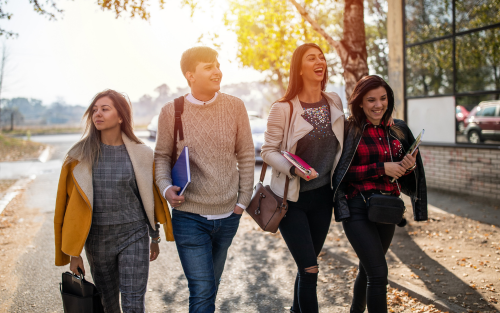
26 Apr Understanding Asynchronous JavaScript
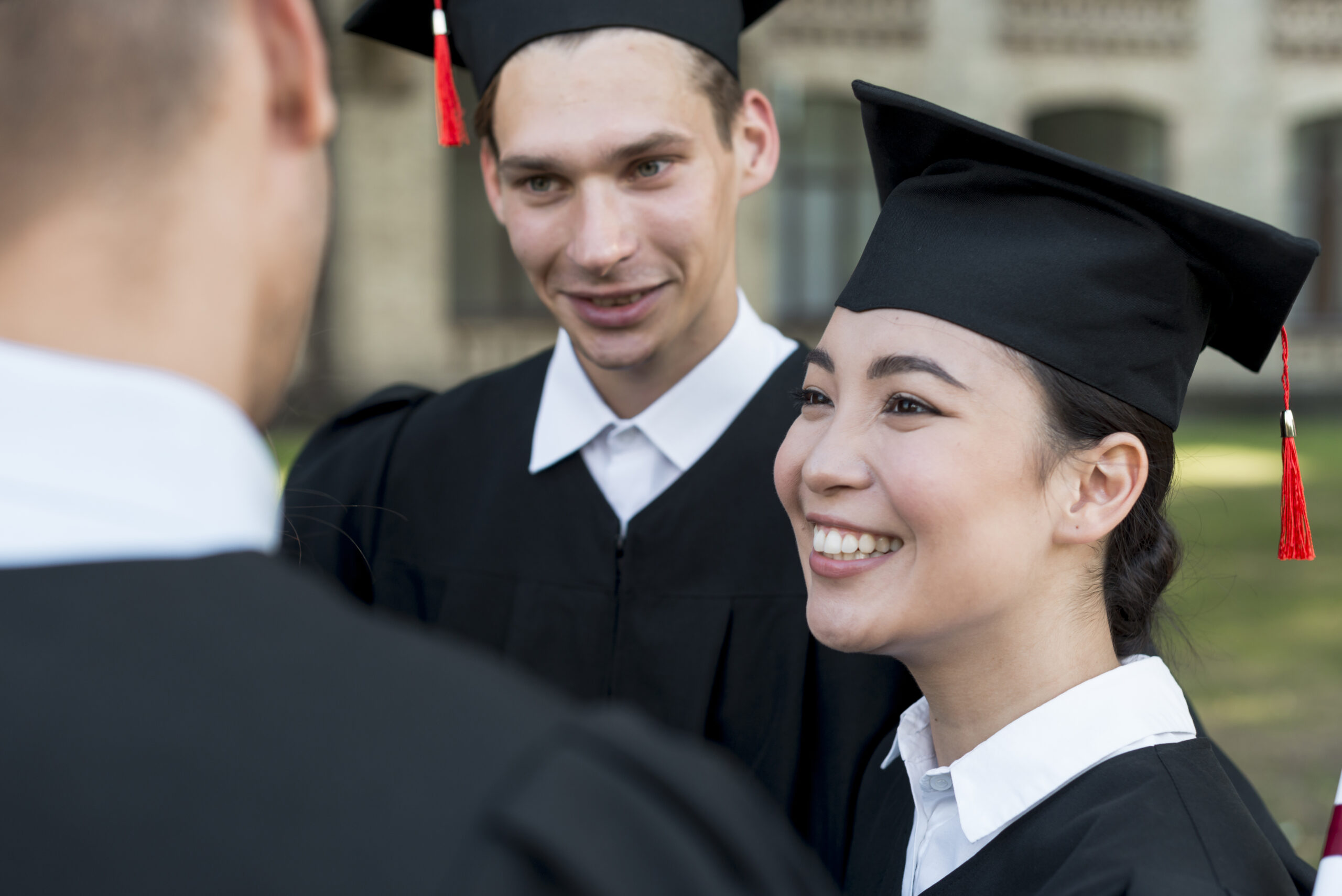
Asynchronous JavaScript is a programming concept that allows the execution of code without blocking the main thread. In simpler terms, asynchronous JavaScript is a way of writing code that allows the program to continue executing while waiting for a response from an external API or function call.
In traditional synchronous programming, when a function is called, the program waits for the function to complete before moving on to the next line of code. This can be problematic when the function takes a long time to execute, as it blocks the main thread, making the application slow and unresponsive.
Asynchronous programming solves this problem by executing functions in the background while the program continues to execute the main thread. When the function has finished executing, it sends a signal to the program, which then continues with the next line of code.
Asynchronous programming is particularly useful when working with web applications that need to make API calls or handle user input. With asynchronous programming, the program can continue to execute while waiting for a response from the API or user input.
JavaScript provides several features for writing asynchronous code, including:
Callbacks: Callbacks are functions that are passed as arguments to other functions and are executed when the function they are passed to is completed.
Promises: Promises are objects that represent the completion of an asynchronous operation. They provide a way to handle asynchronous operations in a more structured and readable way.
Async/await: Async/await is a syntax for writing asynchronous code that uses promises. It provides a more readable and concise way of writing asynchronous code.
By using these features, developers can write more efficient and responsive code that can handle complex tasks while keeping the program running smoothly.
Here are some best practices for working with asynchronous JavaScript:
Use Promises or Async/await: Promises and Async/await provide a more structured and readable way of working with asynchronous code.
Handle errors: Always handle errors properly when working with asynchronous code. This can be done using try/catch blocks or by attaching a catch handler to a Promise.
Avoid callback hell: Callback hell is a common problem when working with asynchronous code. To avoid it, use Promises or Async/await, which provide a more structured and readable way of writing asynchronous code.
Use third-party libraries: There are many third-party libraries available for working with asynchronous JavaScript, such as Axios and Async.js. These libraries can simplify complex asynchronous tasks and provide a more streamlined development process.
Optimize performance: Asynchronous code can be faster and more efficient than synchronous code, but it is important to optimize performance. Avoid unnecessary API calls and use caching when possible to reduce the amount of data that needs to be loaded.
Overall, understanding and effectively using asynchronous JavaScript is an important skill for any modern web developer. By following best practices and using the appropriate tools and libraries, developers can write efficient and responsive code that can handle complex tasks while keeping the program running smoothly.
Latest Topic
-
Cloud-Native Technologies: Best Practices
20 April, 2024 -
Generative AI with Llama 3: Shaping the Future
15 April, 2024 -
Mastering Llama 3: The Ultimate Guide
10 April, 2024
Category
- Assignment Help
- Homework Help
- Programming
- Trending Topics
- C Programming Assignment Help
- Art, Interactive, And Robotics
- Networked Operating Systems Programming
- Knowledge Representation & Reasoning Assignment Help
- Digital Systems Assignment Help
- Computer Design Assignment Help
- Artificial Life And Digital Evolution
- Coding and Fundamentals: Working With Collections
- UML Online Assignment Help
- Prolog Online Assignment Help
- Natural Language Processing Assignment Help
- Julia Assignment Help
- Golang Assignment Help
- Design Implementation Of Network Protocols
- Computer Architecture Assignment Help
- Object-Oriented Languages And Environments
- Coding Early Object and Algorithms: Java Coding Fundamentals
- Deep Learning In Healthcare Assignment Help
- Geometric Deep Learning Assignment Help
- Models Of Computation Assignment Help
- Systems Performance And Concurrent Computing
- Advanced Security Assignment Help
- Typescript Assignment Help
- Computational Media Assignment Help
- Design And Analysis Of Algorithms
- Geometric Modelling Assignment Help
- JavaScript Assignment Help
- MySQL Online Assignment Help
- Programming Practicum Assignment Help
- Public Policy, Legal, And Ethical Issues In Computing, Privacy, And Security
- Computer Vision
- Advanced Complexity Theory Assignment Help
- Big Data Mining Assignment Help
- Parallel Computing And Distributed Computing
- Law And Computer Science Assignment Help
- Engineering Distributed Objects For Cloud Computing
- Building Secure Computer Systems Assignment Help
- Ada Assignment Help
- R Programming Assignment Help
- Oracle Online Assignment Help
- Languages And Automata Assignment Help
- Haskell Assignment Help
- Economics And Computation Assignment Help
- ActionScript Assignment Help
- Audio Programming Assignment Help
- Bash Assignment Help
- Computer Graphics Assignment Help
- Groovy Assignment Help
- Kotlin Assignment Help
- Object Oriented Languages And Environments
- COBOL ASSIGNMENT HELP
- Bayesian Statistical Probabilistic Programming
- Computer Network Assignment Help
- Django Assignment Help
- Lambda Calculus Assignment Help
- Operating System Assignment Help
- Computational Learning Theory
- Delphi Assignment Help
- Concurrent Algorithms And Data Structures Assignment Help
- Machine Learning Assignment Help
- Human Computer Interface Assignment Help
- Foundations Of Data Networking Assignment Help
- Continuous Mathematics Assignment Help
- Compiler Assignment Help
- Computational Biology Assignment Help
- PostgreSQL Online Assignment Help
- Lua Assignment Help
- Human Computer Interaction Assignment Help
- Ethics And Responsible Innovation Assignment Help
- Communication And Ethical Issues In Computing
- Computer Science
- Combinatorial Optimisation Assignment Help
- Ethical Computing In Practice
- HTML Homework Assignment Help
- Linear Algebra Assignment Help
- Perl Assignment Help
- Artificial Intelligence Assignment Help
- Uncategorized
- Ethics And Professionalism Assignment Help
- Human Augmentics Assignment Help
- Linux Assignment Help
- PHP Assignment Help
- Assembly Language Assignment Help
- Dart Assignment Help
- Complete Python Bootcamp From Zero To Hero In Python Corrected Version
- Swift Assignment Help
- Computational Complexity Assignment Help
- Probability And Computing Assignment Help
- MATLAB Programming For Engineers
- Introduction To Statistical Learning
- Database Systems Implementation Assignment Help
- Computational Game Theory Assignment Help
- Database Assignment Help
- Probabilistic Model Checking Assignment Help
- Mathematics For Computer Science And Philosophy
- Introduction To Formal Proof Assignment Help
- Creative Coding Assignment Help
- Foundations Of Self-Programming Agents Assignment Help
- Machine Organization Assignment Help
- Software Design Assignment Help
- Data Communication And Networking Assignment Help
- Computational Biology
- Data Structure Assignment Help
- Foundations Of Software Engineering Assignment Help
- Mathematical Foundations Of Computing
- Principles Of Programming Languages Assignment Help
- Software Engineering Capstone Assignment Help
- Algorithms and Data Structures Assignment Help
No Comments