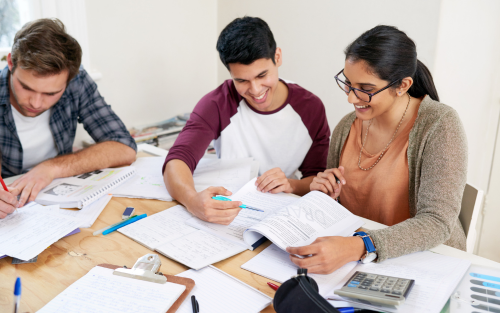
02 May Tips For Writing More Robust Javascript Code
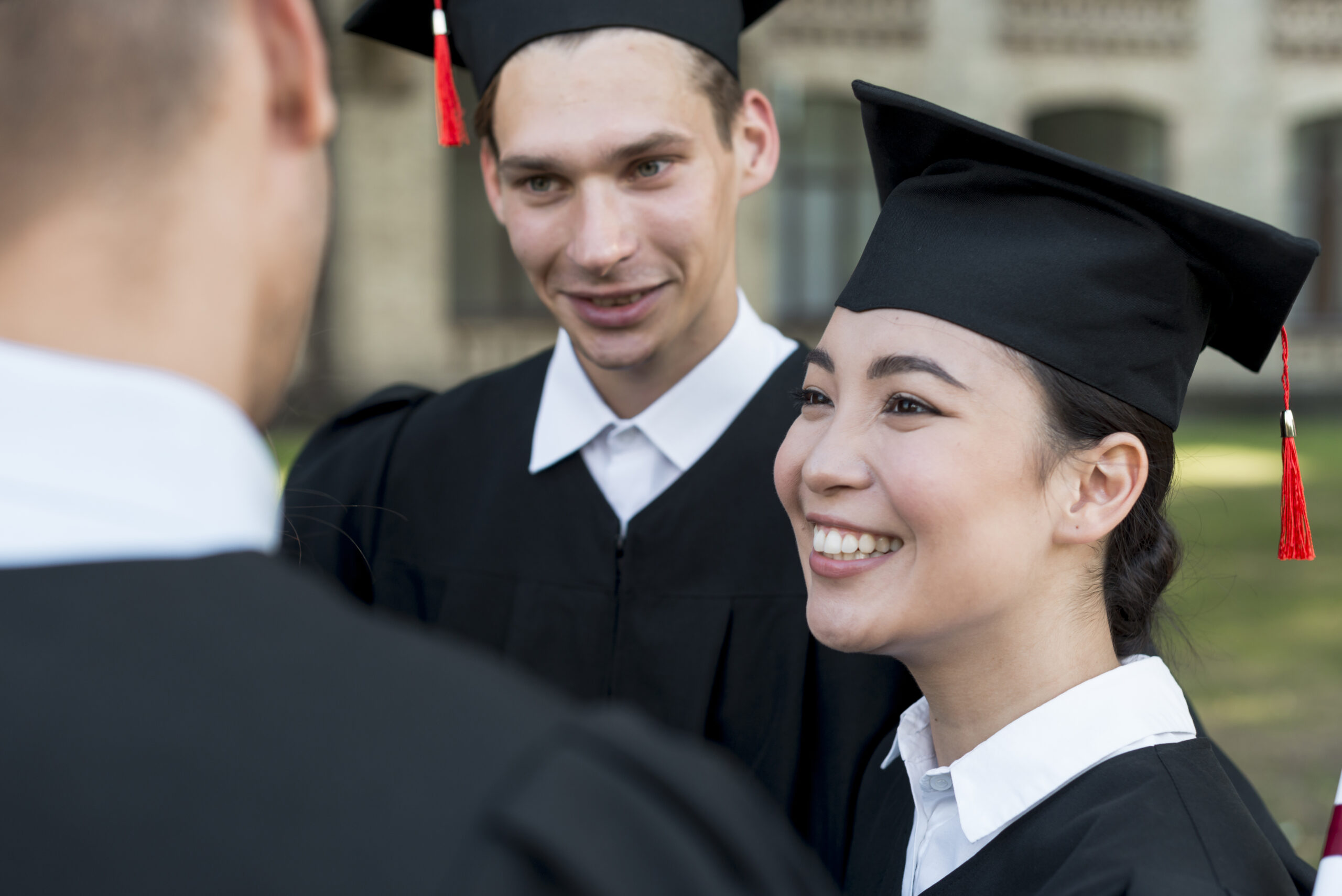
JavaScript is a powerful language that allows developers to create interactive and dynamic web applications. However, with great power comes great responsibility. As the complexity of JavaScript applications grows, it becomes increasingly important to write robust code that can handle errors and edge cases. In this article, we will share some tips for writing more robust JavaScript code that is easier to maintain and debug.
Improve the reliability and maintainability of your JavaScript code with these valuable tips for writing more robust JavaScript applications. Follow best practices such as enabling strict mode, adhering to coding conventions, validating input data, handling errors gracefully, and optimizing code performance. Minimize the use of global variables, practice defensive programming, implement testing, and regularly refactor your code for better robustness. These tips will help you create JavaScript code that is less prone to errors, easier to maintain, and delivers a seamless user experience.
Use Strict Mode
One of the simplest and most effective ways to write more robust JavaScript code is to use strict mode. Strict mode is a feature introduced in ECMAScript 5 that enforces stricter rules for JavaScript syntax and behavior. By using strict mode, you can catch common errors that may go unnoticed in non-strict mode.
To enable strict mode, simply add the following line at the beginning of your JavaScript file:
'use strict';
This will enable strict mode for the entire file and enforce stricter rules for variable declaration, function invocation, and other common JavaScript operations.
Use Meaningful Variable Names
When writing JavaScript code, it is important to use meaningful variable names that accurately describe the purpose of the variable. This not only makes your code easier to understand for other developers, but also makes it easier to debug and maintain.
Avoid using single-letter variable names or abbreviations that are difficult to understand. Instead, use descriptive names that accurately convey the purpose of the variable. For example, use userInput
instead of input
, or errorMessage
instead of err
.
Avoid Global Variables
Global variables can be convenient, but they can also cause issues in larger applications. Global variables can be modified by any part of the code, which can lead to unexpected behavior and hard-to-debug issues.
Instead of using global variables, use local variables and pass them as arguments to functions. This will make your code more modular and easier to test, and will also reduce the risk of naming conflicts and unexpected behavior.
Use Error Handling
Error handling is an essential part of writing robust JavaScript code. By handling errors, you can prevent your application from crashing and provide a better user experience. Use try-catch blocks to catch errors and handle them gracefully.
For example, instead of allowing an error to crash your application, you can display an error message to the user or log the error to the console for debugging purposes.
Use Comments
Comments are a powerful tool for documenting your code and making it more readable. Use comments to explain the purpose of your code, describe what it does, and provide context for other developers.
Comments can also be used to temporarily disable code that is causing issues or to leave notes for future developers. However, be careful not to overuse comments, as too many comments can make your code harder to read.
Use ES6 Features
ES6 introduced many new features and syntax improvements that can make your code more robust and easier to maintain. Some of the most useful features include:
Arrow functions: provide a more concise syntax for declaring functions
Template literals: provide a more concise syntax for string interpolation
Let and const: provide block scope for variables, which can reduce the risk of naming conflicts and unexpected behavior
By using these and other ES6 features, you can write more modern and maintainable JavaScript code.
Test Your Code
Testing is an essential part of writing robust JavaScript code. By testing your code, you can catch errors and edge cases before they cause issues in production. Use tools like Jest, Mocha, or Jasmine to write automated tests for your code.
Automated tests can help you catch errors and edge cases that may go unnoticed during manual testing. Use test-driven development (TDD) to write tests before you write your code, which can help you write more modular and maintainable code.
Use a Linter
There are many different linters available for JavaScript, but some of the most popular include ESLint, JSLint, and JSHint. Each of these linters has its own set of rules and conventions, so it’s important to choose one that matches your development style and preferences.
Once you’ve installed a linter, you can configure it to enforce a specific set of rules and conventions for your code. This might include things like enforcing consistent indentation, requiring semicolons at the end of lines, and prohibiting the use of certain language features that are known to be problematic.
By using a linter, you can catch many common errors and issues in your code before they cause problems in production. This can save you time and effort in the long run, and help you produce more robust and reliable code.
Handle Errors Gracefully
Another important tip for writing more robust JavaScript code is to handle errors gracefully. JavaScript code can encounter errors for many different reasons, including network problems, unexpected user input, and unexpected system behavior.
When an error occurs, it’s important to handle it gracefully so that your application can recover gracefully and continue functioning properly. This might involve displaying an error message to the user, logging the error to a file or console, or taking other appropriate actions.
One way to handle errors gracefully is to use try-catch blocks to catch and handle exceptions. A try-catch block is a code construct that allows you to specify a block of code that may generate an exception, and a separate block of code that should be executed if an exception occurs.
For example, suppose you have a function that attempts to load data from a remote server using an AJAX request. If the request fails for some reason, you can use a try-catch block to catch the exception and display an error message to the user.
Here’s an example:
function loadData() {
try {
// Attempt to load data from the server
$.ajax({
url: '/data',
success: function(data) {
// Do something with the data
},
error: function(xhr, status, error) {
// Handle the error gracefully
showError('Failed to load data: ' + error);
}
});
} catch (ex) {
// Handle any other exceptions that might occur
showError('An unexpected error occurred: ' + ex.message);
}
}
function showError(message) {
// Display an error message to the user
alert(message);
}
In this example, the loadData
function attempts to load data from the server using an AJAX request. If the request fails, the error callback is executed, which calls the showError
function to display an error message to the user.
The try-catch block ensures that any exceptions that might occur during the AJAX request are caught and handled gracefully. If any other unexpected exceptions occur, they will be caught by the catch block and handled in a similar way.
Use Defensive Programming Techniques
Defensive programming is a technique that involves writing code that is designed to handle unexpected or invalid inputs and conditions. The goal of defensive programming is to prevent errors and exceptions from occurring by checking for and handling potential issues before they can cause problems.
One example of defensive programming is input validation. When writing a function that takes input from a user or external source, it’s important to validate that input to ensure that it meets the expected criteria.
FAQs
What does it mean to write robust JavaScript code?
Writing robust JavaScript code means creating code that is reliable, resilient, and able to handle various scenarios and edge cases without unexpected errors or failures. Robust code is less prone to bugs, easier to maintain, and performs well in different environments.
What are some general tips for writing more robust JavaScript code?
- Use descriptive variable and function names: Choose meaningful and self-explanatory names that accurately describe the purpose and functionality of your code elements.
- Follow coding conventions: Adhere to consistent coding style guidelines, such as indentation, naming conventions, and code organization, to improve code readability and maintainability.
- Validate input data: Validate user input or data from external sources to ensure it meets the expected format and prevent potential security vulnerabilities or runtime errors.
- Handle errors gracefully: Use try-catch blocks to catch and handle exceptions, providing appropriate error messages or fallback mechanisms to avoid abrupt crashes or unexpected behavior.
- Use strict mode: Enable strict mode in your JavaScript code to enforce stricter rules, catch common programming mistakes, and prevent the use of potentially problematic features.
- Modularize your code: Break down your code into reusable and independent modules or functions, promoting code reusability and making it easier to test and maintain.
- Comment your code: Add clear and concise comments to explain complex or non-obvious sections of your code, making it easier for others (including your future self) to understand the logic and purpose of the code.
- Test your code: Write automated tests to validate the behavior of your code, covering different scenarios and edge cases. Testing helps catch bugs early and ensures that your code behaves as expected.
- Use linting and static analysis tools: Employ tools like ESLint or JSLint to enforce coding standards, catch common errors, and identify potential issues in your JavaScript code.
How can I prevent common security vulnerabilities in my JavaScript code?
To prevent common security vulnerabilities:
- Sanitize user input to prevent code injection attacks.
- Validate and sanitize data on the server-side to ensure data integrity.
- Avoid storing sensitive data in plain text or using weak encryption methods.
- Implement proper authentication and authorization mechanisms to protect against unauthorized access.
- Regularly update your dependencies to address known security vulnerabilities.
How can I optimize the performance of my JavaScript code?
- Minimize DOM manipulation and use efficient selectors when working with HTML elements.
- Cache frequently used values or function results to avoid redundant computations.
- Use efficient algorithms and data structures to optimize time and space complexity.
- Reduce network requests by bundling and minifying your code.
- Implement debouncing or throttling techniques for expensive operations triggered by user actions.
- Leverage browser caching and compression techniques to optimize network performance.
Are there any recommended tools or libraries for writing robust JavaScript code?
There are several tools and libraries that can assist you in writing robust JavaScript code, including:
- ESLint: A widely used JavaScript linter that helps enforce coding standards and catch common errors.
- Prettier: A code formatter that automatically formats your JavaScript code to ensure consistency and readability.
- Jest or Mocha: Popular testing frameworks for writing automated tests for your JavaScript code.
- Lodash: A utility library that provides helpful functions for working with arrays, objects, and other data types, improving code quality and readability.
- How can I handle asynchronous operations effectively in JavaScript?
- To handle asynchronous operations effectively:
- Use Promises or async/await syntax to handle asynchronous code flows and avoid callback hell.
- Handle errors properly by using try-catch blocks or Promise error handling mechanisms.
- Utilize asynchronous libraries or functions provided by frameworks like Axios or the built-in Fetch API for network requests.
- Be aware of race conditions and synchronization issues when dealing with shared resources or concurrent operations.
What are some common pitfalls to avoid when writing JavaScript code?
- Using global variables excessively, which can lead to naming conflicts and unexpected behavior.
- Ignoring memory leaks and not properly managing resources.
- Relying too heavily on string manipulation instead of using proper data structures or regular expressions.
- Overcomplicating code logic by nesting too many conditional statements.
- Neglecting error handling and not providing meaningful error messages or fallback mechanisms.
- Failing to handle backward compatibility for older browsers or unsupported JavaScript features.
- Not optimizing code for performance, resulting in slow execution or excessive memory usage.
Conclusion
Some of the tips discussed in this article include using descriptive variable and function names, following a consistent code style, handling errors and edge cases effectively, writing efficient and optimized code, using built-in methods and libraries, applying code testing and debugging techniques, and using a linter to catch common errors and enforce code standards.
By implementing these tips and techniques, developers can ensure that their JavaScript code is more robust, reliable, and efficient, ultimately leading to better user experiences and more successful software projects.
Latest Topic
-
Cloud-Native Technologies: Best Practices
20 April, 2024 -
Generative AI with Llama 3: Shaping the Future
15 April, 2024 -
Mastering Llama 3: The Ultimate Guide
10 April, 2024
Category
- Assignment Help
- Homework Help
- Programming
- Trending Topics
- C Programming Assignment Help
- Art, Interactive, And Robotics
- Networked Operating Systems Programming
- Knowledge Representation & Reasoning Assignment Help
- Digital Systems Assignment Help
- Computer Design Assignment Help
- Artificial Life And Digital Evolution
- Coding and Fundamentals: Working With Collections
- UML Online Assignment Help
- Prolog Online Assignment Help
- Natural Language Processing Assignment Help
- Julia Assignment Help
- Golang Assignment Help
- Design Implementation Of Network Protocols
- Computer Architecture Assignment Help
- Object-Oriented Languages And Environments
- Coding Early Object and Algorithms: Java Coding Fundamentals
- Deep Learning In Healthcare Assignment Help
- Geometric Deep Learning Assignment Help
- Models Of Computation Assignment Help
- Systems Performance And Concurrent Computing
- Advanced Security Assignment Help
- Typescript Assignment Help
- Computational Media Assignment Help
- Design And Analysis Of Algorithms
- Geometric Modelling Assignment Help
- JavaScript Assignment Help
- MySQL Online Assignment Help
- Programming Practicum Assignment Help
- Public Policy, Legal, And Ethical Issues In Computing, Privacy, And Security
- Computer Vision
- Advanced Complexity Theory Assignment Help
- Big Data Mining Assignment Help
- Parallel Computing And Distributed Computing
- Law And Computer Science Assignment Help
- Engineering Distributed Objects For Cloud Computing
- Building Secure Computer Systems Assignment Help
- Ada Assignment Help
- R Programming Assignment Help
- Oracle Online Assignment Help
- Languages And Automata Assignment Help
- Haskell Assignment Help
- Economics And Computation Assignment Help
- ActionScript Assignment Help
- Audio Programming Assignment Help
- Bash Assignment Help
- Computer Graphics Assignment Help
- Groovy Assignment Help
- Kotlin Assignment Help
- Object Oriented Languages And Environments
- COBOL ASSIGNMENT HELP
- Bayesian Statistical Probabilistic Programming
- Computer Network Assignment Help
- Django Assignment Help
- Lambda Calculus Assignment Help
- Operating System Assignment Help
- Computational Learning Theory
- Delphi Assignment Help
- Concurrent Algorithms And Data Structures Assignment Help
- Machine Learning Assignment Help
- Human Computer Interface Assignment Help
- Foundations Of Data Networking Assignment Help
- Continuous Mathematics Assignment Help
- Compiler Assignment Help
- Computational Biology Assignment Help
- PostgreSQL Online Assignment Help
- Lua Assignment Help
- Human Computer Interaction Assignment Help
- Ethics And Responsible Innovation Assignment Help
- Communication And Ethical Issues In Computing
- Computer Science
- Combinatorial Optimisation Assignment Help
- Ethical Computing In Practice
- HTML Homework Assignment Help
- Linear Algebra Assignment Help
- Perl Assignment Help
- Artificial Intelligence Assignment Help
- Uncategorized
- Ethics And Professionalism Assignment Help
- Human Augmentics Assignment Help
- Linux Assignment Help
- PHP Assignment Help
- Assembly Language Assignment Help
- Dart Assignment Help
- Complete Python Bootcamp From Zero To Hero In Python Corrected Version
- Swift Assignment Help
- Computational Complexity Assignment Help
- Probability And Computing Assignment Help
- MATLAB Programming For Engineers
- Introduction To Statistical Learning
- Database Systems Implementation Assignment Help
- Computational Game Theory Assignment Help
- Database Assignment Help
- Probabilistic Model Checking Assignment Help
- Mathematics For Computer Science And Philosophy
- Introduction To Formal Proof Assignment Help
- Creative Coding Assignment Help
- Foundations Of Self-Programming Agents Assignment Help
- Machine Organization Assignment Help
- Software Design Assignment Help
- Data Communication And Networking Assignment Help
- Computational Biology
- Data Structure Assignment Help
- Foundations Of Software Engineering Assignment Help
- Mathematical Foundations Of Computing
- Principles Of Programming Languages Assignment Help
- Software Engineering Capstone Assignment Help
- Algorithms and Data Structures Assignment Help
No Comments