Language Assignment Help
- Dart Assignment Help
- Complete Python Bootcamp From Zero To Hero In Python Corrected Version
- Swift Assignment Help
- Computational Complexity Assignment Help
- Probability And Computing Assignment Help
- MATLAB Programming For Engineers
- Introduction To Statistical Learning
- Database Systems Implementation Assignment Help
- Computational Game Theory Assignment Help
- Database Assignment Help
- Probabilistic Model Checking Assignment Help
- Mathematics For Computer Science And Philosophy
- Introduction To Formal Proof Assignment Help
- Creative Coding Assignment Help
- Foundations Of Self-Programming Agents Assignment Help
- Machine Organization Assignment Help
- Software Design Assignment Help
- Data Communication And Networking Assignment Help
- Computational Biology
- Data Structure Assignment Help
- Foundations Of Software Engineering Assignment Help
- Mathematical Foundations Of Computing
- Principles Of Programming Languages Assignment Help
- Software Engineering Capstone Assignment Help
- Algorithms and Data Structures Assignment Help
No Comments